- Step 1: Obtain API Access
- Step 2: Create a PHP Script to Fetch Pins
- Step 3: Display Pins on Your Website
- Step 4: Style Your Pins
- Step 5: Use the Shortcode
- Step 6: Clear and Manage Cache (Optional)
- Wrapping up
Integrating Pinterest content into your WordPress website can enhance your site's visual appeal and engagement by showcasing your latest pins. While several plugins can achieve this, using the Pinterest API directly offers more control and reduces dependencies. This tutorial will guide you through the process of fetching and displaying Pinterest pins on your WordPress website without using a plugin.
Step 1: Obtain API Access
Before you can retrieve pins from Pinterest, you need access to the Pinterest API. Here’s how to get started:
-
Create a Pinterest Developer Account:
- Visit Pinterest for Developers and log in with your Pinterest account.
- Navigate to the Apps section and create a new app to get your API credentials (API key).
-
Obtain an Access Token:
- After creating your app, you will need an access token to make authenticated requests to the Pinterest API. Follow the authentication steps provided in the Pinterest API documentation to get your token.
Step 2: Create a PHP Script to Fetch Pins
You will use PHP to interact with the Pinterest API. Create a PHP script that fetches your Pinterest pins and can be included in your WordPress theme.
-
Create a PHP Function to Fetch Pins:
- Add the following function to your theme’s
functions.php
file. Replace'your-access-token'
and'your-board-id'
with your actual access token and the ID of the Pinterest board from which you want to fetch pins.
- Add the following function to your theme’s
function fetch_pinterest_pins() {
$access_token = 'your-access-token';
$board_id = 'your-board-id';
$url = "https://api.pinterest.com/v1/boards/{$board_id}/pins/?access_token={$access_token}&fields=id,link,creator(image,url),image,note,created_at,counts";
$response = wp_remote_get($url);
if (is_wp_error($response)) {
return []; // Handle error appropriately
}
$body = wp_remote_retrieve_body($response);
$data = json_decode($body);
if (isset($data->data)) {
return $data->data;
}
return [];
}
This function uses WordPress’s HTTP API to make a GET request to the Pinterest API and fetches pins from a specific board.
Step 3: Display Pins on Your Website
Now that you have a function to fetch the pins, you can use it to display these pins within your WordPress templates.
-
Create a Shortcode to Display Pins:
- Add the following code to your
functions.php
to create a shortcode[pinterest_pins]
that you can use anywhere on your site to display the pins.
- Add the following code to your
function display_pinterest_pins() {
$pins = fetch_pinterest_pins();
$output = '<div class="pinterest-pins">';
foreach ($pins as $pin) {
$output .= '<div class="pin">';
$output .= '<a href="' . esc_url($pin->link) . '" target="_blank">';
$output .= '<img src="' . esc_url($pin->image->original->url) . '" alt="' . esc_attr($pin->note) . '">';
$output .= '</a>';
$output .= '</div>';
}
$output .= '</div>';
return $output;
}
add_shortcode('pinterest_pins', 'display_pinterest_pins');
This shortcode loops through the fetched pins and creates an HTML structure to display them. Each pin is wrapped in an
anchor tag linking back to the original pin on Pinterest, and the images are displayed using the <img>
tag.
Step 4: Style Your Pins
To ensure the pins look good on your site, add some basic CSS to your theme's stylesheet. You can customize this CSS to match your site's design.
.pinterest-pins {
display: flex;
flex-wrap: wrap;
justify-content: center;
gap: 15px;
}
.pinterest-pins .pin {
width: 200px;
border: 1px solid #ccc;
padding: 10px;
box-shadow: 0 2px 5px rgba(0,0,0,0.1);
}
.pinterest-pins .pin img {
width: 100%;
height: auto;
display: block;
}
This CSS will arrange the pins in a flexible grid layout, ensuring they are spaced evenly and are visually appealing.
Step 5: Use the Shortcode
With the shortcode [pinterest_pins]
now available, you can add it to any post, page, or widget area where shortcodes are supported. Simply insert the shortcode where you want the pins to appear:
[pinterest_pins]
Alternatively, if you want to directly insert it within template files, use the do_shortcode
function:
echo do_shortcode('[pinterest_pins]');
Step 6: Clear and Manage Cache (Optional)
If your WordPress site uses a caching plugin or service, remember to clear the cache after updating the functions.php
file or the stylesheet. This ensures that the latest changes are visible to your visitors.
