- 1. Prerequisites:
- 2. Create a Stripe Payment Form:
- 3. Integrate Stripe.js:
- 4. Process the Payment:
- 5. Confirming the Payment on Frontend:
- 6. Handling Post-Payment Actions:
Online payments have become an integral part of e-commerce, and Stripe stands out as one of the most popular and secure payment gateways. While many WordPress users rely on plugins to integrate Stripe, it's entirely possible—and sometimes more flexible—to set up Stripe manually. Here's how to do it.
1. Prerequisites:
Before you begin, ensure you have:
- A Stripe account. You'll need to ensure it's activated before you can take live payments, but you can build and test with a dev account immediately.
- Stripe API keys: Publishable Key and Secret Key which can be found in your Stripe dashboard.
- An SSL certificate installed on your WordPress site to ensure security during payment processes.
2. Create a Stripe Payment Form:
First, we need to design a form that will take payment details. On a new page or post, input the following HTML:
<form action="/process-payment" method="post" id="stripe-payment-form">
<div>
<label for="name">Name</label>
<input type="text" id="name" required>
</div>
<div>
<label for="email">Email</label>
<input type="email" id="email" required>
</div>
<div>
<label>Card Info</label>
<div id="card-element"></div>
</div>
<button type="submit">Submit Payment</button>
</form>
Note the card-element
element. This will be populated with a Stripe payment form in the next step.
3. Integrate Stripe.js:
Stripe offers its JavaScript library, Stripe.js, which manages card details securely. Add it to your site by embedding the following script:
<script src="https://js.stripe.com/v3/"></script>
Now, let's set up Stripe elements for our payment form. Add this JavaScript below the previous script:
var stripe = Stripe('YOUR_PUBLISHABLE_KEY'); // Replace with your key
var elements = stripe.elements();
var card = elements.create('card');
card.mount('#card-element');
4. Process the Payment:
Once a user submits the form, you'll need to handle the payment on the server-side. This involves creating a payment intent, capturing details, and finalizing the payment.
To your site's functions.php
or a custom functionality plugin, add:
function process_stripe_payment() {
require_once('path_to_stripe_php_init.php'); // Get this from Stripe's PHP SDK
\Stripe\Stripe::setApiKey("YOUR_SECRET_KEY");
header('Content-Type: application/json');
$checkout_session = \Stripe\Checkout\Session::create([
'payment_method_types' => ['card'],
'line_items' => [[
'price_data' => [
'currency' => 'usd',
'unit_amount' => 2000, // $20.00, for example
'product_data' => [
'name' => 'Your Product Name',
],
],
'quantity' => 1,
]],
'mode' => 'payment',
'success_url' => 'https://yourdomain.com/success',
'cancel_url' => 'https://yourdomain.com/cancel',
]);
echo json_encode(['id' => $checkout_session->id]);
exit;
}
add_action('wp_ajax_process_stripe_payment', 'process_stripe_payment'); // If user is logged in
add_action('wp_ajax_nopriv_process_stripe_payment', 'process_stripe_payment'); // If user is not logged in
You'll need to install Stripe's PHP SDK and link to it in the first line. If you're using Composer to manage your dependencies this should be straightforward, otherwise you may need to manually download it.
5. Confirming the Payment on Frontend:
After creating the payment intent on the server, you should confirm it on the frontend:
const form = document.getElementById('stripe-payment-form');
form.addEventListener('submit', async (e) => {
e.preventDefault();
const {error, paymentIntent} = await stripe.confirmCardPayment(CLIENT_SECRET, {
payment_method: {card: cardElement}
});
if (error) {
console.log('Payment failed:', error);
} else if (paymentIntent.status === 'succeeded') {
console.log('Payment successful!');
}
});
It's up to you what you do here. You may wish to set up a dedicated success or failure page, or swap out content on the existing page. You may also have some features you want to implement post payment.
6. Handling Post-Payment Actions:
Once the payment is completed, you might want to:
- Display a thank-you page.
- Send a confirmation email to the customer.
- Update your order database.
Implement these as per your needs using WordPress actions and filters.
This guide provides a straightforward method to integrate Stripe into your WordPress site without plugins. While plugins can simplify the process, understanding the manual integration empowers you with more control and customization options for your e-commerce needs. Ensure you test thoroughly in Stripe's sandbox mode before going live.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
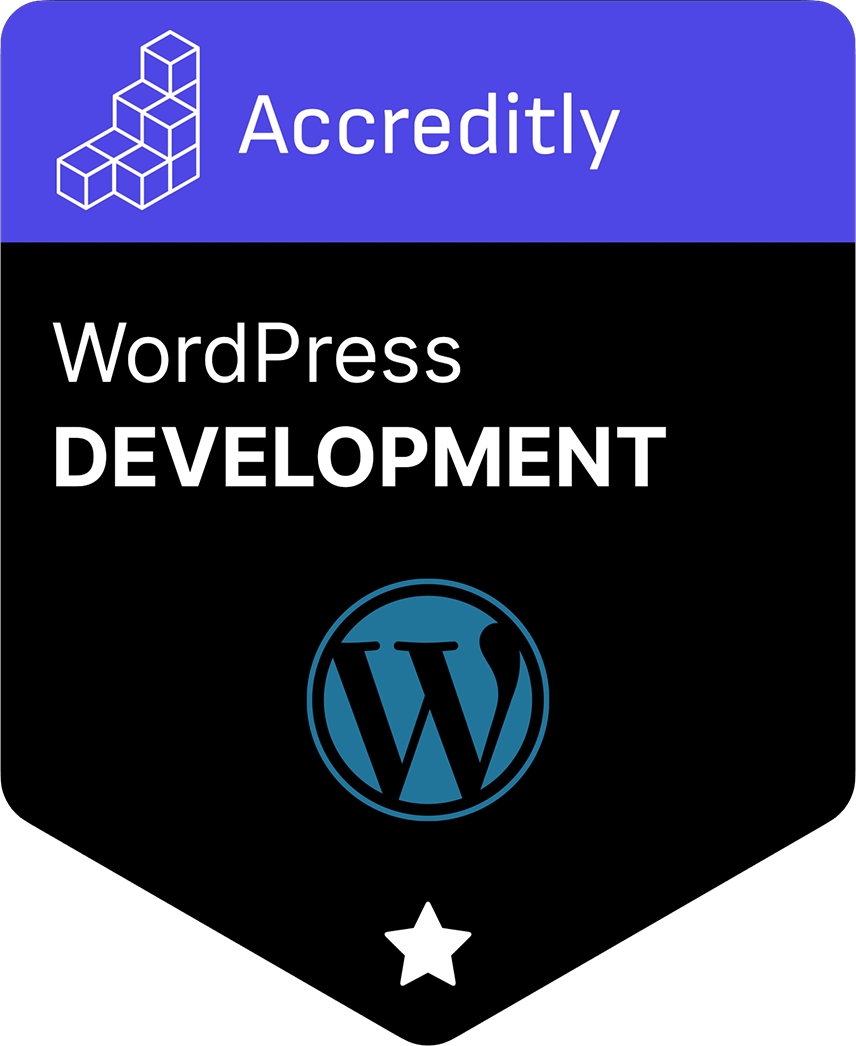