- Before you begin
- Step 1: Registering and Displaying Menus
- Step 2: Creating and Managing Menus in the WordPress Admin
- Step 4: Customizing Menu Output with Walker Classes
- Step 4: Styling and Enhancing Menus with JavaScript
- Summary
Menus are an essential part of any WordPress website, allowing users to navigate through different pages and sections with ease. As a developer, understanding how to create, manage, and customise menus in WordPress is crucial for providing an excellent user experience. In this guide, we'll explore how to properly manage and build menus in WordPress using built-in features, custom code, and best practices.
Before you begin
This tutorial expects you to have a basic knowledge of managing your WordPress theme files and that you are comfortable within the WordPress admin. Although we discuss creating menus within WordPress admin, learning how to do that is outside of the scope of this tutorial.
Also remember WordPress best practices. Wherever we mention a new function we will prefix the function name with mytheme_
, you should update this to be the name of your theme or plugin.
If all of this sounds obvious to you then you might be interested in our WordPress Development Certification which focuses on WordPress best practices, theme and plugin development.
Step 1: Registering and Displaying Menus
WordPress provides a built-in menu management system, which allows you to register and display custom menus in your theme. To get started, follow these steps:
-
Register your menu: In your theme's
functions.php
file, register the custom menu location using theregister_nav_menus()
function. This allows you to assign menus to specific locations in your theme.
function mytheme_register_menus() {
register_nav_menus(
array(
'primary' => __( 'Primary Menu', 'mytheme' ),
'footer' => __( 'Footer Menu', 'mytheme' ),
)
);
}
add_action( 'init', 'mytheme_register_menus' );
In this example, we registered two menu locations: primary for the main navigation and footer for the footer navigation.
-
Display the menu in your theme: To display the menu in your theme, use the
wp_nav_menu()
function, specifying the desired menu location. Add the following code to the appropriate template file (e.g.,header.php
for the primary menu orfooter.php
for the footer menu).
wp_nav_menu(
array(
'theme_location' => 'primary',
'container' => 'nav',
'container_class' => 'main-navigation',
)
);
This code snippet will display the primary menu within a <nav>
element with the class main-navigation. You can customise the output further by modifying the wp_nav_menu()
parameters.
wp_nav_menu()
accepts many arguments for customisation, check out the docs for more info.
Step 2: Creating and Managing Menus in the WordPress Admin
Once you've registered and displayed your menu locations in your theme, you can create and manage menus using the WordPress admin interface.
- Create a new menu: To create a new menu, navigate to Appearance > Menus in the WordPress admin area. Click "create a new menu," enter a name for your menu, and click "Create Menu."
- Add items to the menu: On the left side of the Menus screen, you'll find panels containing different types of menu items, such as Pages, Posts, Custom Links, and Categories. Click the checkboxes next to the items you want to add to your menu, and then click "Add to Menu." You can also add custom links by expanding the "Custom Links" panel, entering a URL and link text, and clicking "Add to Menu."
- Organise menu items: You can easily arrange your menu items by dragging and dropping them into the desired order. To create a submenu, simply drag a menu item to the right, and it will become a child of the item above it.
- Assign the menu to a location: In the "Menu Settings" section, you'll find a list of available menu locations in your theme. Check the box next to the location where you want your menu to appear (e.g., "Primary Menu" or "Footer Menu"), and then click "Save Menu."
Check out the docs for WordPress menus.
Step 4: Customizing Menu Output with Walker Classes
By default, WordPress generates menu markup using the built-in Walker_Nav_Menu
class. However, you might want to customise the output to meet specific design or functionality requirements. To do this, you can extend the Walker_Nav_Menu
class and override its methods to generate custom menu markup.
-
Create a custom walker class: In your theme's functions.php file or a separate PHP file, create a new class that extends
Walker_Nav_Menu
. Override the necessary methods to customise your menu output. Here's an example of a custom walker class that adds a custom class to each menu item:
class MyTheme_Custom_Walker extends Walker_Nav_Menu {
function start_el( &$output, $item, $depth = 0, $args = array(), $id = 0 ) {
$classes = empty( $item->classes ) ? array() : (array) $item->classes;
$classes[] = 'mytheme-custom-menu-item';
$class_names = join( ' ', apply_filters( 'nav_menu_css_class', array_filter( $classes ), $item, $args, $depth ) );
$class_names = $class_names ? ' class="' . esc_attr( $class_names ) . '"' : '';
$output .= '<li' . $class_names . '>';
$atts = array();
$atts['title'] = ! empty( $item->attr_title ) ? $item->attr_title : '';
$atts['target'] = ! empty( $item->target ) ? $item->target : '';
$atts['rel'] = ! empty( $item->xfn ) ? $item->xfn : '';
$atts['href'] = ! empty( $item->url ) ? $item->url : '';
$atts = apply_filters( 'nav_menu_link_attributes', $atts, $item, $args, $depth );
$attributes = '';
foreach ( $atts as $attr => $value ) {
if ( ! empty( $value ) ) {
$value = ( 'href' === $attr ) ? esc_url( $value ) : esc_attr( $value );
$attributes .= ' ' . $attr . '="' . $value . '"';
}
}
$title = apply_filters( 'the_title', $item->title, $item->ID );
$item_output = $args->before;
$item_output .= '<a' . $attributes . '>';
$item_output .= $args->link_before . $title . $args->link_after;
$item_output .= '</a>';
$item_output .= $args->after;
$output .= apply_filters( 'walker_nav_menu_start_el', $item_output, $item, $depth, $args );
}
}
-
Apply the custom walker to your menu: To use your custom walker class, update the
wp_nav_menu()
function in your theme template file, adding the 'walker
' parameter:
wp_nav_menu(
array(
'theme_location' => 'primary',
'container' => 'nav',
'container_class' => 'main-navigation',
'walker' => new MyTheme_Custom_Walker(),
)
);
By using the 'walker
' parameter, you're telling WordPress to use your custom MyTheme_Custom_Walker
class to generate the menu markup, which now includes the custom class for each menu item.