Additionally, you might want to cache the results of the Pinterest API calls to reduce the load on your server and speed up page loading times. You can implement a simple caching mechanism using WordPress transients:
function fetch_pinterest_pins() {
$cached_pins = get_transient('cached_pinterest_pins');
if ($cached_pins) {
return $cached_pins;
}
// [Insert the rest of the fetch_pinterest_pins function code here]
// Save the fetched pins in a transient for 12 hours
set_transient('cached_pinterest_pins', $data->data, 12 * HOUR_IN_SECONDS);
return $data->data;
}
This modification adds caching to your pin fetching function, storing the results for 12 hours before needing to refetch them from Pinterest.
Wrapping up
By integrating Pinterest pins directly into your WordPress site without a plugin, you maintain greater control over the functionality and presentation of your content. This approach not only enhances the visual appeal of your site but also leverages social proof by showcasing your Pinterest activity. Remember to comply with Pinterest's API usage terms and manage your API rate limits wisely to ensure your integration remains effective and sustainable.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
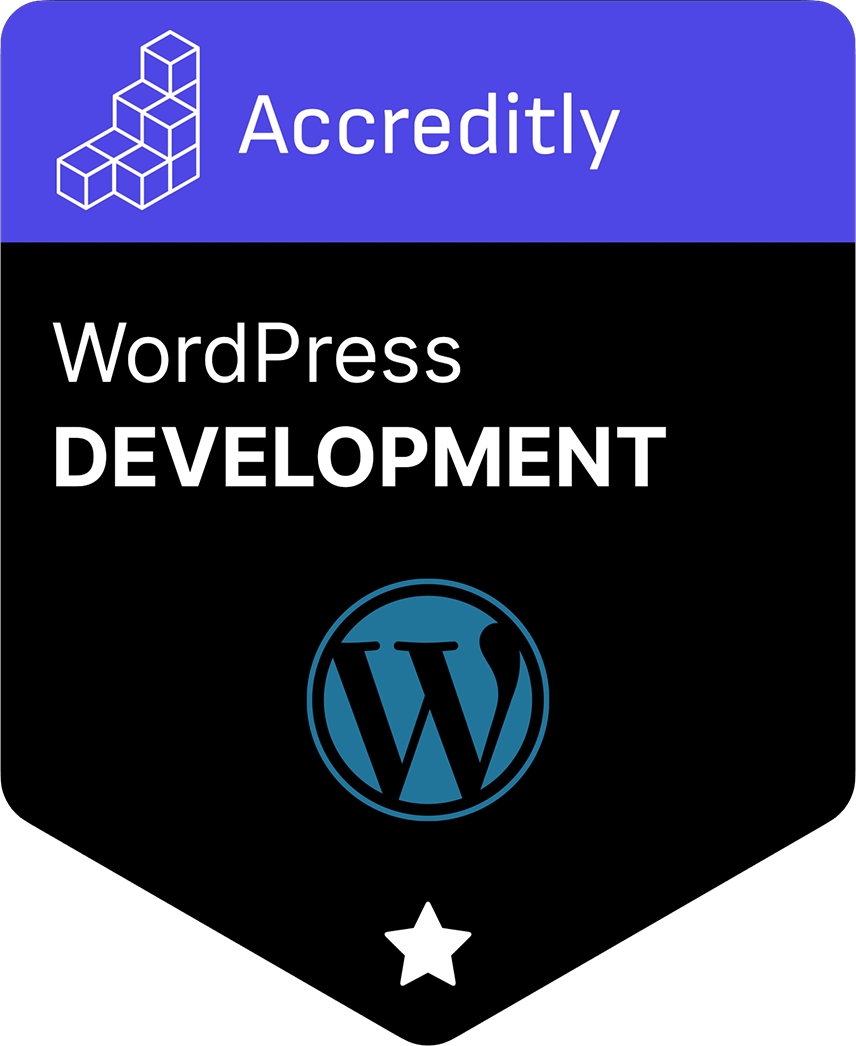