- Prerequisites
- Understanding WordPress User Roles and Capabilities
- Step 1: Using a Plugin for Frontend Post Editing
- Step 2: Creating a Custom Frontend Editing Form
- Step 3: Adding Security Measures
- Final Take
Allowing users to edit posts directly from the frontend of your WordPress website can enhance the user experience and streamline content management. This feature is especially useful for websites with multiple contributors or for user-generated content platforms. In this article, we'll explore different methods to enable frontend post editing, including creating custom forms, managing permissions, and using plugins.
Prerequisites
Before diving into the implementation, ensure you have the following:
- A WordPress website set up and running.
- Basic knowledge of WordPress themes and plugins.
- Familiarity with WordPress user roles and capabilities.
Understanding WordPress User Roles and Capabilities
WordPress has a built-in user role management system, where each role has specific capabilities. For example, authors can write and manage their own posts, while editors can manage all posts. For more details, refer to the WordPress Roles and Capabilities documentation.
Step 1: Using a Plugin for Frontend Post Editing
The easiest way to enable frontend post editing is by using a plugin. Several plugins can help you achieve this without writing custom code.
Recommended Plugin: WP User Frontend
WP User Frontend is a popular plugin that allows users to submit and edit posts from the frontend. It provides a simple interface and a variety of customization options.
Installation and Setup:
-
Install the Plugin: Go to Plugins > Add New in your WordPress dashboard, search for "WP User Frontend," and install it.
-
Activate the Plugin: Once installed, activate the plugin.
-
Configure the Plugin: Go to WP User Frontend > Settings to configure the plugin. You can set permissions, customize forms, and more.
-
Create a Frontend Form: Use the plugin to create a form for post editing. Go to WP User Frontend > Post Forms and click Add New to create a new form.
-
Display the Form on the Frontend: Use the shortcode provided by the plugin to display the form on a page. For example:
[wpuf_edit]
Step 2: Creating a Custom Frontend Editing Form
If you prefer a more customized solution, you can create your own frontend editing form. This involves creating a custom page template and handling form submissions.
Creating the Frontend Form
-
Create a Custom Page Template: Add a new file to your theme directory, e.g.,
page-edit-post.php
.<?php /* Template Name: Edit Post */ get_header(); // Check if user is logged in if (is_user_logged_in()) { // Get the current user $current_user = wp_get_current_user(); // Get the post ID from the query string $post_id = isset($_GET['post_id']) ? intval($_GET['post_id']) : 0; // Fetch the post $post = get_post($post_id); // Check if the post exists and the current user is the author if ($post && $post->post_author == $current_user->ID) { ?> <form method="post"> <input type="hidden" name="post_id" value="<?php echo $post_id; ?>"> <p> <label for="post_title">Title</label> <input type="text" id="post_title" name="post_title" value="<?php echo esc_attr($post->post_title); ?>"> </p> <p> <label for="post_content">Content</label> <textarea id="post_content" name="post_content"><?php echo esc_textarea($post->post_content); ?></textarea> </p> <p> <input type="submit" name="submit" value="Update Post"> </p> </form> <?php } else { echo '<p>You do not have permission to edit this post.</p>'; } } else { echo '<p>Please <a href="' . wp_login_url() . '">log in</a> to edit your post.</p>'; } get_footer(); ?>
-
Handle Form Submission: Add code to handle the form submission. Place this code in your theme’s
functions.php
file or a custom plugin.function handle_frontend_post_edit() { if (isset($_POST['submit'])) { // Verify nonce for security if (!isset($_POST['_wpnonce']) || !wp_verify_nonce($_POST['_wpnonce'], 'frontend-edit')) { return; } $post_id = intval($_POST['post_id']); $post_title = sanitize_text_field($_POST['post_title']); $post_content = sanitize_textarea_field($_POST['post_content']); // Update the post wp_update_post([ 'ID' => $post_id, 'post_title' => $post_title, 'post_content' => $post_content, ]); // Redirect to the post wp_redirect(get_permalink($post_id)); exit; } } add_action('init', 'handle_frontend_post_edit');
Step 3: Adding Security Measures
When enabling frontend post editing, it’s crucial to ensure the process is secure. Here are some tips:
-
Nonce Verification: Use WordPress nonces to protect your form from CSRF attacks. Add a nonce field to your form:
wp_nonce_field('frontend-edit');
Verify the nonce in your form handling code:
if (!isset($_POST['_wpnonce']) || !wp_verify_nonce($_POST['_wpnonce'], 'frontend-edit')) { return; }
-
User Permissions: Ensure that only authorized users can edit their posts. Check the post author against the current user’s ID, and use WordPress capabilities to control access.
