- What is Paddle?
- Setting Up Paddle
- Integrating Paddle with PHP
- Processing Payments
- Handling Webhooks
- Testing Your Integration
- Wrapping Up: Implementing Paddle in PHP
Implementing a payment gateway into your web application can be a daunting task. Paddle, a popular payment solution for SaaS and digital products, simplifies this process by offering robust APIs and SDKs. This article will guide you through integrating Paddle into a PHP project, covering setup, payment processing, and handling webhooks.
What is Paddle?
Paddle is a comprehensive payment processing platform designed for SaaS and digital product businesses. It offers features like recurring billing, global payment methods, tax handling, and fraud prevention. With its developer-friendly APIs and extensive documentation, integrating Paddle into your PHP project can be streamlined and efficient.
Setting Up Paddle
Before diving into the code, you need to set up your Paddle account and configure the necessary settings.
-
Create a Paddle Account: Sign up for a Paddle account at Paddle's website.
-
Configure Your Paddle Settings: Navigate to your Paddle dashboard and set up your product catalog, pricing, and payment options. Make sure to obtain your Vendor ID and API key from the Developer Tools section.
-
Install the Paddle PHP SDK: Paddle does not have an official PHP SDK, but you can use third-party libraries like
paddle-sdk-php
. Install it using Composer:composer require ampeco/paddle-sdk-php
Integrating Paddle with PHP
To start integrating Paddle into your PHP project, you need to initialize the Paddle client and configure your environment.
-
Initialize the Paddle Client:
Create a file named
config.php
to store your Paddle credentials:<?php require 'vendor/autoload.php'; use Amp\paddle_sdk\Paddle; $vendorId = 'your-vendor-id'; $apiKey = 'your-api-key'; $paddle = new Paddle($vendorId, $apiKey); ?>
-
Create a Checkout Button:
Paddle provides a JavaScript library for creating a checkout experience. Include Paddle's script in your HTML:
<script src="https://cdn.paddle.com/paddle/paddle.js"></script> <script type="text/javascript"> Paddle.Setup({ vendor: your-vendor-id }); </script>
Processing Payments
Once the Paddle client is initialized, you can create checkout links or display a checkout overlay for processing payments.
-
Generate a Checkout Link:
Use the Paddle API to generate a checkout link:
<?php require 'config.php'; $response = $paddle->generatePayLink([ 'product_id' => 12345, 'title' => 'Your Product Title', 'price' => 19.99, 'customer_email' => '[email protected]' ]); echo $response['url']; ?>
This code generates a payment link for a specific product. The URL can be used to redirect customers to the Paddle checkout page.
-
Display a Checkout Overlay:
You can also display a checkout overlay directly on your website using Paddle's JavaScript library:
<button id="checkout-btn">Buy Now</button> <script type="text/javascript"> document.getElementById('checkout-btn').addEventListener('click', function() { Paddle.Checkout.open({ product: 12345, title: 'Your Product Title', price: 19.99 }); }); </script>
Handling Webhooks
Webhooks are crucial for handling post-payment events like order completion, refunds, and subscription changes. Paddle sends webhook notifications to your server when these events occur.
-
Set Up Your Webhook Endpoint:
Create a PHP file named
webhook.php
to handle webhook events:<?php require 'config.php'; $webhookData = json_decode(file_get_contents('php://input'), true); if (isset($webhookData['alert_name'])) { switch ($webhookData['alert_name']) { case 'payment_succeeded': // Handle successful payment $orderId = $webhookData['order_id']; // Update your database, send emails, etc. break; case 'subscription_created': // Handle subscription creation $subscriptionId = $webhookData['subscription_id']; // Update your database, send emails, etc. break; // Handle other webhook events // case 'alert_name': // // code to handle event // break; } } http_response_code(200); // Acknowledge receipt of the webhook ?>
-
Configure Webhook URL in Paddle Dashboard:
Go to your Paddle dashboard and set the webhook URL to the endpoint you just created, e.g.,
https://yourdomain.com/webhook.php
.
