- What is JSON?
- Parsing JSON with JSON.parse()
- Stringifying with JSON.stringify()
- Things to Consider
- Using JSON in Real-world Scenarios
- JSON ftw
JavaScript Object Notation (JSON) has become the de facto standard for data interchange on the web. Its simplicity and ease of use make it an ideal choice for sending data between a server and client. In JavaScript, handling JSON data is straightforward, thanks to built-in methods that facilitate the encoding and decoding processes. Let's explore these methods and their applications.
What is JSON?
JSON is a lightweight data-interchange format that's easy for humans to read and write, and easy for machines to parse and generate. It closely resembles JavaScript's object literal notation, with a few differences (e.g., all keys must be in double quotes).
Example JSON data:
{
"name": "John",
"age": 30,
"isStudent": false,
"subjects": ["Math", "Science"]
}
JSON.parse()
Parsing JSON with The JSON.parse()
method takes a JSON string and transforms it into a JavaScript object or value.
const jsonString = '{"name":"John", "age":30, "isStudent":false}';
const jsObject = JSON.parse(jsonString);
console.log(jsObject.name); // Outputs: John
This method can also accept an optional reviver
function to further process the resulting object.
JSON.stringify()
Stringifying with The opposite of parsing, JSON.stringify()
converts a JavaScript value or object into a JSON string.
const jsObject = {
name: "John",
age: 30,
isStudent: false
};
const jsonString = JSON.stringify(jsObject);
console.log(jsonString); // Outputs: {"name":"John","age":30,"isStudent":false}
JSON.stringify()
can also take additional optional parameters like a replacer function or a space value for formatting.
Things to Consider
-
Not Everything Can Be Stringified: Some JavaScript types can't be represented in JSON. For example, functions and
undefined
values are left out during stringification. -
Parse Errors: Invalid JSON will throw an error during parsing. Always ensure you're dealing with valid JSON before attempting to parse it.
-
Depth and Recursion: The
JSON.stringify()
method has a default depth of 10. If your object is deeply nested, you may encounter issues or lose data in the output. -
Data Security: When parsing data from an unknown source, always ensure it's from a trusted origin. Malicious data can result in security vulnerabilities.
Using JSON in Real-world Scenarios
JSON's primary use-case in JavaScript is for AJAX (Asynchronous JavaScript and XML) communications. When fetching data from a server or sending data to one, it's common to receive or transmit data in JSON format.
For instance, using the Fetch API:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log('Error:', error));
In the example above, the response.json()
method internally uses JSON.parse()
to transform the JSON response into a JavaScript object.
JSON ftw
JSON's integration into JavaScript provides developers with powerful tools for data interchange. With just two primary methods, JSON.parse()
and JSON.stringify()
, handling JSON data becomes a breeze. As web applications continue to grow and rely heavily on data transfer, understanding and effectively using JSON in JavaScript remains an essential skill for modern web development.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
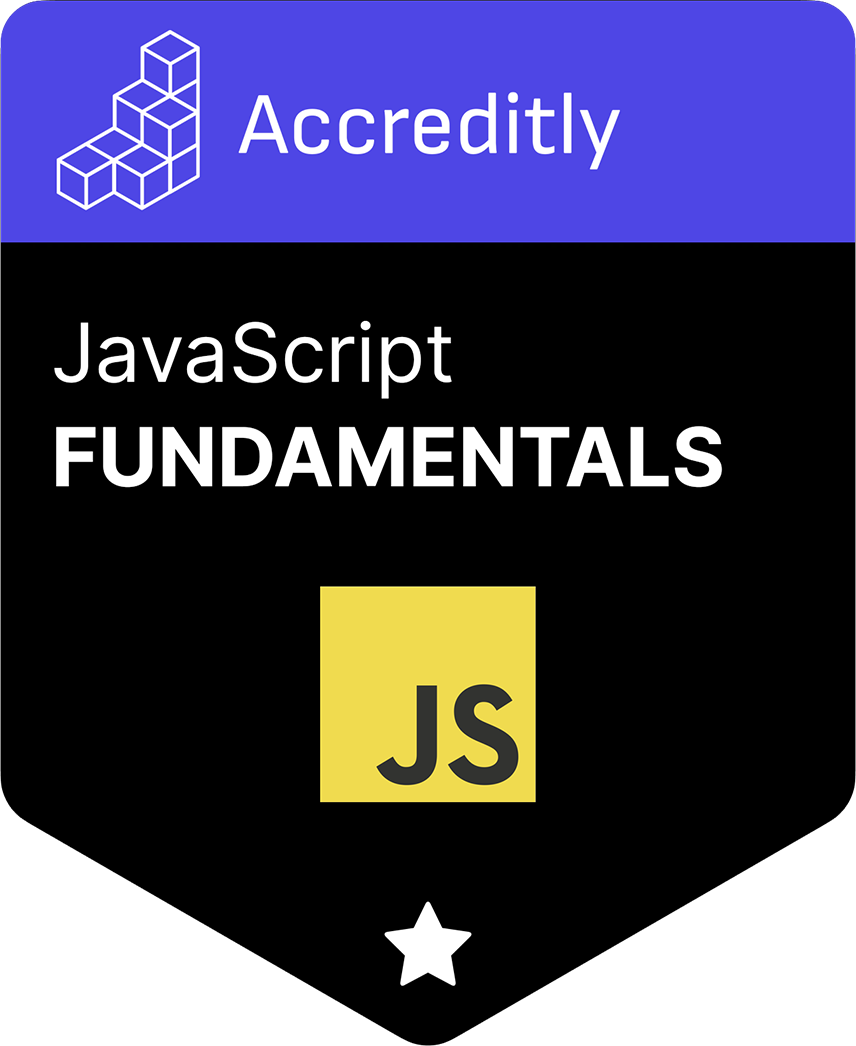