- Creating a Stored Procedure
- Calling a Stored Procedure
- Modifying a Stored Procedure
- Parameters in Stored Procedures
Stored procedures are a powerful feature of MySQL. They are SQL statements that are stored in the database server. Once created, they can be called or referred to as needed. This can significantly streamline operations by reducing network traffic, enhancing the versatility of triggers, and making database applications more robust. This tutorial will guide you through the process of creating, using, and managing stored procedures in MySQL.
Creating a Stored Procedure
Here's how to create a basic stored procedure. In this example, we'll make a procedure that selects all records from a hypothetical users
table.
DELIMITER $$
CREATE PROCEDURE `SelectAllUsers`()
BEGIN
SELECT * FROM users;
END $$
DELIMITER ;
In the above SQL script:
-
DELIMITER $$
changes the standard delimiter (semicolon) in MySQL to$$
. This is necessary because the semicolon is used within the stored procedure, which could cause confusion. We revert to the semicolon as a delimiter after the procedure is created. -
CREATE PROCEDURE
defines the start of the procedure.SelectAllUsers
is the procedure's name. -
BEGIN
andEND
enclose the SQL queries that make up the stored procedure. In this case, there's just one:SELECT * FROM users
.
Calling a Stored Procedure
You can call a stored procedure using the CALL statement. To call the SelectAllUsers
procedure, you would use:
CALL SelectAllUsers();
Modifying a Stored Procedure
To modify a stored procedure, you must drop and recreate it. To drop a procedure, use the DROP PROCEDURE
statement:
DROP PROCEDURE IF EXISTS SelectAllUsers;
Once the procedure has been dropped, you can recreate it with the new modifications.
Parameters in Stored Procedures
Stored procedures can accept parameters. Let's create a stored procedure with an IN
parameter. In this case, our procedure will retrieve users from the users table with a specific role.
DELIMITER $$
CREATE PROCEDURE `GetUsersByRole`(IN role varchar(255))
BEGIN
SELECT * FROM users WHERE role = role;
END $$
DELIMITER ;
To call this stored procedure and retrieve all users with the role "admin", you'd use:
CALL GetUsersByRole('admin');
Stored procedures can simplify complex operations, making your database interactions more efficient. By understanding how to create, use, and manage stored procedures in MySQL, you can unlock a powerful tool for your database operations. Always remember to think carefully about your needs when creating stored procedures - although they are powerful, they can be harder to debug than standard SQL queries and may not always be the best tool for the job.
Interested in proving your knowledge of this topic? Take the Database Fundamentals certification.
Database Fundamentals
Focusing on SQL and database design, this exam will test your knowledge of database fundamentals.
$99
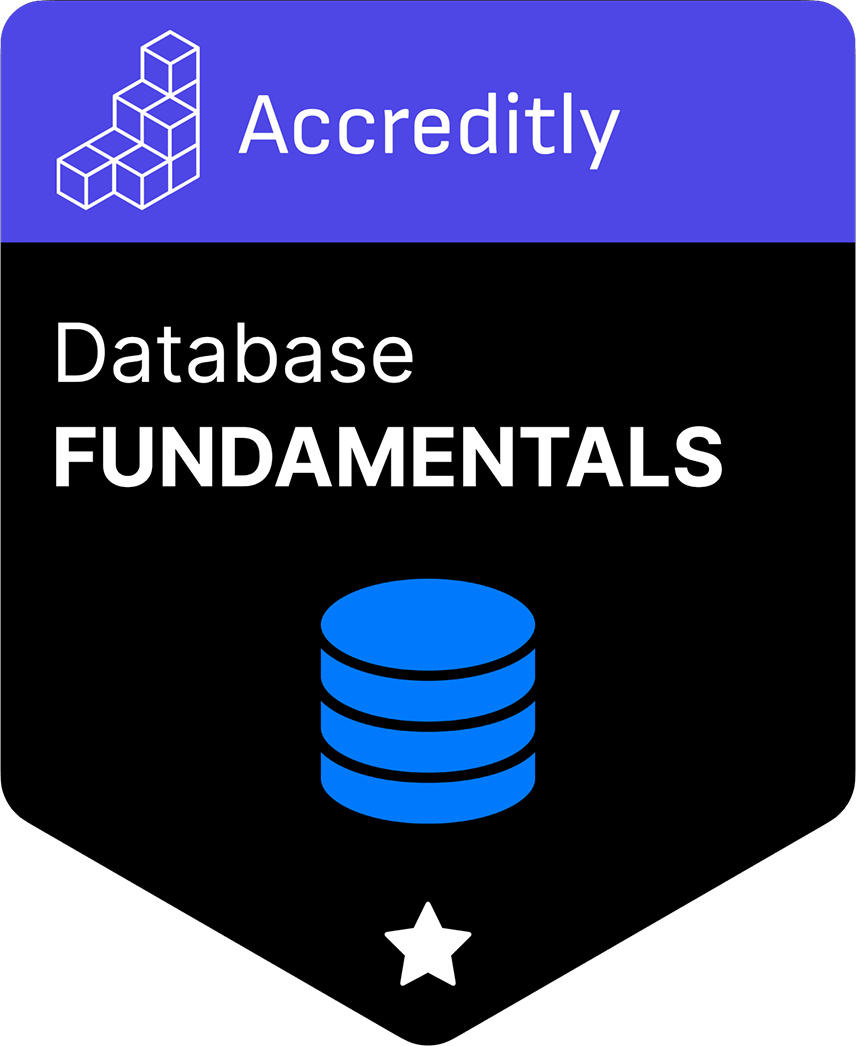