- Understanding Laravel's Task Scheduling
- Scheduling a Task for the Last Day of the Month
- Ensuring the Task Scheduler is Running
Laravel simplifies the process of task scheduling with its concise and expressive syntax, making it easier for developers to automate tasks such as database backups, email dispatches, or custom cleanup operations. A common requirement in many applications is the ability to schedule a task to run on the last day of the month, regardless of the month's length. This article explores how to implement this specific scheduling requirement in Laravel.
Understanding Laravel's Task Scheduling
Laravel's task scheduling system, introduced in version 5.0, allows developers to define their command schedule within the application itself, rather than relying on the cron syntax. The scheduler is defined in the app/Console/Kernel.php
file's schedule
method.
Before diving into scheduling tasks for the last day of the month, ensure you have a basic understanding of creating console commands in Laravel, as scheduled tasks are typically defined as console commands.
Scheduling a Task for the Last Day of the Month
To schedule a task for the last day of each month, Laravel offers a method called when()
, which allows you to define a closure that determines if the scheduled task should run based on a true or false return value. By combining this with PHP's date functions, you can effectively schedule your task for the end of the month.
First, create your console command using Artisan:
php artisan make:command EndOfMonthTask
This command will generate a new command class in the app/Console/Commands
directory. You can define your task logic within the handle()
method of this class.
Next, open your app/Console/Kernel.php
file to schedule the task. In the schedule
method, use the when()
method to check if today is the last day of the month:
protected function schedule(Schedule $schedule)
{
$schedule->command('command:endOfMonthTask')
->daily()
->when(function () {
return now()->endOfMonth()->isToday();
});
}
In this example, command:endOfMonthTask
should be replaced with the name you defined for your command in the signature
property of your command class.
Explanation:
-
->daily()
: This method schedules the task to run daily. -
->when(function () { ... })
: This method allows us to specify a custom condition for when the task should run. Inside the closure, we returntrue
if today is the last day of the month, indicating that the task should execute.
How It Works:
-
now()
is a helper function in Laravel that returns a Carbon instance for the current date and time. -
endOfMonth()
is a Carbon method that returns a copy of the instance set to the last moment of the current month. -
isToday()
checks if the instance (the last day of the month) is equal to today's date.
Ensuring the Task Scheduler is Running
For the scheduler to run, you must add the following Cron entry to your server, which executes the Laravel scheduler every minute:
* * * * * cd /path-to-your-project && php artisan schedule:run >> /dev/null 2>&1
This Cron entry calls the schedule:run
command of Artisan every minute, which in turn evaluates scheduled tasks and runs them if their conditions are met.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
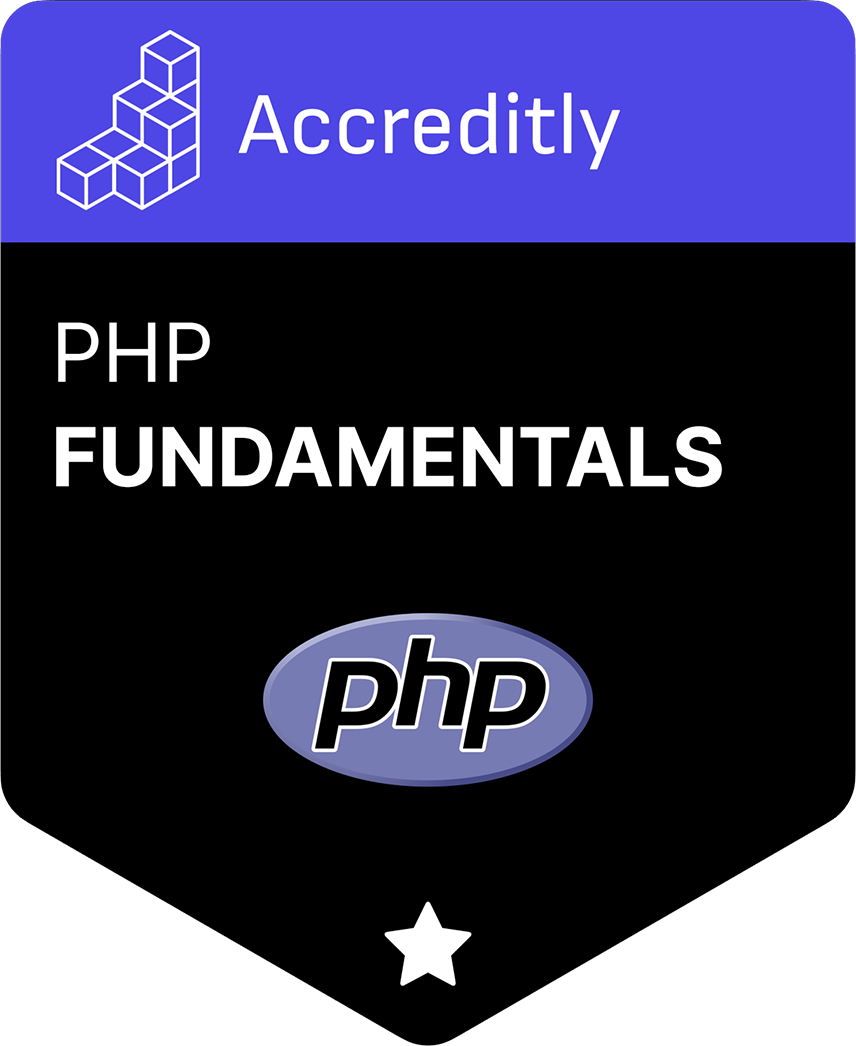