- Prerequisites
- Step 1: Understanding FFmpeg
- Step 2: Setting Up the Environment
- Step 3: Creating a Video From Images
- Step 4: Error Handling and Considerations
Video generation and manipulation is an essential part of many modern web applications. From social media apps that allow users to upload and edit videos, to business applications that generate video reports, the ability to create videos programmatically can enhance your app's capabilities.
In this tutorial, we will discuss how to use JavaScript for this purpose, specifically focusing on a library called FFmpeg.js, which is a JavaScript port of the popular media library FFmpeg.
Prerequisites
- Basic knowledge of JavaScript
- Familiarity with Node.js and npm (Node package manager)
- Basic understanding of video file formats and codecs
If you're interested in learning about video file formats and codecs then we recommend reading this article.
Step 1: Understanding FFmpeg
FFmpeg is a free and open-source software project that produces libraries and programs for handling multimedia data. It provides command-line tools to convert multimedia files between different formats. FFmpeg.js is a JavaScript wrapper around FFmpeg, and it brings the power of FFmpeg to JavaScript.
Step 2: Setting Up the Environment
Before we start using FFmpeg.js, we need to install it using npm (yarn is cool too). Run the following command in your project directory:
npm install ffmpeg.js
Step 3: Creating a Video From Images
Let's consider a simple example where we want to create a video from a sequence of images. Here's how you can do it using FFmpeg.js:
const ffmpeg = require('ffmpeg.js');
const fs = require('fs');
// Load images from disk
const image1 = fs.readFileSync('image1.jpg');
const image2 = fs.readFileSync('image2.jpg');
// Create video using FFmpeg
ffmpeg({
MEMFS: [
{ name: 'image1.jpg', data: image1 },
{ name: 'image2.jpg', data: image2 }
],
arguments: ['-framerate', '1', '-i', 'image%d.jpg', 'output.mp4']
}, function (err, result) {
if (err) {
console.error(err);
return;
}
// Save video to disk
const video = result.MEMFS[0];
fs.writeFileSync(video.name, video.data);
});
In the above code, we first load the images from disk using the fs.readFileSync
method. We then create a video from these images using the ffmpeg
function. The -framerate
option specifies the number of frames (images) per second, and the -i
option specifies the input files. The output.mp4
is the output file.
Step 4: Error Handling and Considerations
Always remember to add error handling in your code. The FFmpeg process can fail for various reasons like invalid input, missing codecs, or insufficient disk space. It's essential to handle these errors appropriately in your application.
Also, keep in mind that video processing is a CPU-intensive task. Performing video processing tasks on the client side could lead to high CPU usage and potentially degrade the performance of your application. Depending on your use case, you might want to consider offloading these tasks to the server or using Web Workers to run them in a separate thread.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
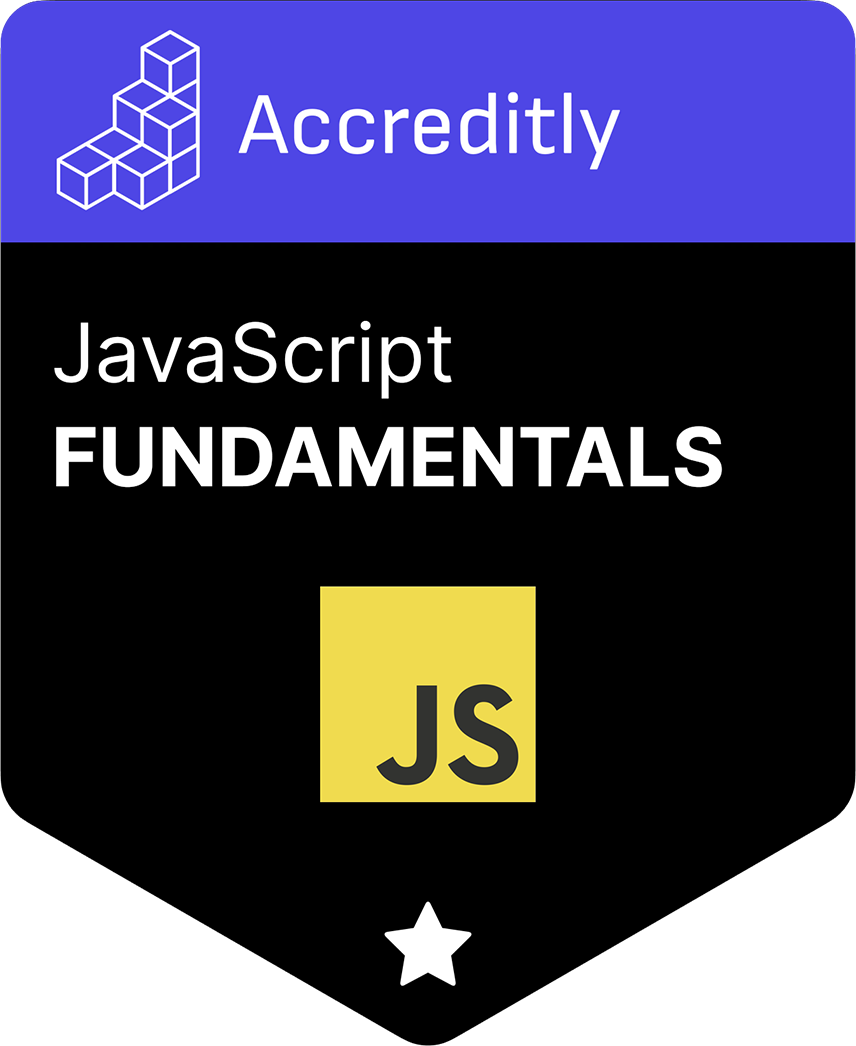