- Step 1: Create a Custom Post Type
- Step 2: Create Custom Fields
- Step 3: Create the ACF Form
- Step 4: Enable Editing
Allowing users to manage their own versions of custom post types on the frontend of a WordPress site can improve user experience and streamline content management. In this tutorial, we'll use Advanced Custom Fields (ACF) forms to enable this feature.
ACF provides a simple way to create frontend forms, perfect for managing custom post types. We'll set up a simple book review site where users can add and edit their own book reviews.
Before we start, ensure you have the ACF Pro plugin installed and activated, as frontend forms are a pro feature.
Step 1: Create a Custom Post Type
First, we'll need to create a custom post type for our book reviews. You can do this either with a plugin like Custom Post Type UI, or by adding code to your theme's functions.php
file. Remember to keep your functions.php file organized.
Here is a simple example of how you could define this post type using code:
function create_book_review_cpt() {
$args = array(
'public' => true,
'label' => 'Book Reviews',
'supports' => array('title', 'editor', 'custom-fields'),
);
register_post_type('book_reviews', $args);
}
add_action('init', 'create_book_review_cpt');
This code will create a new post type labeled 'Book Reviews'. Now, you'll be able to add new book reviews from your WordPress dashboard.
Step 2: Create Custom Fields
Next, let's create some custom fields for our book reviews. We'll add fields for the book title, author, and review text.
In your WordPress dashboard, go to Custom Fields > Add New. Add your new fields and assign them to the 'Book Reviews' post type.
Step 3: Create the ACF Form
Now, we're ready to create the frontend form using ACF. First, we need to add a new page where our form will live. Go to Pages > Add New and create a new page.
Next, let's create the form. We can use the ACF function acf_form()
to display our form on the frontend.
Add the following code to your page template:
<?php
acf_form_head();
get_header();
?>
<div id="primary" class="content-area">
<main id="main" class="site-main" role="main">
<?php
acf_form(array(
'post_id' => 'new_post',
'new_post' => array(
'post_type' => 'book_reviews',
'post_status' => 'publish'
),
'submit_value' => 'Submit a book review'
));
?>
</main><!-- .site-main -->
</div><!-- .content-area -->
<?php get_footer(); ?>
The 'new_post' parameter means that the form will create a new book review when submitted.
Step 4: Enable Editing
To allow users to edit their own book reviews, we need to pass the ID of the post to the ACF form.
Here's an example of how to do this:
<?php
acf_form_head();
get_header();
?>
<div id="primary" class="content-area">
<main id="main" class="site-main" role="main">
<?php
acf_form(array(
'post_id' => get_the_ID(),
'post_title' => true,
'post_content' => true,
'submit_value' => 'Update your book review'
));
?>
</main><!-- .site-main -->
</div><!-- .content-area -->
<?php get_footer(); ?>
This will pre-fill the form with the current post's data, allowing users to edit their reviews.
That's it! You've created a frontend form that allows users to manage their own versions of a custom post type.
This is just the beginning of what you can do with ACF forms. You can add validation, customize form appearance, and much more.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
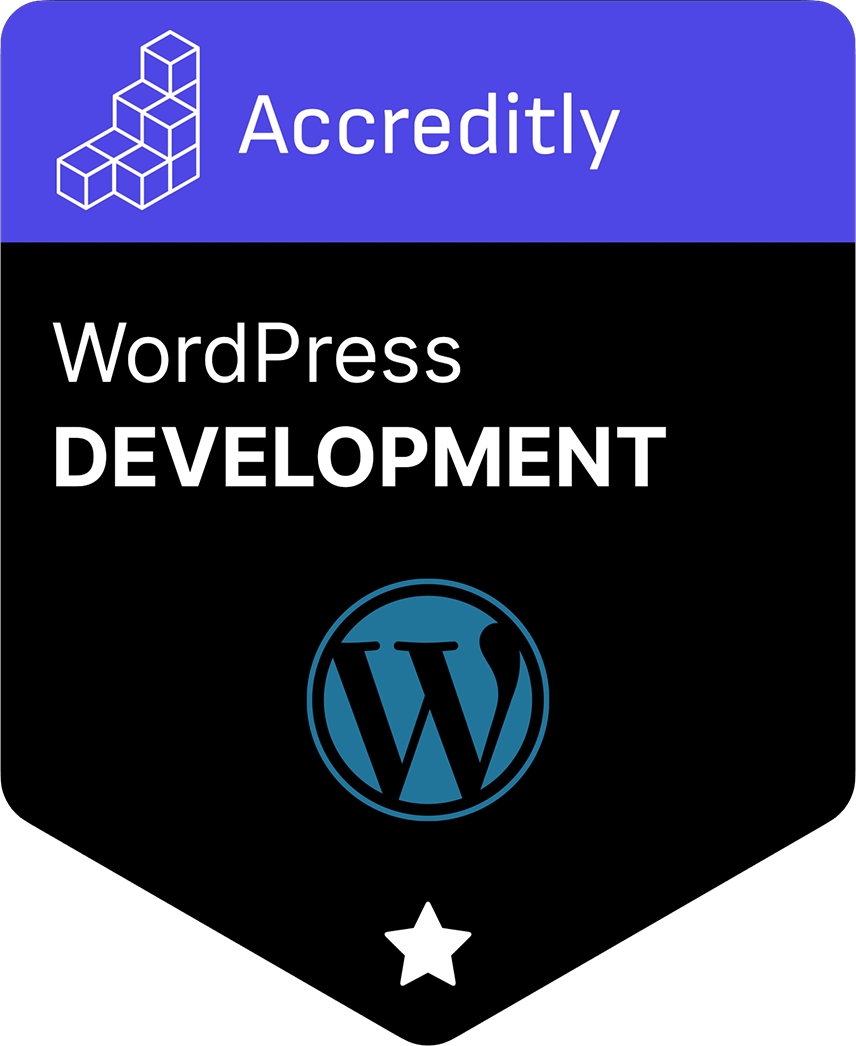