Errors are an integral part of every developer's journey. They're not only inevitable but also valuable tools for understanding and improving our code. JavaScript, being a dynamic and loosely-typed language, throws its fair share of errors, each offering insights into specific problem areas. In this article, we'll focus on three common errors: ReferenceError, SyntaxError, and TypeError.
1. ReferenceError
A ReferenceError
is thrown when you attempt to use a variable (or a reference) that hasn't been declared.
Common Occurrences:
- Accidentally referencing a variable before declaring it.
- Trying to access a variable outside of its scope.
Example:
console.log(undeclaredVariable);
Fix: Ensure the variable has been declared and is within the scope where you're trying to use it.
2. SyntaxError
A SyntaxError
is thrown when you've written code that doesn't conform to the structure or syntax rules of the JavaScript language.
Common Occurrences:
- Missed or extra punctuation, like braces or semicolons.
- Incorrect usage of language constructs.
Example:
if(true)
{
console.log("Missing a closing parenthesis.");
Fix: Review your code to ensure it follows JavaScript's syntax rules. Integrated development environments (IDEs) and linters can help highlight these issues automatically.
3. TypeError
A TypeError
is thrown when an operation is performed on a value of an unexpected type.
Common Occurrences:
- Trying to call something that isn't a function.
- Accessing properties or methods on
null
orundefined
. - Using an object as a function, or vice-versa.
Example:
let greeting = "Hello, world!";
greeting();
Fix: Ensure you're performing operations on values of the correct type. For instance, check if a variable is callable (a function) before invoking it.
Troubleshooting Tips
- Console Logging: If in doubt,
console.log()
the variable or reference causing the error. This can help you understand its current state and type. - Use Linters: Tools like ESLint can help detect potential errors before runtime. They also guide you on best practices.
- Read the Error Message: Often, the error message will tell you the line number and give a brief description of what went wrong.
- Use Debugging Tools: Modern browsers have advanced debugging tools that let you pause execution (breakpoints), step through code, and inspect variables.
Understanding errors is crucial to debugging efficiently. By differentiating between ReferenceError
, SyntaxError
, and TypeError
, you can pinpoint the nature of your mistake more quickly, leading to faster and more effective troubleshooting. So, the next time you encounter these errors, you'll know just where to start your investigation!
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
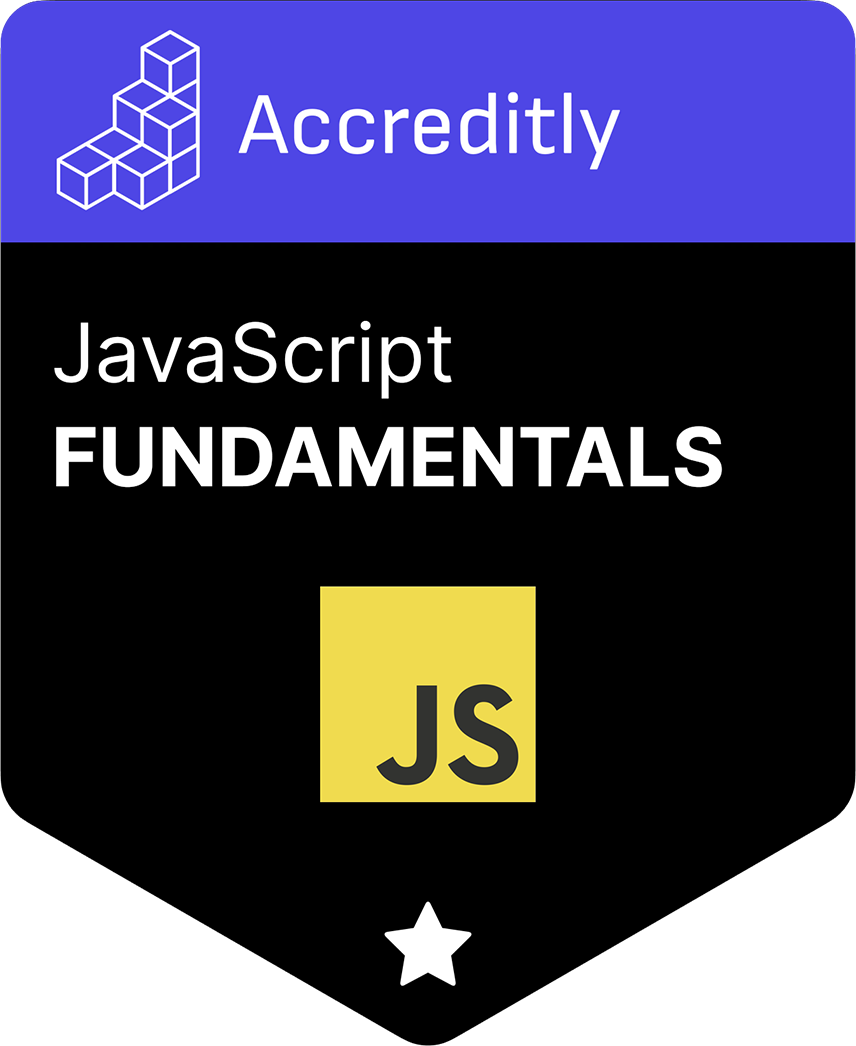