- 1. Displaying a Payment Form on the Frontend
- 2. Handling Form Submission and Saving Payment in the Database
- 3. Handling the PayPal Webhook and Updating the Payment Row in the Database
- Security and Validation Considerations
Integrating PayPal payments into your WordPress site doesn't always require the use of plugins. For those seeking a more hands-on approach or looking to reduce their site's plugin dependency, manually setting up PayPal payments is a viable option. This method offers greater control over the payment process, from the initial setup to handling PayPal webhooks and managing payment information within WordPress. This article will guide you through each step of taking PayPal payments on your WordPress site without relying on plugins.
If you're looking for another way to take payments, take a look at how to take payments in WordPress using Stripe without plugins.
1. Displaying a Payment Form on the Frontend
First, you need a form through which users can initiate a payment. Place this HTML form in your WordPress theme file where you want the payment form to appear, such as in a custom page template or a specific section of your site.
<!-- Place this in your theme's file where you want the form to appear -->
<form id="paypal-payment-form" action="/path-to-your-submission-handler" method="POST">
<input type="text" name="amount" placeholder="Amount" required>
<input type="email" name="payer_email" placeholder="Your Email" required>
<input type="submit" value="Pay with PayPal">
</form>
2. Handling Form Submission and Saving Payment in the Database
You need to handle the form submission on the server side. This involves capturing the form data and saving it to a custom table in your WordPress database before redirecting to PayPal.
First, ensure you have a custom table ready for storing payments. Here’s a simplified SQL statement to create a table. You can run this via a database management tool like phpMyAdmin, or programmatically during your theme/plugin activation hook.
CREATE TABLE wp_custom_payments (
id BIGINT(20) UNSIGNED AUTO_INCREMENT PRIMARY KEY,
amount DECIMAL(10, 2) NOT NULL,
payer_email VARCHAR(255) NOT NULL,
status VARCHAR(20) NOT NULL DEFAULT 'pending',
paypal_payment_id VARCHAR(255) DEFAULT NULL,
created_at DATETIME DEFAULT CURRENT_TIMESTAMP
);
Next, add the following code to your theme's functions.php
file or in a custom plugin. This code snippet captures the form submission, saves the payment details to your custom table, and then redirects to PayPal for payment completion.
add_action('template_redirect', 'handle_paypal_form_submission');
function handle_paypal_form_submission() {
if (isset($_POST['amount']) && isset($_POST['payer_email'])) {
global $wpdb;
$amount = sanitize_text_field($_POST['amount']);
$payer_email = sanitize_email($_POST['payer_email']);
// Insert payment record into the database
$wpdb->insert(
$wpdb->prefix . 'custom_payments',
[
'amount' => $amount,
'payer_email' => $payer_email,
'status' => 'pending' // Default status
],
['%f', '%s', '%s']
);
$payment_id = $wpdb->insert_id;
// Redirect to PayPal for payment (simplified example, implement your actual PayPal payment link)
wp_redirect("https://www.paypal.com/cgi-bin/webscr?cmd=_pay-inv&id=PAYMENT_ID_FROM_PAYPAL");
exit;
}
}
3. Handling the PayPal Webhook and Updating the Payment Row in the Database
Once the payment is made, PayPal can send a webhook notification to a specified URL in your application. You need to handle this webhook to update the payment status in your database.
First, configure a webhook listener URL in PayPal Developer Dashboard and point it to a specific endpoint on your WordPress site.
Next, add the following code to handle the incoming webhook and update the payment status. Place this code in your theme’s functions.php
file or a custom plugin.
add_action('init', 'handle_paypal_webhook');
function handle_paypal_webhook() {
if ($_SERVER['REQUEST_METHOD'] == 'POST' && $_SERVER['REQUEST_URI'] == '/paypal-webhook-listener') {
$payload = file_get_contents('php://input');
$data = json_decode($payload, true);
// Assume $data contains 'payment_id' and 'status'
global $wpdb;
$table_name = $wpdb->prefix . 'custom_payments';
// Validate and verify the webhook payload with PayPal
// Update the payment status based on the webhook data
$wpdb->update(
$table_name,
['status' => sanitize_text_field($data['status'])], // Example: 'completed'
['id' => (int) $data['payment_id']], // Assuming 'payment_id' is how you correlate
['%s'],
['%d']
);
status_header(200);
exit;
}
}
This code is a simplified demonstration. The actual implementation should include validation of the webhook data with PayPal to confirm its authenticity.
