- Understanding the Navigator.onLine Property
- Listening to Online and Offline Events
- Creating a Connection Status Indicator
- Handling False Positives
- Detecting connectivity
In today’s interconnected world, web applications are expected to be smart and adaptive, adjusting their behavior based on the availability of an internet connection. Detecting internet connectivity can significantly improve user experience, allowing applications to warn users about connectivity issues, save changes locally until a connection is re-established, or adjust the content and functionality available based on the connection status. JavaScript provides straightforward methods to monitor internet connectivity. This tutorial explores how to implement these methods effectively.
Understanding the Navigator.onLine Property
JavaScript offers a simple way to check the online status of the browser through the Navigator.onLine
property. It returns a boolean value: true
if the browser has internet access and false
otherwise. It's worth noting, however, that Navigator.onLine
checks if the system is connected to any network, not necessarily the internet. Thus, a true
value doesn't guarantee internet access; it merely indicates network connectivity.
Basic Usage:
if (navigator.onLine) {
console.log("The browser is online.");
} else {
console.log("The browser is offline.");
}
Listening to Online and Offline Events
To create a dynamic application that responds to changes in internet connectivity, you can listen to the online
and offline
events on the window object. These events are triggered whenever the browser's network status changes.
Example Implementation:
window.addEventListener('online', function(e) {
console.log("You are online!");
// Implement any logic that should occur when the connection is restored.
});
window.addEventListener('offline', function(e) {
console.log("You are offline!");
// Implement any logic that should occur when the connection is lost.
});
This method allows your application to react in real-time to connectivity changes, improving the user experience in scenarios where internet access is intermittent or unreliable.
Creating a Connection Status Indicator
Combining Navigator.onLine
with the online
and offline
events, you can develop a feature in your web application that continuously displays the current connection status to the user.
HTML:
<div id="connectionStatus" class="online">You are online</div>
CSS:
#connectionStatus.online {
background-color: green;
color: white;
}
#connectionStatus.offline {
background-color: red;
color: white;
}
JavaScript:
function updateConnectionStatus() {
const statusDisplay = document.getElementById('connectionStatus');
if (navigator.onLine) {
statusDisplay.textContent = 'You are online';
statusDisplay.classList.remove('offline');
statusDisplay.classList.add('online');
} else {
statusDisplay.textContent = 'You are offline';
statusDisplay.classList.remove('online');
statusDisplay.classList.add('offline');
}
}
// Monitor connection changes.
window.addEventListener('online', updateConnectionStatus);
window.addEventListener('offline', updateConnectionStatus);
// Check the connection status when the script loads.
updateConnectionStatus();
Handling False Positives
Since Navigator.onLine
can sometimes give a false positive (indicating online when there's no internet access), you might need to perform an additional check for actual internet connectivity. A common approach is to send a fetch request to a reliable endpoint, like a server you control or a public CDN, and see if it succeeds.
Example:
function checkRealInternetConnection() {
fetch('https://www.gstatic.com/generate_204', {
method: 'HEAD',
cache: 'no-cache'
})
.then(() => console.log("Real internet access confirmed."))
.catch(() => console.log("No real internet access."));
}
// You may call this function after detecting an 'online' status.
Detecting connectivity
Detecting internet connectivity in JavaScript enhances the adaptability of web applications, enabling them to provide feedback and adjust functionality based on the user’s connection status. By responding to online and offline events, applications can offer a more resilient and user-friendly experience, particularly in environments with unstable internet access. Incorporating these checks and balances ensures that your application remains functional and informative, even in the face of connectivity challenges.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
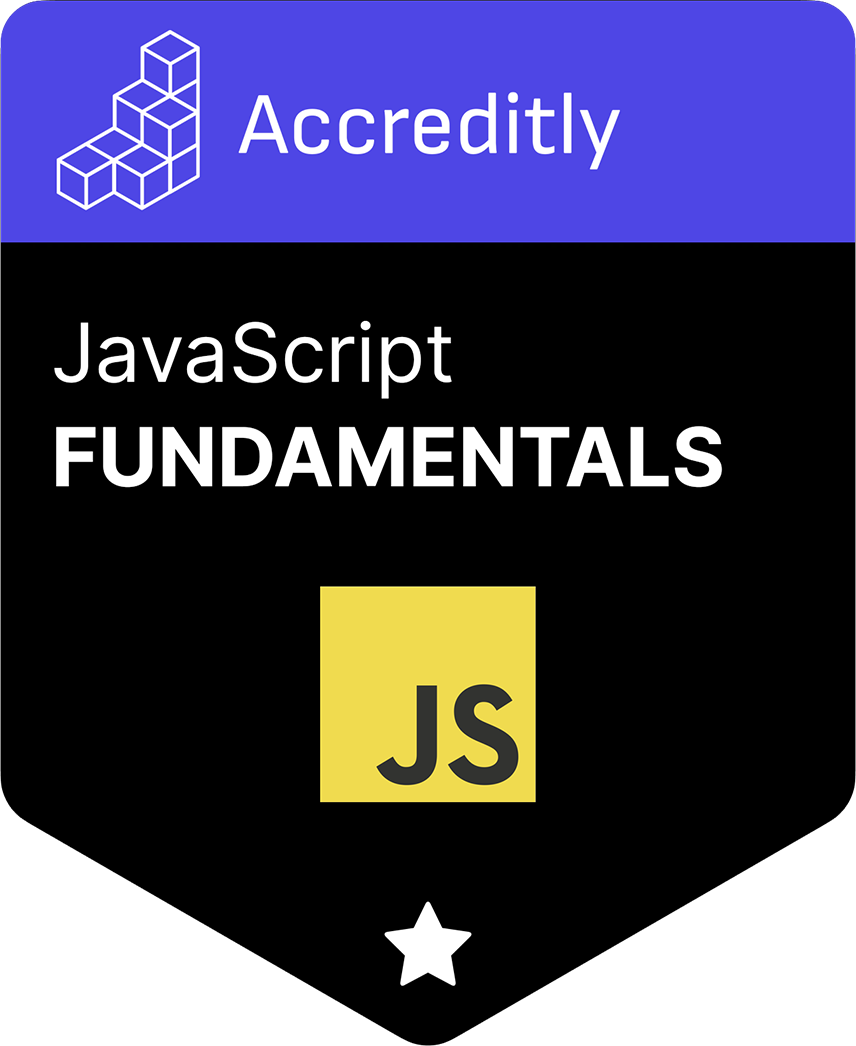