- 1. Basic Syntax
- 2. Defining Custom Attributes
- 3. Retrieving Attributes
- 4. Use Cases
- 5. Important Considerations
PHP 8 introduced a host of new features, but one of the most intriguing is the addition of attributes (also known as annotations in other languages). They allow developers to add metadata to classes, functions, properties, and even function/method parameters without affecting their behavior. This metadata can then be retrieved and acted upon at runtime.
1. Basic Syntax
Attributes in PHP are prefixed with a #
symbol and wrapped in square brackets. They can be applied to classes, functions, and properties.
#[MyAttribute]
class SampleClass {
#[AnotherAttribute]
public $property;
#[YetAnotherAttribute]
public function method() {}
}
2. Defining Custom Attributes
To define a custom attribute, you need to create a class that's marked with the #[Attribute]
attribute.
#[Attribute]
class MyAttribute {
public function __construct(public string $value = '') {}
}
3. Retrieving Attributes
You can retrieve attributes using PHP's reflection classes, like ReflectionClass
, ReflectionMethod
, and ReflectionProperty
.
$reflector = new ReflectionClass(SampleClass::class);
$attributes = $reflector->getAttributes();
foreach ($attributes as $attribute) {
echo 'Attribute name: ' . $attribute->getName() . PHP_EOL;
// You can also instantiate the attribute to get any arguments passed to it.
$instance = $attribute->newInstance();
echo 'Attribute value: ' . $instance->value . PHP_EOL;
}
4. Use Cases
Attributes can be used in a multitude of ways, such as:
-
ORM Mapping: Instead of configuring how a class maps to a database table in separate configuration files, you can use attributes directly on the class and its properties.
-
Validation: Attach validation rules to properties, making the rules and the properties they apply to more closely related.
-
Middleware: Specify middleware on controller methods to indicate what should run before or after the method.
5. Important Considerations
-
Runtime Overhead: Fetching attributes using reflection might introduce some runtime overhead. Be sure to test and profile your application to ensure performance remains acceptable.
-
Documentation and Readability: While attributes can help declutter code by removing configuration or other metadata-related tasks, be aware that overusing attributes might make the code harder to read.
Attributes in PHP provide a robust method for adding metadata to your codebase, enabling more expressive and concise code. By understanding how to define, retrieve, and utilize them, developers can leverage attributes for a range of use cases, from ORM configuration to input validation. As with any feature, though, it's crucial to strike a balance to maintain clarity and performance in your application.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
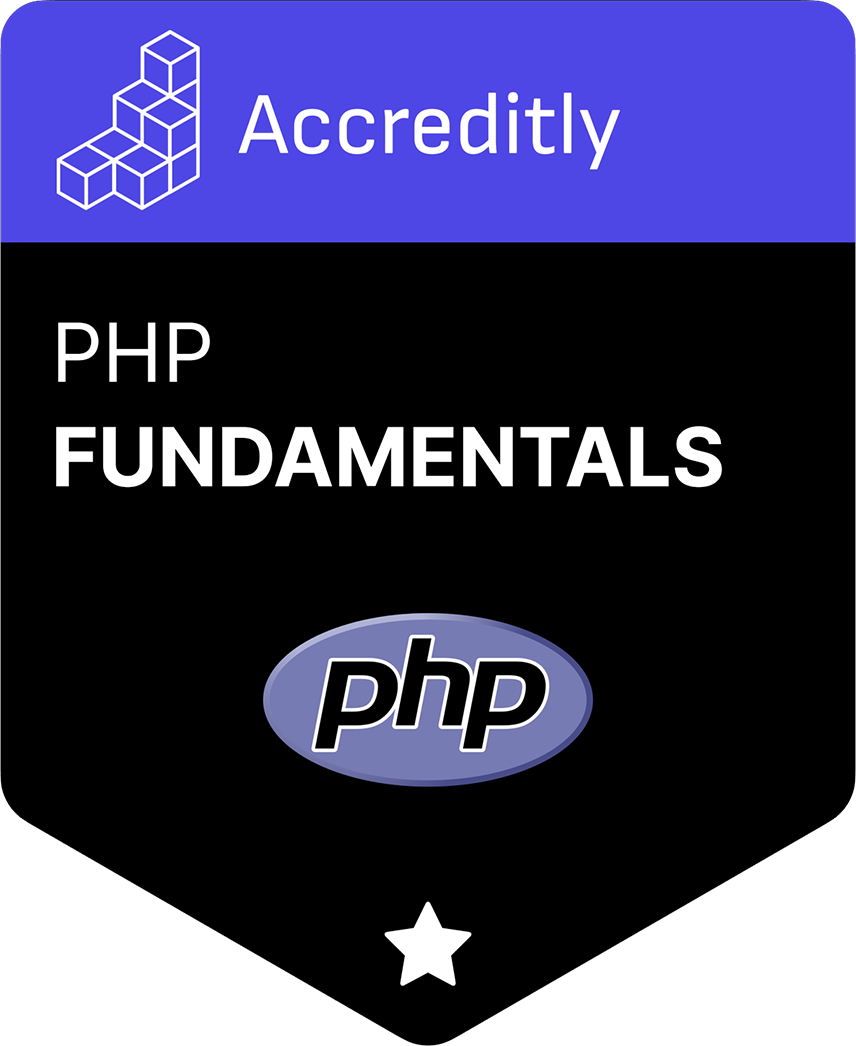