Removing the background from an image is a common task in image processing and can be achieved using PHP. PHP provides two powerful libraries for image manipulation: GD and ImageMagick. In this tutorial, we will explore how to remove the background from an image using both libraries.
Prerequisites
Before you begin, ensure you have the following:
- A server with PHP installed. If you need to set up PHP, follow the PHP installation guide.
- The GD library or ImageMagick installed. You can check your PHP installation for GD using
phpinfo()
. For ImageMagick, you need theimagick
PHP extension.
Method 1: Using GD Library
The GD library is a powerful tool for creating and manipulating images in PHP. For more details, refer to the GD documentation.
Step 1: Load the Image
First, load the image into a GD resource:
$imagePath = 'path/to/your/image.png';
$image = imagecreatefrompng($imagePath);
if (!$image) {
die('Failed to load image');
}
Step 2: Detect the Background Color
For simplicity, let's assume the background color is the top-left pixel of the image:
$bgColor = imagecolorat($image, 0, 0);
Step 3: Create a New Image with Transparency
Create a new image with the same dimensions and enable transparency:
$width = imagesx($image);
$height = imagesy($image);
$newImage = imagecreatetruecolor($width, $height);
imagesavealpha($newImage, true);
$transparency = imagecolorallocatealpha($newImage, 0, 0, 0, 127);
imagefill($newImage, 0, 0, $transparency);
Step 4: Copy the Non-Background Pixels
Loop through each pixel and copy only the non-background pixels to the new image:
for ($x = 0; $x < $width; $x++) {
for ($y = 0; $y < $height; $y++) {
if (imagecolorat($image, $x, $y) !== $bgColor) {
imagesetpixel($newImage, $x, $y, imagecolorat($image, $x, $y));
}
}
}
Step 5: Save the New Image
Finally, save the new image:
imagepng($newImage, 'path/to/your/new_image.png');
imagedestroy($image);
imagedestroy($newImage);
Method 2: Using ImageMagick
ImageMagick is a powerful image processing library. The PHP extension for ImageMagick is imagick
. For more details, refer to the ImageMagick documentation.
Step 1: Load the Image
Load the image using ImageMagick:
$imagePath = 'path/to/your/image.png';
$image = new Imagick($imagePath);
if (!$image) {
die('Failed to load image');
}
Step 2: Set Image to Transparent
Identify and set the background color to transparent. Assume the background color is the top-left pixel:
$backgroundColor = $image->getImagePixelColor(0, 0);
$image->transparentPaintImage($backgroundColor, 0, 0, false);
Step 3: Save the New Image
Save the new image:
$image->writeImage('path/to/your/new_image.png');
$image->destroy();
Conclusion
Removing the background from an image in PHP can be achieved using the GD library or ImageMagick. While GD provides basic image manipulation capabilities, ImageMagick offers more advanced features and better performance. Choose the library that best fits your needs and environment.
For more information, refer to the GD documentation and the ImageMagick documentation. By following these steps, you can efficiently remove backgrounds from images using PHP.
These methods of background removal only work if you have a solid background color on an image. If you have a more complex image then you will need to implement machine learning and AI techniques to help remove the image background, which is beyond the scope of this article.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
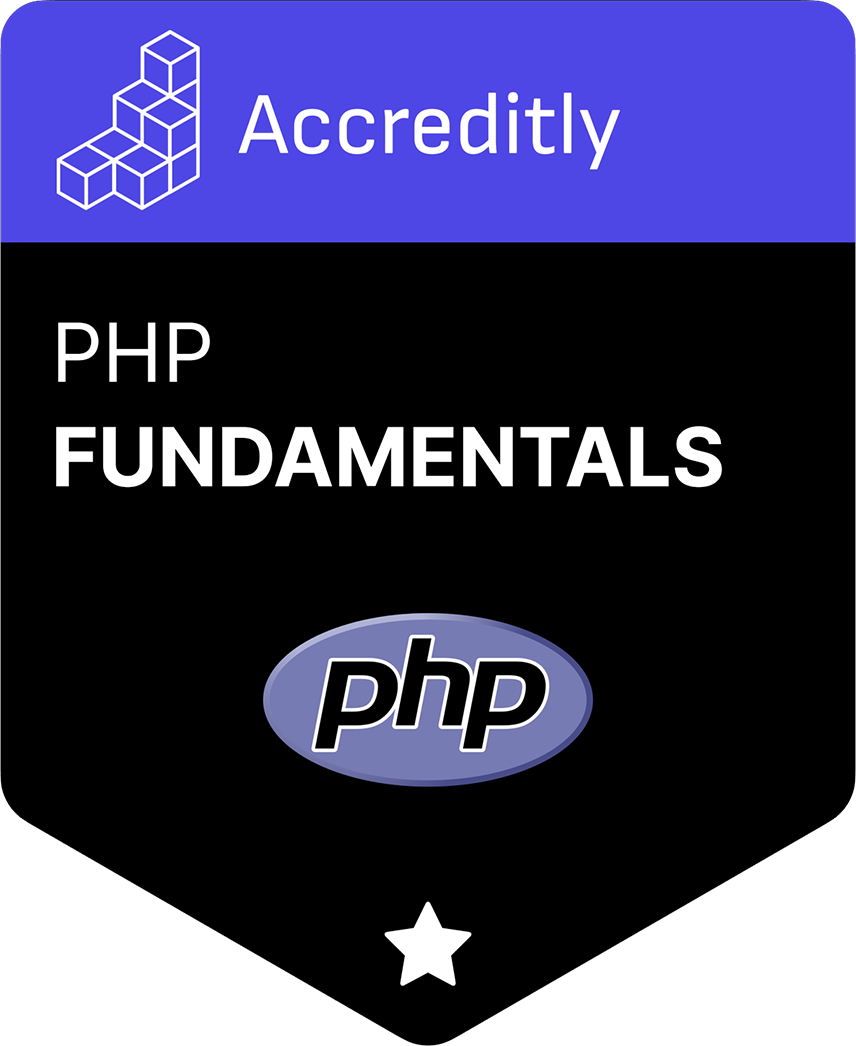