Testing Your Integration
Before going live, thoroughly test your integration to ensure everything works as expected.
-
Use Paddle's Sandbox Environment:
Paddle provides a sandbox environment for testing. Enable the sandbox mode in your Paddle settings and use the sandbox credentials for testing.
-
Simulate Webhook Events:
Use Paddle’s dashboard to manually trigger webhook events to ensure your webhook handling code works correctly.
Wrapping Up: Implementing Paddle in PHP
Integrating Paddle into your PHP project involves setting up your Paddle account, initializing the Paddle client, processing payments, and handling webhooks. By following this guide, you can seamlessly incorporate Paddle's payment processing capabilities into your application, ensuring a smooth and secure checkout experience for your customers.
For further details, refer to the official Paddle documentation and the Paddle SDK for PHP.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
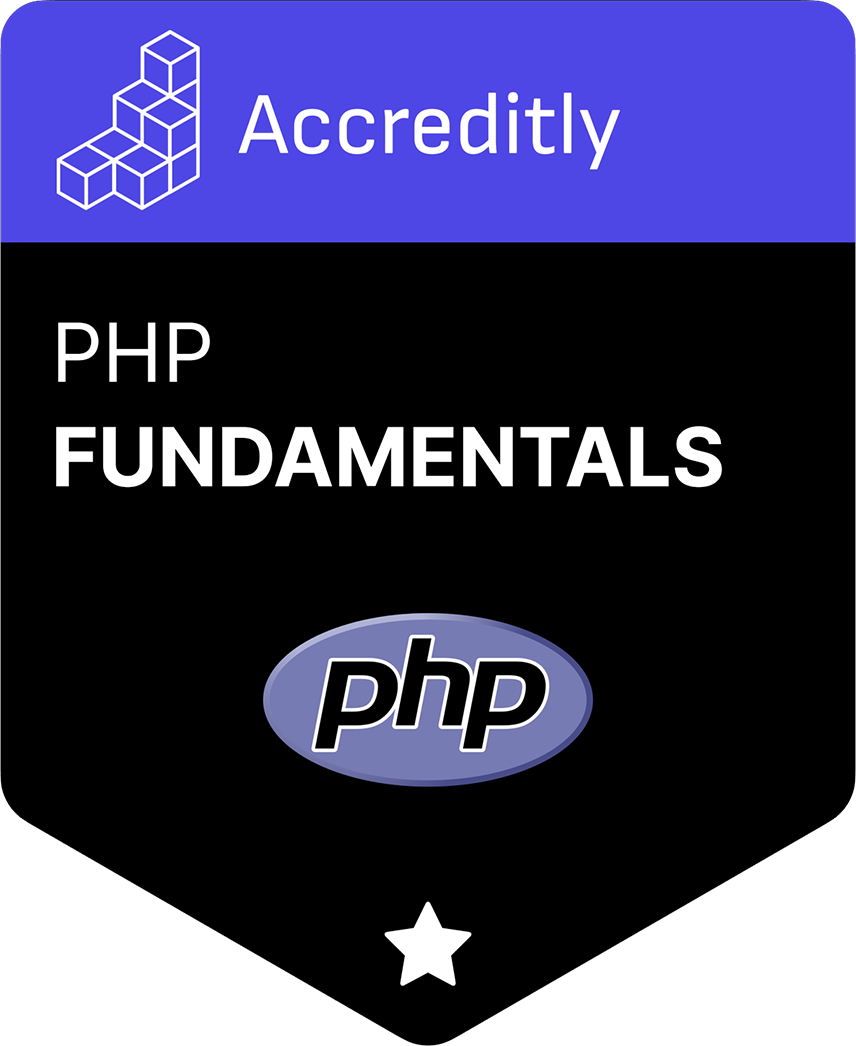