Phaser is a robust, versatile game development framework for creating interactive games that can run in any web browser. Phaser 3, the latest version, brings new features and improvements that make it even more powerful and easier to use for developers. This tutorial will guide you through the process of creating a basic Phaser 3 game from scratch, covering setup, game mechanics, and deployment.
Getting Started with Phaser 3
To begin, you need to set up your development environment for Phaser 3. Here’s how you can start:
Step 1: Setting Up Your Project
-
Create a New Directory for your project:
mkdir PhaserGame cd PhaserGame
-
Set Up a Local Server:
- Phaser games require a web server due to browser cross-origin policies. You can set up a simple server using Python or Node.js. For Python 3.x:
python -m http.server
- Navigate to
http://localhost:8000
in your browser to see the server running.
- Phaser games require a web server due to browser cross-origin policies. You can set up a simple server using Python or Node.js. For Python 3.x:
Step 2: Include Phaser
Include Phaser in your project by adding it to your HTML file. You can link directly to a Phaser build via CDN:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Phaser 3 Game</title>
<script src="https://cdn.jsdelivr.net/npm/phaser@3/dist/phaser.min.js"></script>
</head>
<body>
<script src="game.js"></script>
</body>
</html>
Creating Your First Game
Phaser 3 uses scenes to manage different parts of the game, like the main menu, game levels, and the game over screen. We’ll create a simple game scene with preload, create, and update functions.
Step 3: Create the Game Scene
-
Create a JavaScript file named
game.js
and set up your game scene:
var config = {
type: Phaser.AUTO,
width: 800,
height: 600,
physics: {
default: 'arcade',
arcade: {
gravity: { y: 300 },
debug: false
}
},
scene: {
preload: preload,
create: create,
update: update
}
};
var game = new Phaser.Game(config);
function preload() {
this.load.image('sky', 'assets/sky.png');
this.load.image('star', 'assets/star.png');
this.load.image('platform', 'assets/platform.png');
this.load.spritesheet('dude',
'assets/dude.png',
{ frameWidth: 32, frameHeight: 48 }
);
}
function create() {
this.add.image(400, 300, 'sky');
var platforms = this.physics.add.staticGroup();
platforms.create(400, 568, 'platform').setScale(2).refreshBody();
var player = this.physics.add.sprite(100, 450, 'dude');
player.setBounce(0.2);
player.setCollideWorldBounds(true);
this.physics.add.collider(player, platforms);
this.anims.create({
key: 'left',
frames: this.anims.generateFrameNumbers('dude', { start: 0, end: 3 }),
frameRate: 10,
repeat: -1
});
this.anims.create({
key: 'turn',
frames: [{ key: 'dude', frame: 4 }],
frameRate: 20
});
this.anims.create({
key: 'right',
frames: this.anims.generateFrameNumbers('dude', { start: 5, end: 8 }),
frameRate: 10,
repeat: -1
});
}
function update() {
var cursors = this.input.keyboard.createCursorKeys();
if (cursors.left.isDown) {
player.setVelocityX(-160);
player.anims.play('left', true);
} else if (cursors.right.isDown) {
player.setVelocityX(160);
player.anims.play('right', true);
} else {
player.setVelocityX(0);
player.anims.play('turn');
}
if (cursors.up.isDown && player.body.touching.down) {
player.setVelocityY(-330);
}
}
- Add Assets: You need
some images and sprites for this game. Download or create a background image (sky.png
), a platform image (platform.png
), a star image (star.png
), and a sprite sheet for the character (dude.png
). Place them in an assets
directory in your project.
Step 4: Testing and Running Your Game
- Open your local server link in your browser and see your game in action.
- Use the arrow keys to move the character around.
Taking it further
Building a simple game with Phaser 3 involves setting up your environment, coding game logic, and running the game on a server. By following this tutorial, you've learned the basics of game development with Phaser 3, including scene management, loading assets, adding physics, and creating animations. As you become more comfortable with Phaser, you can explore more complex game mechanics, incorporate sound, and even integrate networked multiplayer features. Enjoy the process of learning and game development!
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
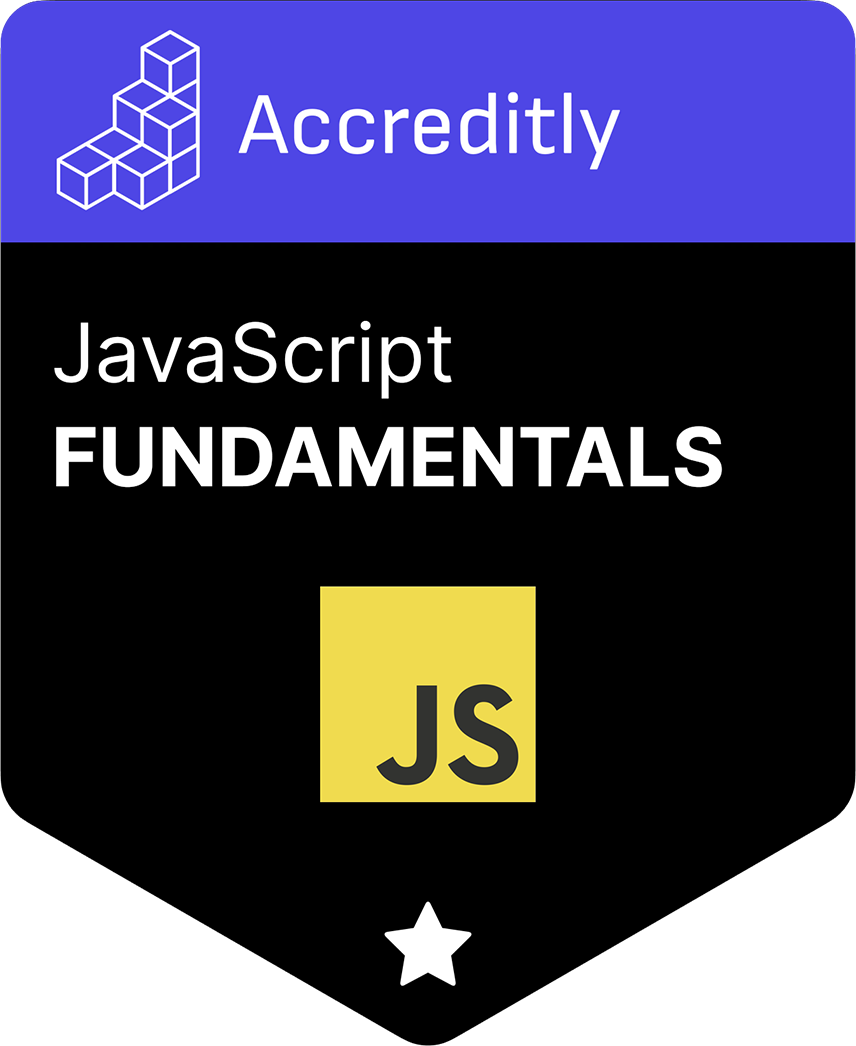