Caching is an essential performance optimization strategy that stores the static elements of a webpage, reducing the load on servers and providing a faster experience for users. However, caching can sometimes cause problems when you've updated your stylesheets or JavaScript files, and the changes are not immediately visible because the browser is loading the old, cached versions. This is where cache busting comes in handy.
Cache busting is a strategy used to prevent a browser from reusing cached resources. It works by appending a version number or timestamp to the URLs of static files, so the browser treats them as new resources and fetches the latest versions from the server.
For example:
<link rel="stylesheet" type="text/css" href="styles.css?100" />
Is seen as a different file to:
<link rel="stylesheet" type="text/css" href="styles.css?200" />
(note the ?100
and ?200
on the file path)
This article will guide you through how to implement cache busting for stylesheets and JavaScript files in WordPress. Let's get started.
Adding Version Numbers to Enqueued Files
WordPress provides built-in functions to enqueue styles and scripts, which are wp_enqueue_style
and wp_enqueue_script.
These functions have a parameter for the version number which can be used for cache busting.
Here's an example of how to enqueue a stylesheet with version number:
function my_theme_styles() {
wp_enqueue_style('my-theme-styles', get_stylesheet_directory_uri() . '/style.css', array(), '1.0.0');
}
add_action('wp_enqueue_scripts', 'my_theme_styles');
In this example, '1.0.0' is the version number of the stylesheet. Every time you modify the stylesheet, you can increment the version number, and the browser will fetch the new version.
Using File Modification Time as the Version Number
An even better approach is to use the file's modification time as the version number. This way, every time the file changes, the version number will be automatically updated, and you won't have to remember to manually change it.
Here's how to implement this method:
function my_theme_styles() {
wp_enqueue_style(
'my-theme-styles',
get_stylesheet_directory_uri() . '/style.css',
array(),
filemtime(get_stylesheet_directory() . '/style.css')
);
}
add_action('wp_enqueue_scripts', 'my_theme_styles');
In this example, the filemtime
function is used to get the modification time of the stylesheet file, and it's used as the version number.
The same method can be applied for JavaScript files:
function my_theme_scripts() {
wp_enqueue_script(
'my-theme-scripts',
get_template_directory_uri() . '/js/scripts.js',
array('jquery'), // Dependecy
filemtime(get_template_directory() . '/js/scripts.js'),
true
);
}
add_action('wp_enqueue_scripts', 'my_theme_scripts');
In the script enqueue function, the fifth parameter is set to true to load the script in the footer.
Implementing cache busting in WordPress is relatively straightforward and can ensure that your users are always getting the latest updates to your stylesheets and JavaScript files. By taking advantage of the built-in enqueue functions and PHP's file modification time function, you can automate cache busting and make your development workflow smoother and more efficient.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
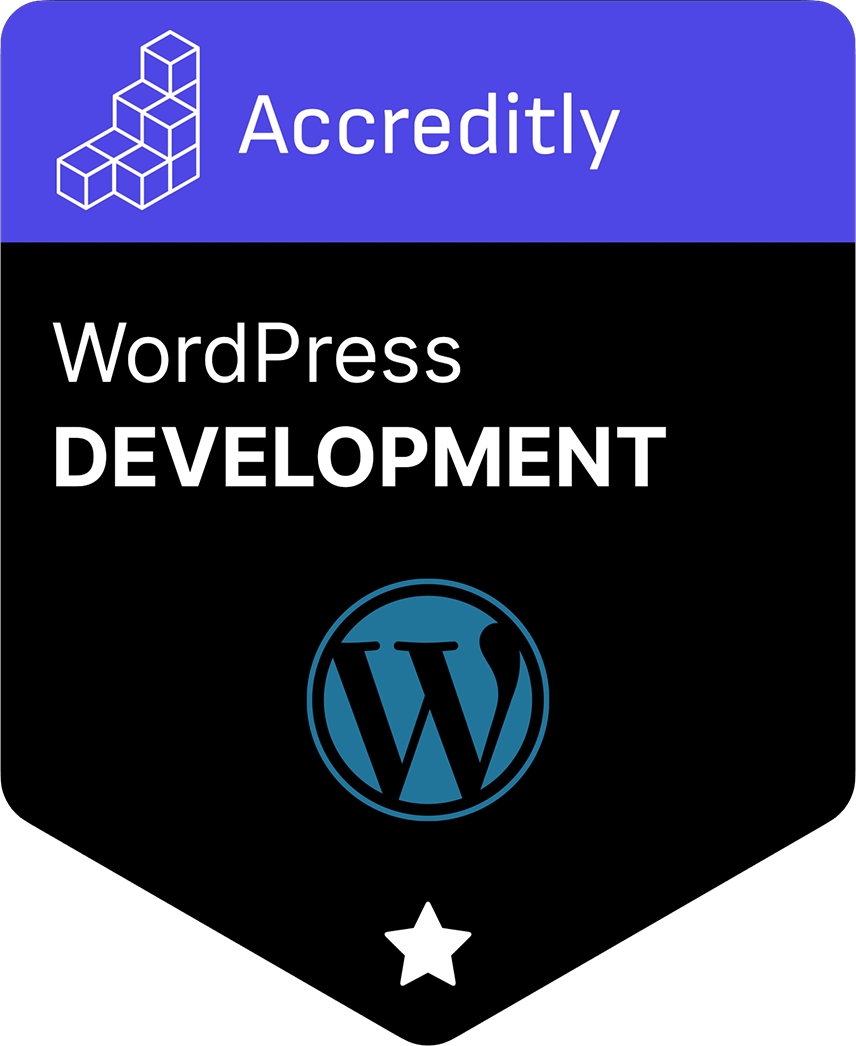