In PHP, one might occasionally encounter the double not operator (!!
) and be left scratching their head. It's a syntax that is not commonly used, but when applied, it can be a handy tool for specific use cases. This article aims to demystify the double not operator in PHP by explaining what it does and when you might want to use it.
!
Single not operator: Before we jump into the double not operator, it's important to understand its single counterpart: the not operator (!
). In PHP, the not operator is a logical operator that inverts the truthiness of a variable or expression. If the variable is truthy (true
when converted to a boolean), !
will return false. If the variable is falsy (false
when converted to a boolean), !
will return true.
$var = true;
echo !$var; // Outputs: false
$var = false;
echo !$var; // Outputs: true
!!
Double not operator: The double not operator (!!
) in PHP is simply the not operator applied twice. The first !
inverts the truthiness of the variable, and the second !
inverts it back. So, why would you want to do this?
$var = 'Hello, World!';
$bool = !!$var;
var_dump($bool); // Outputs: bool(true)
In this example, 'Hello, World!'
is a truthy value. The first !
converts it to false
, and the second !
converts it back to true
.
The main benefit of the double not operator is that it effectively converts any variable to its boolean equivalent. This can be incredibly handy if you need to explicitly ensure a variable is a boolean value for comparison purposes or to satisfy a function's expected parameters.
When to use double not operator
Let's look at a practical example. Suppose you have a function that expects a boolean parameter. If you pass a non-boolean value, the function may behave unexpectedly. By using the double not operator, you can ensure that you're always passing a boolean value.
function isUserLoggedIn($isLoggedIn) {
if ($isLoggedIn) {
echo 'Welcome, user!';
} else {
echo 'Please log in.';
}
}
$isLoggedIn = 'yes'; // This could come from a form or API and might not always be a boolean.
isUserLoggedIn(!!$isLoggedIn);
In this case, even though $isLoggedIn
is a string, !!$isLoggedIn
ensures it is passed as a boolean to the isUserLoggedIn
function.
Should you use it?
The double not operator is a useful tool in PHP for explicitly converting values to their boolean equivalent. It's not a syntax you'll use in every project, but understanding how and when to use it can help you write cleaner, more predictable code.
Having said that, because it isn't often used it can make code less readable for developers who haven't come across it before. You should approach with caution, and follow the guidelines for whatever project you're working on.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
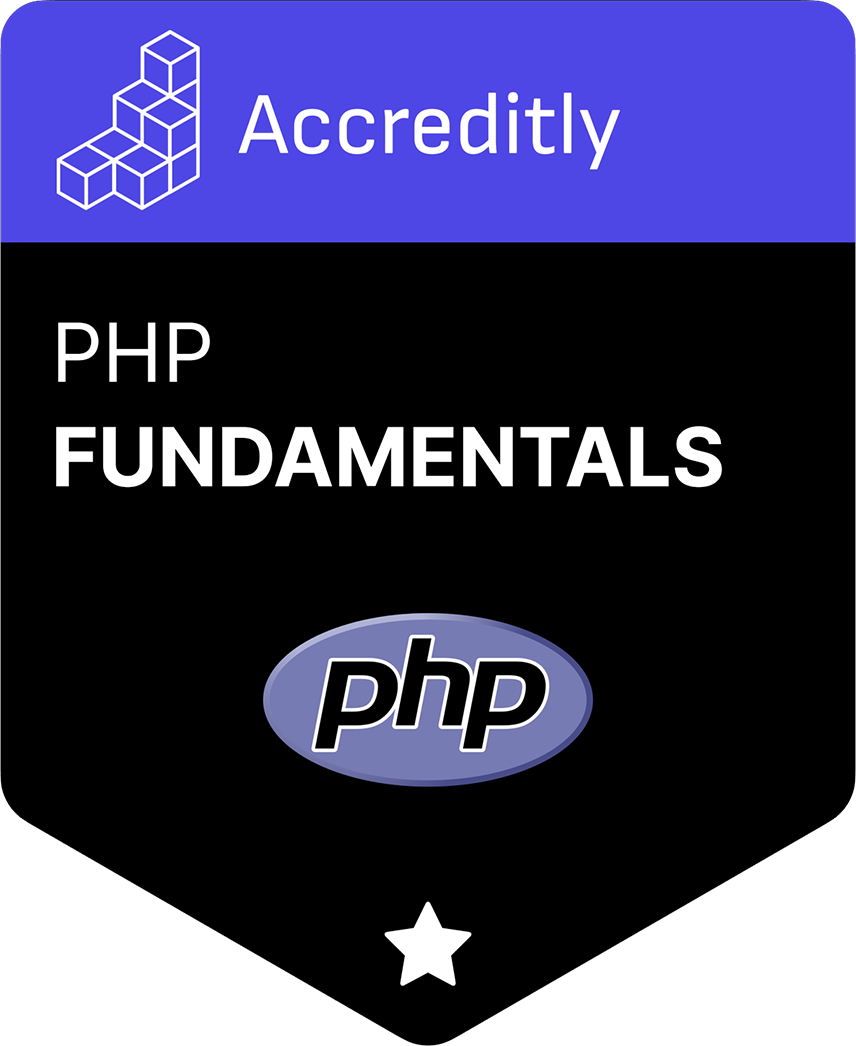