- Understanding the Problem
- Using Laravel's withoutOverlapping Method
- Configuring a Timeout
- Ensuring Proper Cache Configuration
- Alternative Methods
- Best Practices
Laravel's task scheduler is a powerful feature that allows developers to schedule various tasks with ease. However, some tasks, especially long-running ones, risk overlapping if not properly managed. An overlapping task could lead to data inconsistencies, overuse of resources, and other unexpected issues.
This article delves into methods to prevent your Laravel scheduled commands from running simultaneously.
Understanding the Problem
Imagine a scenario where you have a scheduled command that runs every minute to sync data. If the process of syncing takes longer than a minute, a new instance of the command could start running while the previous one is still active. This overlap can introduce complications, especially if they're accessing shared resources or databases.
withoutOverlapping
Method
Using Laravel's Laravel provides a built-in method called withoutOverlapping
that prevents the scheduled task from running if the previous instance of the task is still running.
To use it, simply chain the withoutOverlapping
method to your scheduled task:
$schedule->command('sync:data')->everyMinute()->withoutOverlapping();
When this is set, Laravel will check for the existence of a specific cache file before running the task. If the file exists (indicating the task is still running), the new task won't be initiated.
Configuring a Timeout
By default, the withoutOverlapping
method prevents tasks from overlapping for 24 hours. If you expect a task might hang or wish to allow another attempt sooner, you can define a timeout (in minutes) as an argument:
$schedule->command('sync:data')->everyMinute()->withoutOverlapping(10);
In this case, if the sync:data
command runs longer than 10 minutes, the next scheduled task after the 10-minute mark can start.
Ensuring Proper Cache Configuration
The withoutOverlapping
method relies on Laravel's caching mechanism to function. Ensure you've configured your cache correctly, and it's operational. Using the file
or redis
cache driver is generally recommended for scheduled tasks.
Alternative Methods
-
External mutex systems, like Redis or database locks, can be used to ensure a task doesn't overlap. When a task starts, it attempts to obtain a lock. If it can't (because another instance has the lock), it won't run.
-
Tools like Laravel Horizon (for queued jobs) or third-party services like Laravel Forge provide insights into running tasks. They can alert you if a task is taking longer than expected, helping you adjust schedules or optimize command logic. This method does rely on some manual intervention, but depending on your use-case this may be acceptable, especially in very complex and very long-running tasks.
Best Practices
-
Before setting up overlap prevention, consider optimizing your commands to ensure they run efficiently and within the expected time frames. It's better for your tasks to not need to overlap in the first place.
-
Implement logging in your scheduled tasks. This way, if tasks are frequently taking longer than expected, you'll have records to analyze and base optimizations on.
-
If possible, stagger your tasks to run at different intervals or times, reducing the chances of overlap, especially for tasks accessing shared resources. In Laravel you can run the same task at multiple time schedules by adding multiple lines to the scheduler.
Laravel's task scheduler is immensely powerful, but with great power comes the need for responsibility. By understanding the potential issues of overlapping tasks and using tools like withoutOverlapping
, you can ensure your scheduled tasks run smoothly and your application remains robust and efficient.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
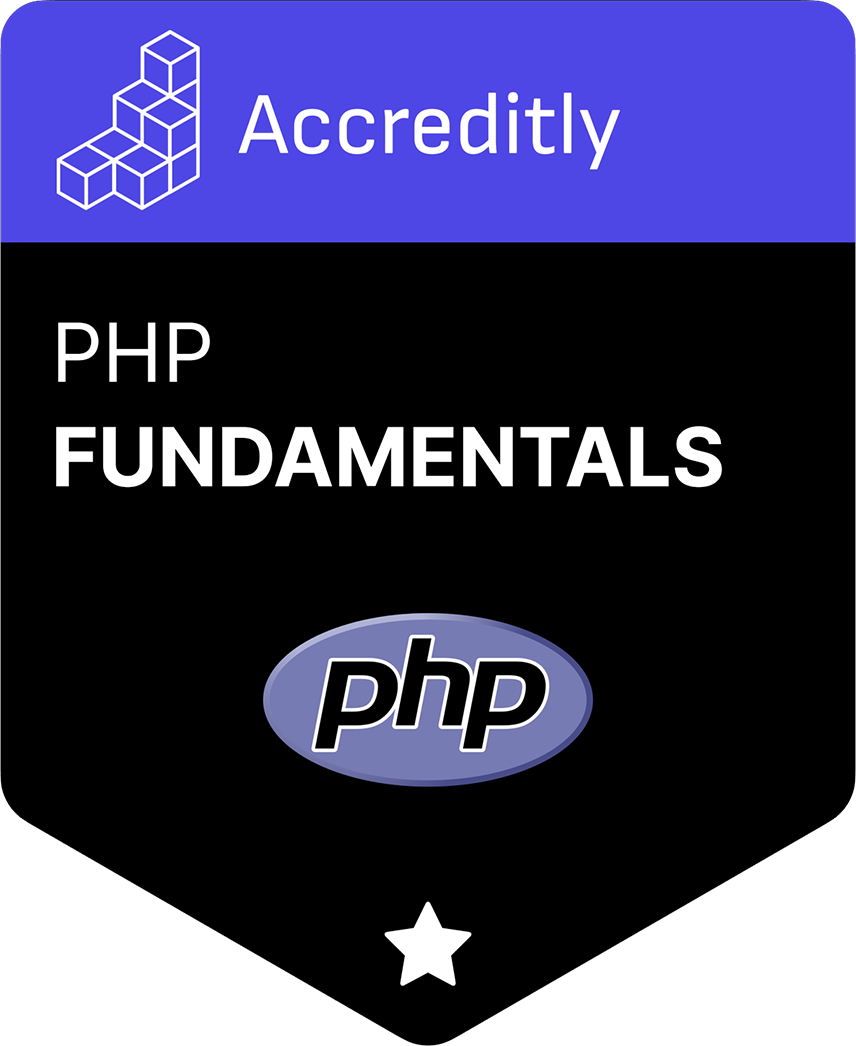