- What is findMany?
- Basic Usage of findMany
- Why Use findMany?
- Advanced Usage with Conditions
- Handling Results
- When to Use findMany
In Laravel, Eloquent ORM provides an elegant and efficient way to interact with databases. Among its rich set of functionalities, findMany
stands out as a powerful method for retrieving multiple records with a single query. Understanding how to use findMany
can significantly streamline your data fetching process. Let’s delve into the nuances of findMany
and how to leverage it in Laravel applications. Before we begin, you may find it useful to look at Laravel's API docs for findMany
, as well as checking out our article on useful but little-known Laravel methods.
findMany
?
What is findMany
is a method in Laravel's Eloquent ORM that allows you to retrieve multiple models based on an array of primary key values. It's a streamlined way to fetch a collection of records without needing to loop through multiple find
operations.
findMany
Basic Usage of The findMany
method is used on an Eloquent model and takes an array of primary key values as its argument. Here's a basic example of its usage:
use App\Models\User;
$users = User::findMany([1, 2, 3]);
In this example, findMany
retrieves users with the IDs 1, 2, and 3 from the database.
findMany
?
Why Use -
Performance Efficiency:
findMany
condenses what could be multiple database queries into a single query, reducing the overhead and improving performance. - Code Simplicity: It simplifies your code, making it cleaner and more readable.
- Reduced Database Load: By minimizing the number of queries, it reduces the load on your database, which can be crucial for applications with heavy data-fetching requirements.
Advanced Usage with Conditions
findMany
can be combined with other Eloquent methods to add conditions to your query. For example, if you only want to fetch users who are active:
$activeUsers = User::where('active', 1)->findMany([1, 2, 3]);
This query fetches users with IDs 1, 2, and 3, but only if they are marked as 'active' in the database.
Handling Results
The findMany
method returns an Eloquent collection, allowing you to use Laravel's collection methods, such as each
, map
, and filter
, to manipulate the results:
$users = User::findMany([1, 2, 3]);
$users->each(function ($user) {
echo $user->name;
});
findMany
When to Use findMany
is particularly useful when:
- You have a known set of primary key values to fetch.
- You need to efficiently retrieve multiple records in a single query.
- You are looking to maintain clean and maintainable code.
Laravel's findMany
method is an invaluable tool for efficiently fetching multiple records with Eloquent. By utilizing findMany
, you can write more performant, cleaner, and more readable code, enhancing both the back-end efficiency and the maintainability of your Laravel applications.
Laravel has a number of lesser-known methods available that aren't necessarily found in the main documentation. Check out our article on the lesser-known Laravel methods for a more comprehensive list.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
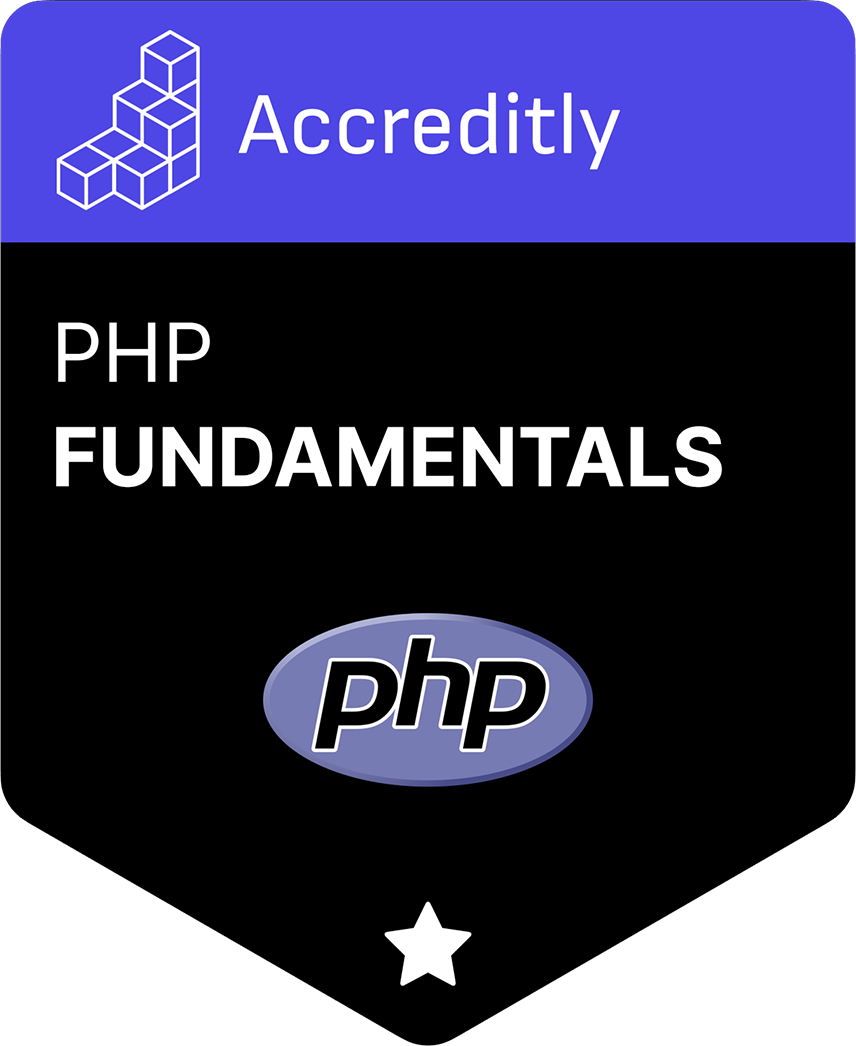