- Understanding the MouseEvent Object
- Setting Up Event Listeners
- Detecting Specific Mouse Buttons
- Preventing Default Actions for Right Clicks
- Compatibility and Considerations
Interpreting mouse clicks—beyond the simple left click—opens up a new dimension of interactivity for web applications. From context menus triggered by a right-click to actions initiated by a middle-click or even custom functionalities assigned to mice with more than three buttons, the ability to discern between different mouse buttons is crucial. JavaScript's mousedown
event makes it possible to detect these clicks, offering developers the capability to create nuanced and responsive user interfaces. This article guides you through detecting mousedown
events for various mouse buttons, including right, middle clicks, and more.
MouseEvent
Object
Understanding the The MouseEvent
object in JavaScript contains specific properties that provide information about the mouse event that occurred. For detecting different mouse buttons, the button
property of the MouseEvent
object is particularly useful. It returns a number indicating which button was pressed:
- 0: Left button
- 1: Middle button (wheel button)
- 2: Right button
- 3: Browser Back button (not always supported)
- 4: Browser Forward button (not always supported)
Setting Up Event Listeners
To detect various mouse button clicks, you first need to set up an event listener for the mousedown
event. This can be done on the document
object or on specific elements where you want to detect mouse clicks.
document.addEventListener('mousedown', function(event) {
console.log(`Mouse button pressed: ${event.button}`);
});
Detecting Specific Mouse Buttons
Within the event listener callback function, you can use the event.button
property to determine which mouse button was pressed and execute different actions based on the button.
document.addEventListener('mousedown', function(event) {
switch (event.button) {
case 0:
console.log('Left button clicked.');
break;
case 1:
console.log('Middle button clicked.');
break;
case 2:
console.log('Right button clicked.');
break;
case 3:
console.log('Browser Back button clicked.');
break;
case 4:
console.log('Browser Forward button clicked.');
break;
default:
console.log('Unknown button clicked.');
}
});
Preventing Default Actions for Right Clicks
Browsers typically assign default actions to certain mouse clicks, such as displaying the context menu on a right-click. If your application needs to override the default behavior for the right mouse button, you can use the event.preventDefault()
method.
document.addEventListener('mousedown', function(event) {
if (event.button === 2) { // Right button
event.preventDefault();
console.log('Right click detected; default context menu prevented.');
// Implement custom logic here
}
});
Compatibility and Considerations
While detecting mouse clicks using the button
property is widely supported, there are a few considerations to keep in mind:
- Ensure to test your mouse event handling across different browsers to confirm consistent behavior, especially for back and forward button detections which might not be universally supported.
- Overriding default browser behaviors (like the right-click context menu) should be done sparingly and only when it significantly enhances the user experience or is necessary for the application’s functionality.
- Relying solely on mouse interactions can impact accessibility. Always provide alternative methods for interacting with your application, especially for critical functionalities.
Detecting different mouse buttons in JavaScript allows for a richer, more interactive web experience. Whether it's enhancing navigational efficiency with middle-click actions, offering custom context menus with right-clicks, or utilizing additional mouse buttons for specific functions, the ability to distinguish between these inputs is a powerful tool in a web developer's arsenal. By leveraging the mousedown
event and the MouseEvent
object's button
property, developers can unlock sophisticated interaction patterns, making web applications more intuitive and engaging for users.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
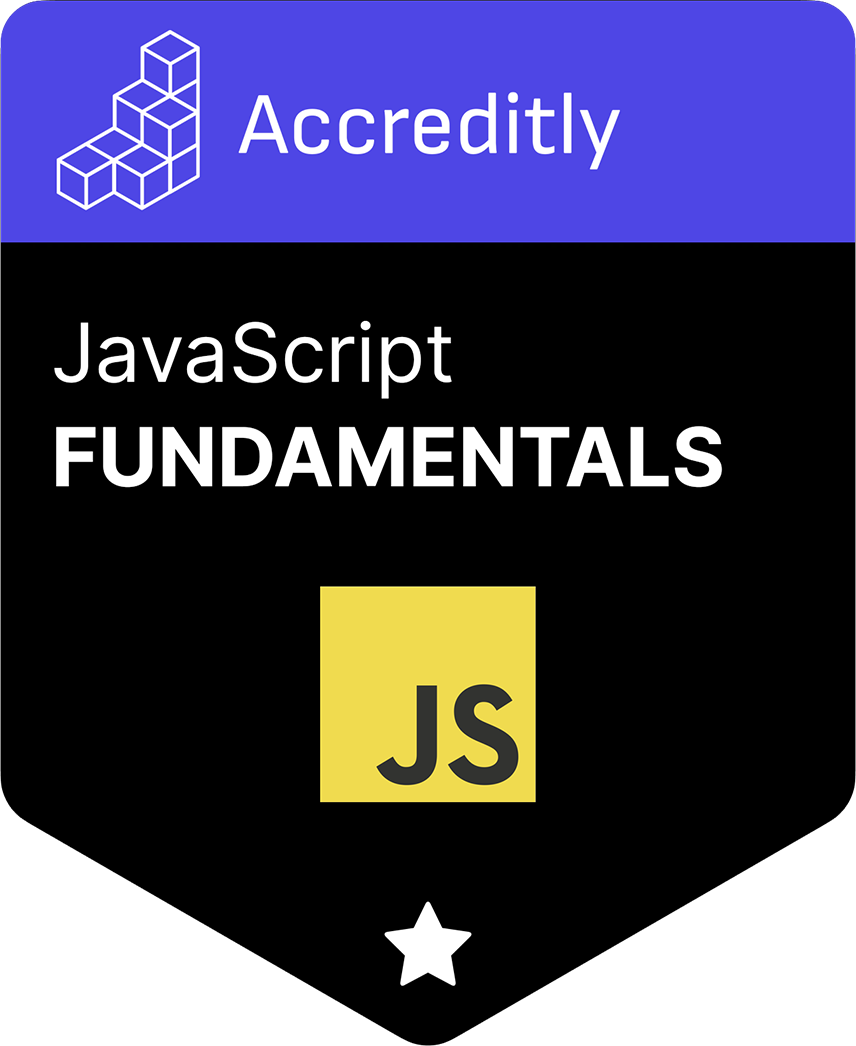