- What Are Namespaces?
- Using Namespaces
- Splitting Across Multiple Files
- Namespaces vs Modules
- Conclusion
When working with larger TypeScript projects, the organization of code becomes crucial. Namespaces, a TypeScript-specific feature, allow developers to structure their code in a clean, modular, and maintainable manner. Let's explore the concept of namespaces, how to use them, and why they can be so beneficial.
What Are Namespaces?
Namespaces are a way to group related code under a single umbrella or 'namespace'. This way, you can prevent naming collisions and create a logical structure in your project, especially when dealing with multiple files and modules.
Imagine namespaces as drawers in a cupboard, each labeled for specific items. Instead of cluttering your cupboard with a jumble of items, you neatly categorize and store them in their respective drawers.
Using Namespaces
To declare a namespace, use the namespace
keyword followed by the name you wish to use.
namespace Utilities {
export function logMessage(message: string): void {
console.log(message);
}
export function logError(errorMessage: string): void {
console.error(errorMessage);
}
}
Utilities.logMessage("Hello from the Utilities namespace!");
Note the use of the export
keyword. If you want something (be it a variable, function, or class) to be accessible outside the namespace, you'll need to explicitly export it.
Splitting Across Multiple Files
For larger projects, you might have a namespace that spans multiple files. In such cases, the order in which scripts are loaded becomes significant.
Here's how you can split a namespace:
Validation.ts
namespace Validation {
export const isEmailValid = (email: string): boolean => {
// ... email validation logic
}
}
Registration.ts
/// <reference path="Validation.ts" />
namespace Validation {
export const isUsernameValid = (username: string): boolean => {
// ... username validation logic
}
}
Using the /// <reference path="..." />
directive tells the compiler about dependencies between files.
Namespaces vs Modules
As the TypeScript ecosystem evolved, especially with ES6's module system's advent, the line between "namespaces" and "modules" became evident.
-
Modules: With ES6 modules, each file is its module. If you're using
import
andexport
in your TypeScript file, you're working with modules. -
Namespaces: These are specific to TypeScript and are mainly used in scripts where all the code is on a single global scope.
For modern TypeScript development, especially with tools like Webpack and frameworks like Angular or React, modules are the recommended way to organize code. However, understanding namespaces is still valuable, especially if you work on legacy TypeScript projects.
Conclusion
Namespaces offer a convenient way to bundle up related functionalities and ensure clean, collision-free code in larger TypeScript applications. While they've taken a back seat with ES6 modules becoming the standard, they remain an essential tool in the TypeScript toolkit, especially for those maintaining or interacting with older codebases. So, the next time you're wading through a TypeScript project, appreciate the clarity and structure namespaces (or modules) bring to the table!
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
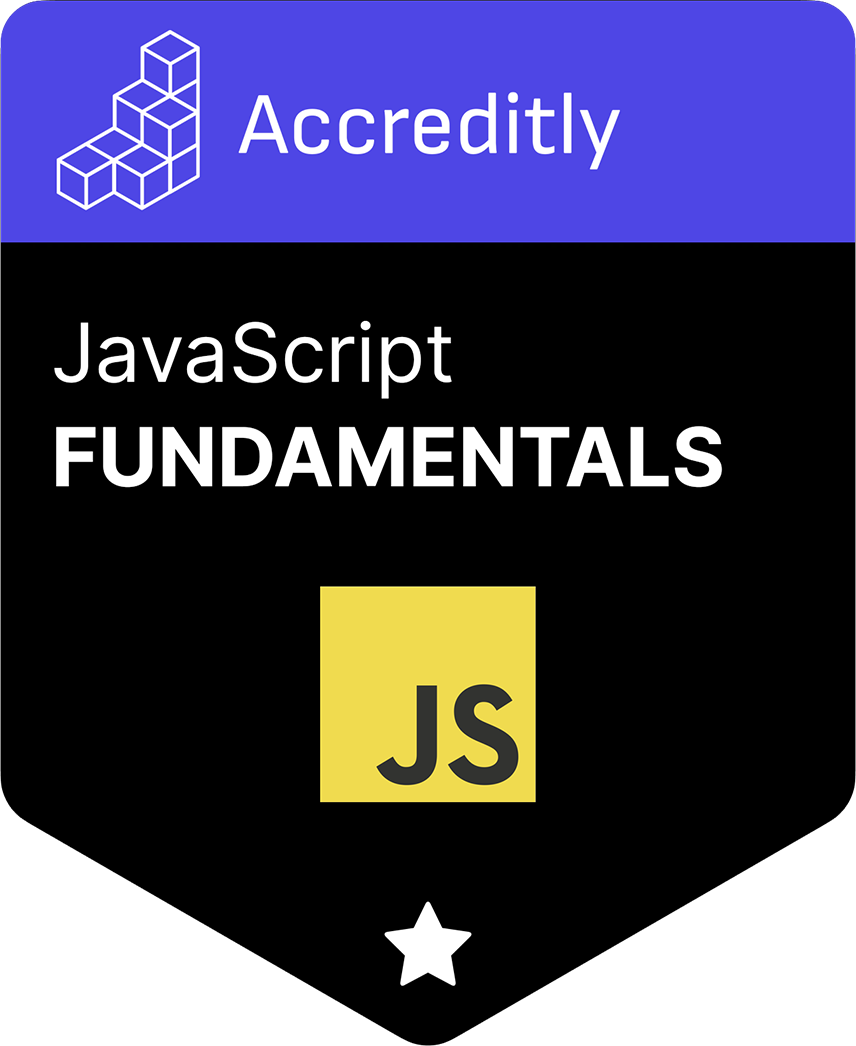