- What Are WordPress Transients?
- Why Use Transients?
- Creating a Transient in WordPress
- Retrieving a Transient
- Deleting a Transient
- Best Practices for Using Transients
In the realm of WordPress development, transients offer a powerful way to store and retrieve temporary data, thereby enhancing your website's performance. Transients are essentially a caching mechanism that temporarily stores data with an expiration time. This feature is particularly useful for storing data that is expensive to generate, like API call results, complex query results, or dynamically generated content.
What Are WordPress Transients?
WordPress transients provide a straightforward API for storing cached data in the database temporarily. Unlike options, transients have an expiration time, after which they expire and are deleted from the database. This makes them ideal for temporary data that doesn't need to persist for long periods.
Why Use Transients?
Transients can significantly reduce server load and page loading times. By caching data that would otherwise require time-consuming operations to generate, you can deliver content faster to your users.
Creating a Transient in WordPress
Creating a transient involves using the set_transient()
function. This function requires three parameters:
1. The Transient Name: A unique name for your transient.
2. The Transient Value: The data you want to store.
3. Expiration Time: How long the data should be stored (in seconds).
Example:
$weather_data = [
'temperature' => '75°F',
'condition' => 'Sunny'
];
set_transient('weather_data', $weather_data, 12 * HOUR_IN_SECONDS);
In this example, weather data is stored for 12 hours. After that, the transient expires and is removed from the database.
Retrieving a Transient
To retrieve the data stored in a transient, use the get_transient()
function:
$weather_data = get_transient('weather_data');
if (false !== $weather_data) {
// The transient exists and is valid
echo 'Weather: ' . $weather_data['condition'];
} else {
// The transient does not exist or has expired
}
This code fetches the weather data if it hasn't expired yet.
Deleting a Transient
To delete a transient before it expires, use the delete_transient()
function:
delete_transient('weather_data');
Best Practices for Using Transients
1. Unique Names: Ensure transient names are unique to avoid conflicts.
2. Expiration Times: Choose appropriate expiration times based on how often the data changes.
3. Error Handling: Always check if the transient retrieval was successful.
4. Not a Replacement for Permanent Storage: Transients should not be used for permanent data storage.
Transients in WordPress are a powerful tool for optimizing performance by caching temporary data. They can help reduce database load and speed up your site, especially if you're frequently handling complex operations. By mastering the use of transients, WordPress developers can significantly enhance the efficiency and speed of their websites.
Remember, while transients are a handy feature, they should be used judiciously and for the right purposes. Leveraging transients effectively can be a key part of a strategy to optimize your WordPress site for better performance and a more seamless user experience.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
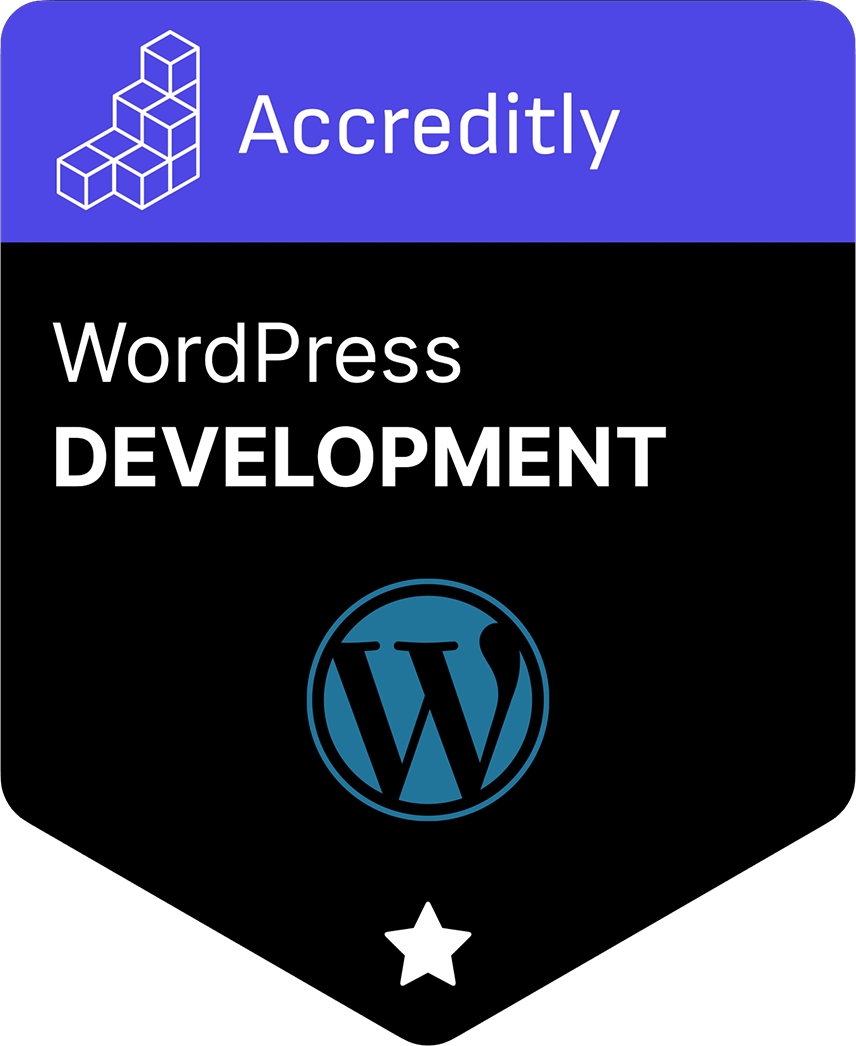