Security and Validation Considerations
- Always validate and sanitize input data to protect against SQL injection and other security vulnerabilities.
- Verify the webhook data with PayPal to ensure the notification is legitimate.
- Implement CSRF protection for your form submission.
Integrating PayPal payments into WordPress without plugins involves direct handling of payment forms, server-side processing, and webhook management. This approach gives you full control over the payment process, enabling custom payment flows and data handling tailored to your needs. Remember, handling financial transactions requires attention to security, data validation, and regulatory compliance to provide a safe and reliable payment experience for your users.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
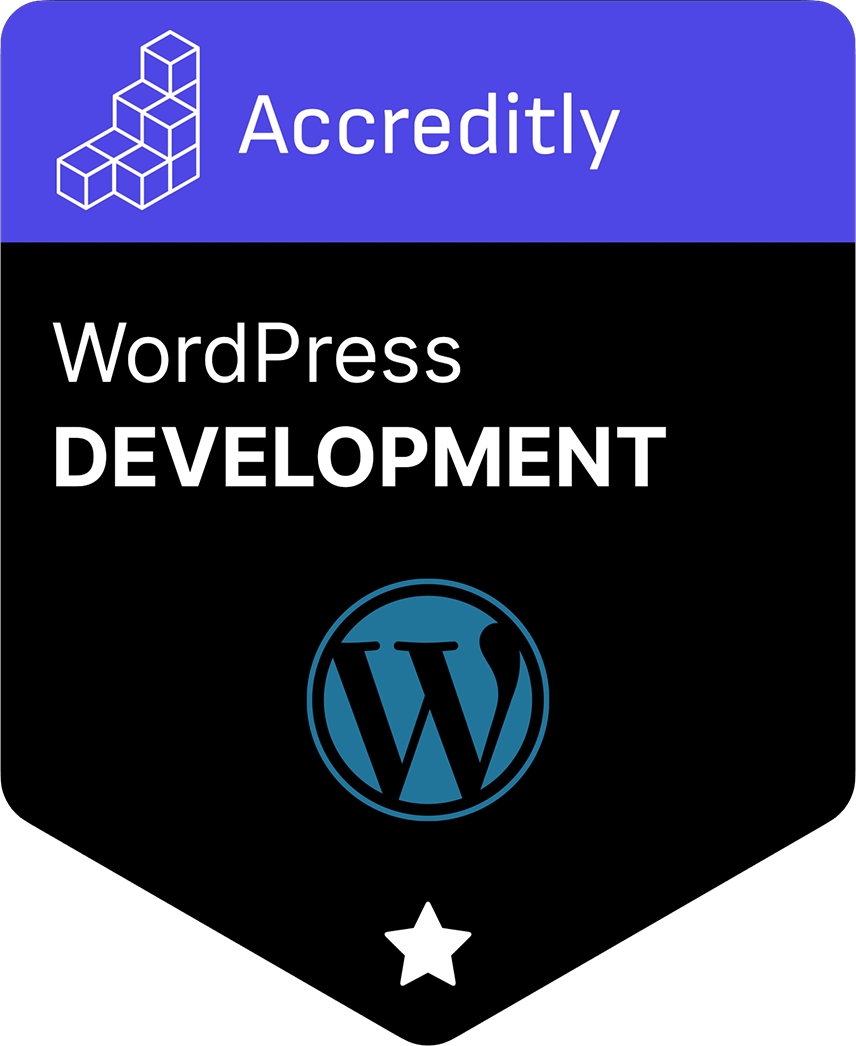
Related articles
Tutorials WordPress PHP Tooling
How to Build an Image Carousel in Gutenberg
Create an image carousel directly within the Gutenberg editor in WordPress. This step-by-step guide provides all the necessary insights and tips to enhance your posts and pages with visually captivating carousels.