- Understanding wp_enqueue_script() and wp_enqueue_style()
- Including CSS Files
- Including JavaScript Files
- Why Not Just Include in Header/Footer?
- Managing Dependencies
- Versioning and Cache Busting
- Conditional Loading
Incorporating CSS and JavaScript into your WordPress theme isn't just about dropping links into your template files. WordPress provides a structured way to include these resources, ensuring they are loaded efficiently and without conflicts. Understanding the correct method to include CSS and JavaScript is crucial for any theme developer. Let's explore the best practices for doing this the right way.
Before we jump in, remember that one of the key benefits to all of this is to allow effective cache busting, so be sure to check out our article on how to implement effective cache busting in WordPress.
wp_enqueue_script()
and wp_enqueue_style()
Understanding The functions wp_enqueue_script()
and wp_enqueue_style()
are the heart of script and style management in WordPress. They help you add CSS and JavaScript files while managing dependencies, versions, and in-footer/in-header placements.
Including CSS Files
To include a CSS file, use wp_enqueue_style()
:
function my_theme_enqueue_styles() {
wp_enqueue_style('bootstrap-css', 'https://example.com/bootstrap.css', array(), '4.1.0');
wp_enqueue_style('my-theme-style', get_stylesheet_uri(), array('bootstrap-css'), '1.0.0');
}
add_action('wp_enqueue_scripts', 'my_theme_enqueue_styles');
In this example, we’re loading a Bootstrap stylesheet from an external source and then our theme’s stylesheet, specifying Bootstrap as a dependency. This ensures that Bootstrap’s CSS loads first.
Including JavaScript Files
For JavaScript files, use wp_enqueue_script()
:
function my_theme_enqueue_scripts() {
wp_enqueue_script('jquery');
wp_enqueue_script('bootstrap-js', 'https://example.com/bootstrap.js', array('jquery'), '4.1.0', true);
wp_enqueue_script('my-theme-script', get_template_directory_uri() . '/js/custom.js', array('jquery', 'bootstrap-js'), '1.0.0', true);
}
add_action('wp_enqueue_scripts', 'my_theme_enqueue_scripts');
Here, we first enqueue jQuery (included with WordPress), then Bootstrap’s JS, and finally our custom script. The last parameter true
specifies that the script should be loaded in the footer.
Why Not Just Include in Header/Footer?
While directly including scripts and styles in the header or footer might seem straightforward, this approach doesn't manage dependencies and can lead to conflicts or duplications. Furthermore, it doesn’t handle different environments or conditionally loading scripts.
Managing Dependencies
One of the primary benefits of using the enqueue system is dependency management. WordPress intelligently loads scripts and styles only once, even if they are enqueued multiple times across various plugins and themes.
Versioning and Cache Busting
Another advantage is version control. By specifying a version number in your wp_enqueue_script
or wp_enqueue_style
function, WordPress can help with cache busting. When you update the version number, WordPress ensures users receive the latest version of the file.
Conditional Loading
Sometimes you only want to load a script or style on a specific page or under certain conditions. WordPress's conditional tags (like is_home()
, is_single()
, etc.) can be used within your enqueue functions to load resources only where needed.
Properly enqueuing scripts and styles in WordPress themes is crucial for a well-functioning website. It ensures that your CSS and JavaScript files are loaded in the right order, without conflicts, and optimizes your site’s performance and user experience.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
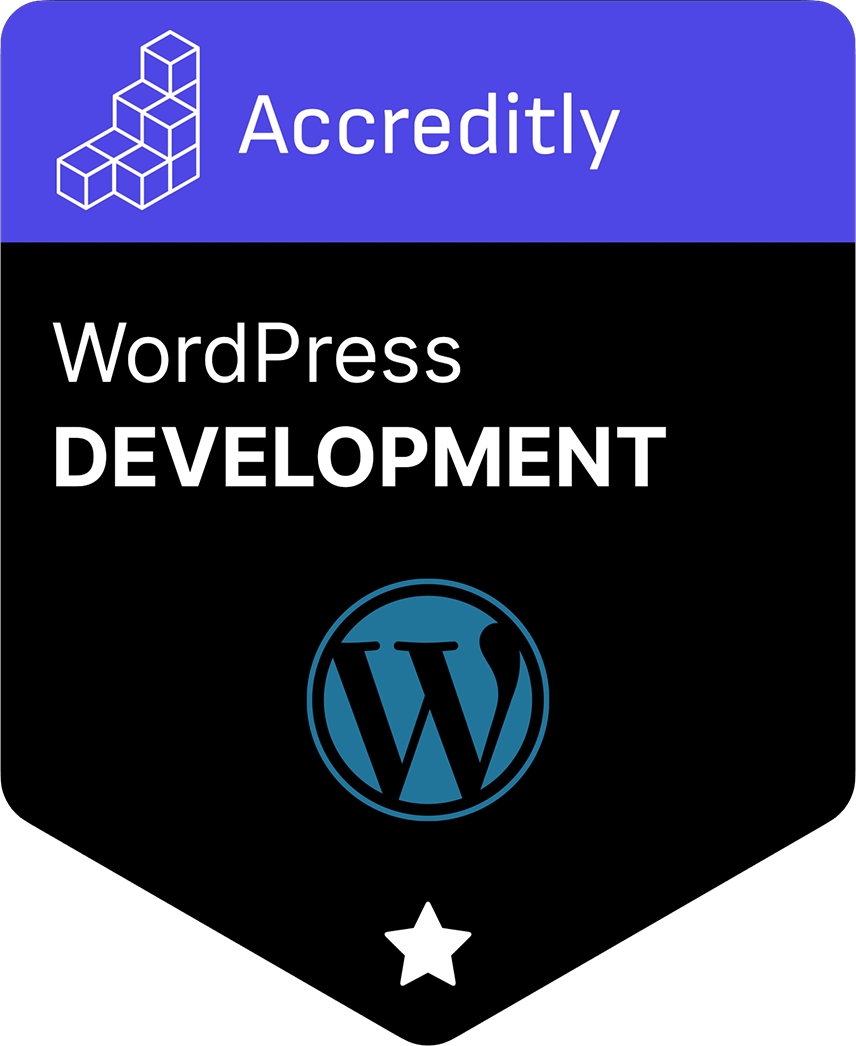