- What is nextSibling?
- Working with nextSibling
- Dealing with Whitespace and Comments
- Using nextElementSibling for Elements Only
- Practical Usage of nextSibling
JavaScript offers a plethora of methods and properties to navigate and manipulate the Document Object Model (DOM). One such property is nextSibling
. This article will provide a comprehensive understanding of the nextSibling
property in JavaScript, demonstrating its use with practical code examples.
Read about nextSibling
in the MDN docs.
What is nextSibling?
The nextSibling
property is part of the Node interface in the DOM API. It allows you to access the next sibling node of the selected element in the node tree. A sibling node is a node that shares the same parent node.
It's important to understand that nextSibling considers all types of nodes, including element nodes, text nodes, and comment nodes. This might lead to unexpected results if your HTML structure includes whitespace or comments.
nextSibling
Working with Let's start with a basic HTML structure:
<ul id="list">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
If we want to select the next sibling of the first li element, we can do so with nextSibling
:
let firstItem = document.querySelector('#list li');
let nextItem = firstItem.nextSibling;
console.log(nextItem.textContent); // outputs: "Item 2"
This code selects the first li
element in the list and then selects its next sibling.
Dealing with Whitespace and Comments
As mentioned earlier, nextSibling
considers all types of nodes. This can become an issue with the way HTML is usually formatted. For example, consider the following HTML:
<ul id="list">
<li>Item 1</li>
<!-- This is a comment -->
<li>Item 2</li>
<li>Item 3</li>
</ul>
If you use the same JavaScript code as above, the output will be different:
let firstItem = document.querySelector('#list li');
let nextItem = firstItem.nextSibling;
console.log(nextItem.textContent); // outputs: " This is a comment "
Here, nextSibling
selects the comment node, not the next li
element as you might expect. So what do we do?
nextElementSibling
for Elements Only
Using To ensure that you're only dealing with element nodes, you can use the nextElementSibling
property instead. This property only considers element nodes, which are typically what you want when manipulating the DOM.
Let's adjust the previous JavaScript code to use nextElementSibling
:
let firstItem = document.querySelector('#list li');
let nextItem = firstItem.nextElementSibling;
console.log(nextItem.textContent); // outputs: "Item 2"
Even though there's a comment node between the li
elements, nextElementSibling
correctly selects the next li
element.
Practical Usage of nextSibling
While nextSibling
or nextElementSibling
are helpful for traversing the DOM, they can also be useful for manipulating elements based on their position.
For example, suppose you have a list where clicking an item highlights the next item. You could use nextElementSibling
to easily select the next item:
let listItems = document.querySelectorAll('#list li');
listItems.forEach((item) => {
item.addEventListener('click', (e) => {
let nextItem = e.target.nextElementSibling;
if (nextItem) {
nextItem.style.backgroundColor = 'yellow';
}
});
});
In this example, clicking an li
item changes the background color of the next item in the list.
Understanding and using the nextSibling
property in JavaScript is key to traversing and manipulating the DOM effectively. While you may more often need nextElementSibling
to deal with element nodes, being aware of nextSibling
and its behaviours will help you handle more complex scenarios and improve your JavaScript coding skills.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
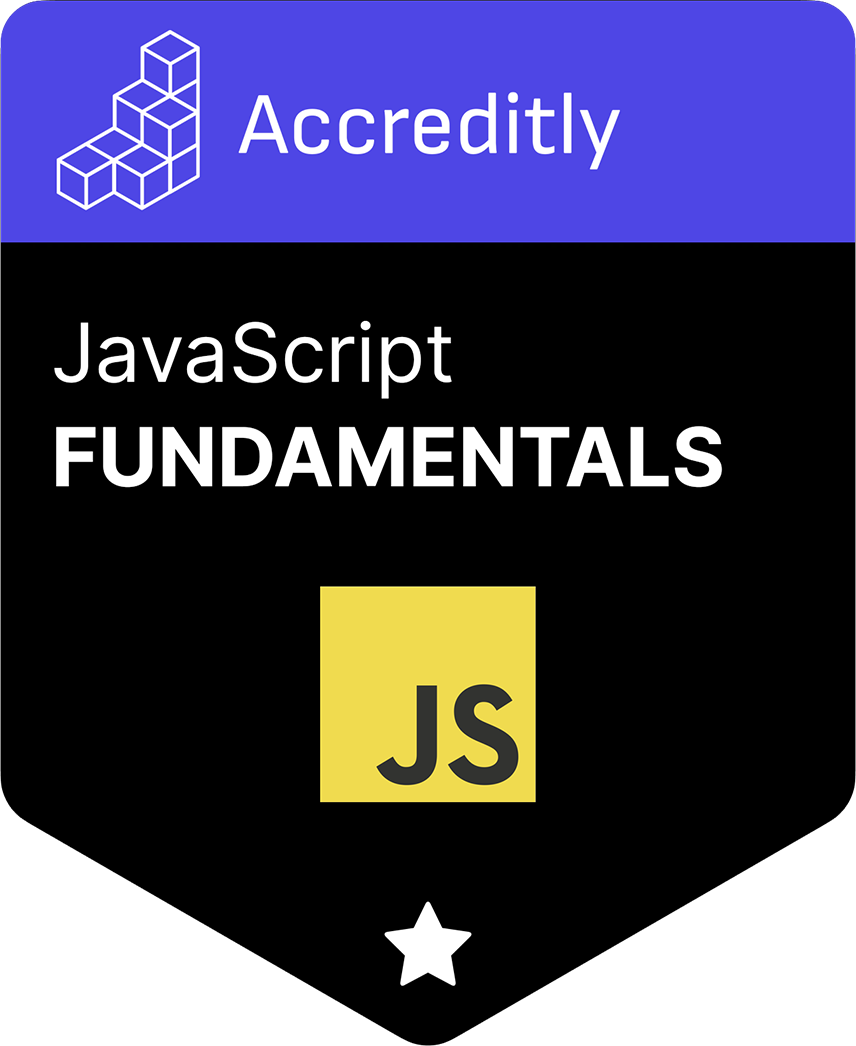