- Understanding Livewire Nesting
- Every Component is an Island
- Nesting Components: An Example
- Gotchas with Livewire Nesting
- Keeping Track of Components in a Loop
- Conclusion
In modern web development, component-based architectures have become a staple for building scalable and maintainable applications. Laravel Livewire, being a full-stack framework for Laravel, adheres to this paradigm and introduces a straightforward way to nest components within one another. This article delves into the concept of component nesting in Livewire 3, illustrated with code block examples to provide a clear understanding of this feature.
Understanding Livewire Nesting
Like many other component-based frameworks, Livewire allows for component nesting, which means a component can render other components within itself. However, the nesting system in Livewire is built differently than in other frameworks, which brings about certain implications and constraints.
You may find it useful to skim the docs before reading on.
Before diving into nesting, it's crucial to understand Livewire's hydration system, as it plays a significant role in how nested components behave and interact with each other.
Every Component is an Island
In Livewire, each component on a page tracks its state and makes updates independently of other components. For instance, consider the following Posts
and nested ShowPost
components:
namespace App\Livewire;
use Illuminate\Support\Facades\Auth;
use Livewire\Component;
class Posts extends Component {
public $postLimit = 2;
public function render() {
return view('livewire.posts', [
'posts' => Auth::user()->posts()
->limit($this->postLimit)->get(),
]);
}
}
// In the Blade view
<div>
Post Limit: <input type="number" wire:model.live="postLimit">
@foreach ($posts as $post)
<livewire:show-post :post="$post" :key="$post->id">
@endforeach
</div>
In the example above, the Posts
component renders multiple ShowPost
components, each tied to a specific post.
Nesting Components: An Example
Below is another example showcasing a Dashboard
parent component that contains a nested TodoList
component:
namespace App\Livewire;
use Livewire\Component;
class Dashboard extends Component {
public function render() {
return view('livewire.dashboard');
}
}
// In the Blade view
<div>
<h1>Dashboard</h1>
<livewire:todo-list />
</div>
In this snippet, the Dashboard
component nests the TodoList
component within its view. Read more in the docs.
Gotchas with Livewire Nesting
Livewire supports nesting, yet there are certain "gotchas" to be aware of:
- Data Passing: Nested components can accept data parameters from their parents, but they are not reactive like props in Vue.js.
- Blade Snippets: Livewire components should not be used for extracting Blade snippets into separate files; instead, Blade includes or components are preferable.
Keeping Track of Components in a Loop
When rendering components inside a loop, Livewire offers a special "key" syntax to keep track of each component, similar to Vue.js. Here's an example:
<div>
@foreach ($users as $user)
@livewire('user-profile', ['user' => $user], key($user->id))
@endforeach
</div>
In Laravel 7 or above, you can use the tag syntax as follows:
<div>
@foreach ($users as $user)
<livewire:user-profile :user="$user" :wire:key="$user->id">
@endforeach
</div>
This way, each component retains its identity across renders, which is crucial for consistent behavior.
Conclusion
Nesting components in Laravel Livewire 3 provides a modular approach to building your applications. By understanding the principles and best practices of nesting, you can create more structured, maintainable, and scalable Laravel applications.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
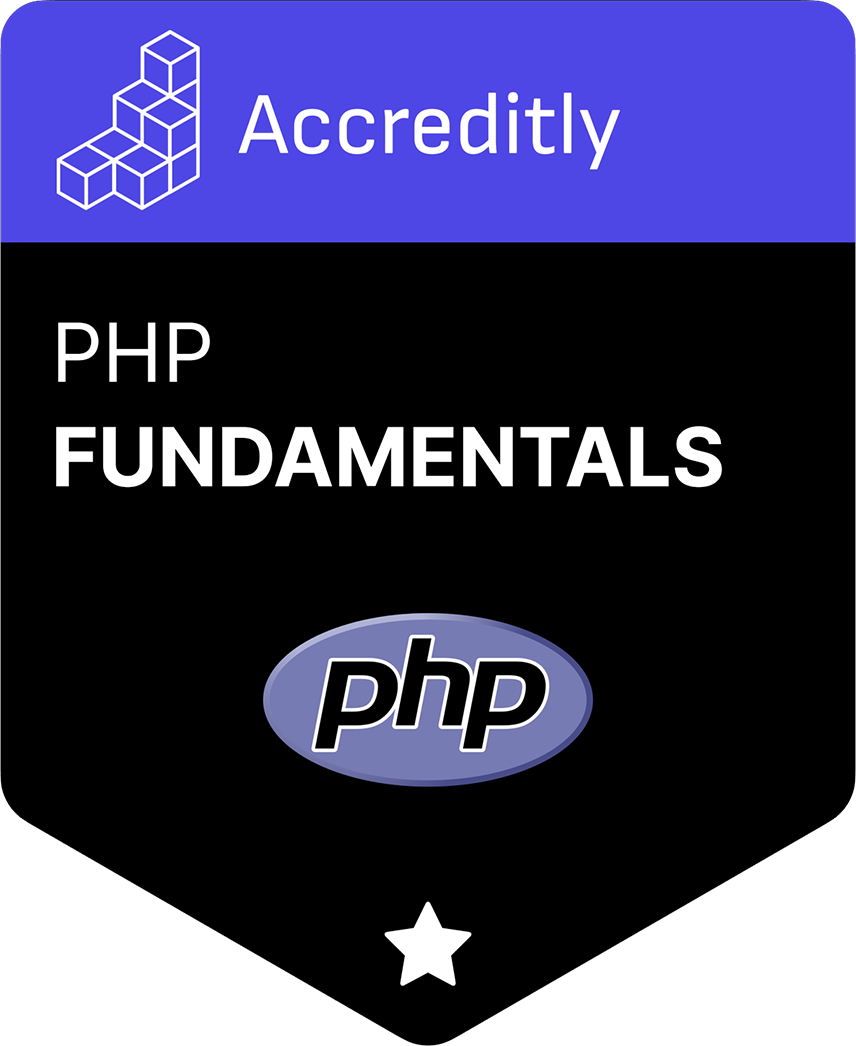
Related articles
Tutorials WordPress PHP Tooling
Optimizing WordPress YouTube Embeds with Lite YouTube Embed
Learn how to enhance your WordPress site's performance by replacing the native YouTube embed with Lite YouTube Embed. This guide takes you through the steps to override the default oEmbed behaviour for YouTube videos.