$post = get_post($post_id); if ($post->post_author != get_current_user_id()) { wp_die('You are not allowed to edit this post.'); }
Final Take
Enabling frontend post editing in WordPress enhances the user experience and can streamline content management on your site. Whether you choose to use a plugin like WP User Frontend or create a custom solution, it's important to handle permissions and security properly.
For more detailed information, refer to the WordPress Roles and Capabilities documentation and the WordPress Plugin Handbook. By following these steps, you can create a user-friendly and secure environment for your contributors.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
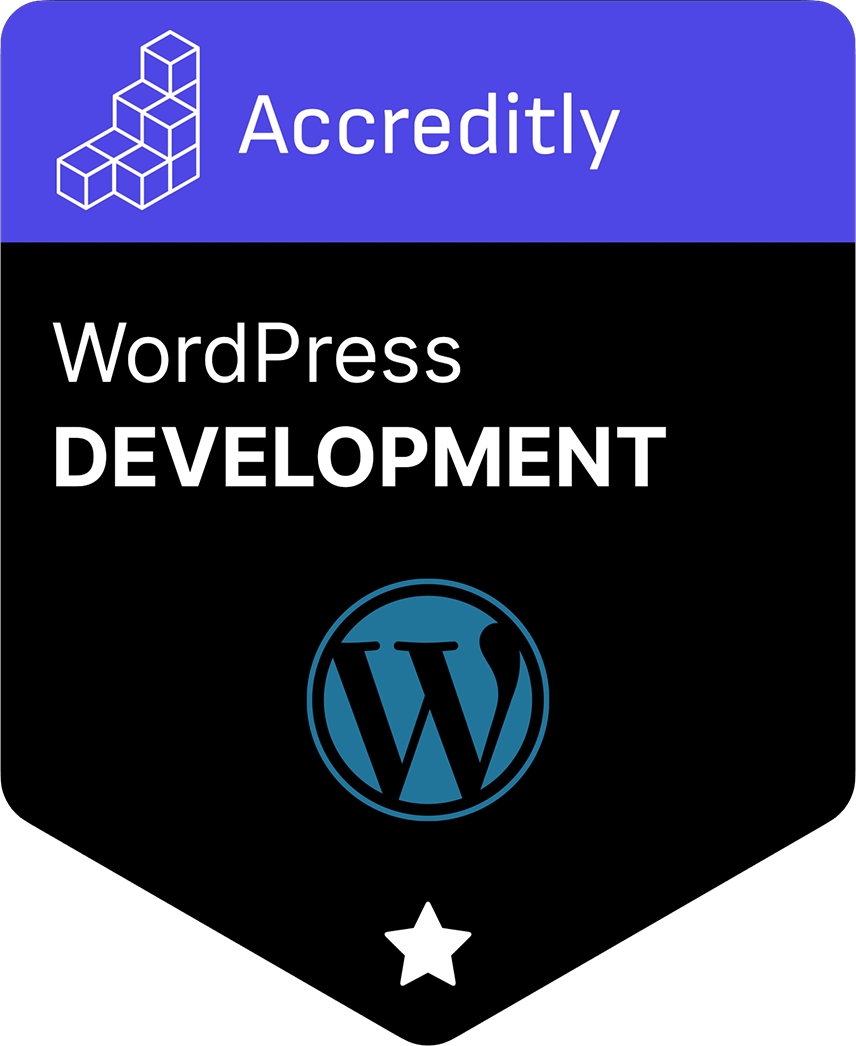