Step 4: Styling and Enhancing Menus with JavaScript
After customising your menu structure, you may need to apply additional styling or add JavaScript enhancements for improved user experience. This could include CSS for responsive designs or JavaScript for dropdown menus, off-canvas navigation, or accessibility enhancements.
- Add CSS styles: In your theme's stylesheet, add custom CSS styles targeting the menu classes generated by your walker class or the default WordPress classes.
-
Add JavaScript enhancements: Enqueue a custom JavaScript file in your theme's
functions.php
file usingwp_enqueue_script()
and write your JavaScript code to add interactivity or other enhancements to your menu.
Summary
Creating and managing menus in WordPress involves registering and displaying menu locations, using the WordPress admin interface, customising menu output with walker classes, and adding styles and JavaScript enhancements. By mastering these techniques, you can provide an excellent user experience and ensure your WordPress website's menus meet your design and functionality requirements.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
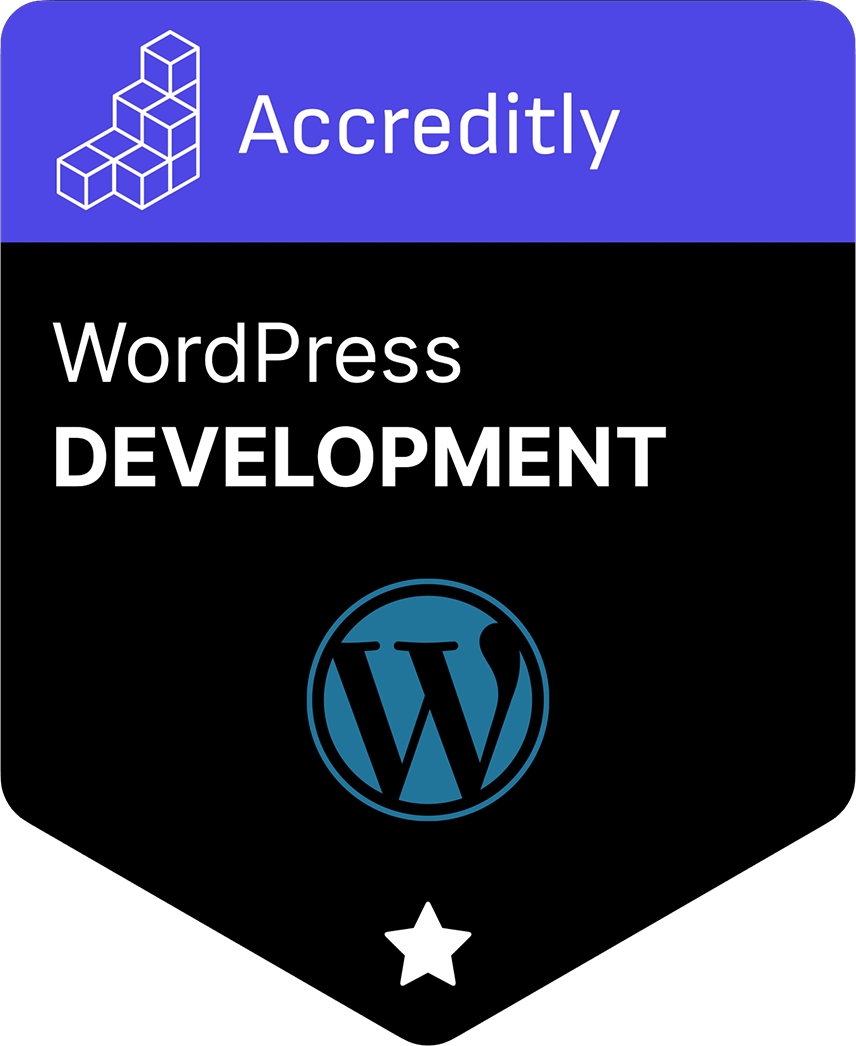