- Bad functions.php file organisation
- functions.php organisation the right way
- Example theme structure
- Example functions.php file
When building a WordPress theme, it's important to maintain a clean and tidy codebase. A well-organized code structure not only improves maintainability but also makes it easier for other developers to understand and contribute to your theme. In this article, we'll explore how to lay out code in a WordPress theme for a clean, organized, and efficient structure.
A WordPress theme typically consists of multiple files, such as header.php
, footer.php
, index.php
, page.php
, single.php
, and functions.php
. However, placing all your code in these files can quickly become overwhelming and challenging to manage.
To keep your code organized and maintainable, it's a good practice to break your code into smaller, manageable pieces and store them in separate files within organized subdirectories. This approach enables you to quickly locate specific code blocks and reduces the complexity of your theme.
functions.php
file organisation
Bad Lets start with the functions.php
file. It's very easy for this file to quickly grow in size and become unmanageable. I have seen production sites with a functions.php
file measuring tens of thousands of lines long, which is completely unworkable.
When a file becomes large like this it introduces a number of problems. The first is obvious; it's a pain to work with. Imagine needing to make a subtle tweak to a navigation walker, but to make that tweak you have to find the line you need to change in a 30,000 line file. That may not be too difficult if you have the name of the function or class that you need to edit but if you're searching for something more generic then you could waste hours.
The other issues are less obvious. You should be using version control like git to manage code changes. If you have multiple developers in your team making changes then they're almost certainly going to be editing the same file. That means every time you go to push code changes you're going to have merge conflicts, and given how complex that file can get it's easy for you to erase or mis-merge code.
So how should you do it?
functions.php
organisation the right way
The best rule to follow when keeping your functions.php
file organised is: No code in functions.php
. Your functions.php
file should just be used for including other files. Nothing more. It's really easy to have a small piece of code you need to add to just drop it in, but that quickly gets out of hand.
Example theme structure
Organise your code that you include into functions.php
into sub-folders. Take the following folder structure as an example:
my-theme/
├── assets/
│ ├── css/
│ ├── js/
│ └── images/
├── inc/
│ ├── custom-code/
│ ├── custom-post-types/
│ ├── custom-shortcodes/
│ ├── custom-taxonomies/
│ ├── custom-widgets/
│ ├── theme-setup.php
│ └── theme-scripts.php
├── templates/
│ ├── header/
│ ├── footer/
│ └── parts/
├── footer.php
├── functions.php
├── header.php
├── index.php
├── page.php
├── single.php
└── style.css
Everything within the inc
directory are files and folders that you include in your functions.php
file.
functions.php
file
Example Lets take a look at a sample functions.php
file that would be used in this folder structure.
<?php
/**
* functions.php
*
* @author Accreditly <[email protected]>
*/
// Setup
include('inc/theme-setup.php');
include('inc/theme-scripts.php');
// CPT
include('inc/custom-post-types/pages.php');
include('inc/custom-post-types/reviews.php');
include('inc/custom-post-types/products.php');
// Shortcodes
include('inc/custom-shortcodes/contact-form.php');
include('inc/custom-shortcodes/newsletter.php');
// Taxonomies
include('inc/custom-taxonomies/categories.php');
include('inc/custom-taxonomies/review-categories.php');
include('inc/custom-taxonomies/tags.php');
// Widgets
include('inc/custom-widgets/latest-posts.php');
include('inc/custom-widgets/latest-reviews.php');
include('inc/custom-widgets/latest-products.php');
// Custom code
include('inc/custom-code/nav-walker.php');
As you can see, there's no actual code in this file, but rather a signpost for other blocks of code.
It's extremely easy to manage, and each file you include is probably less than 50 lines long. Even in this extremely simple example, if you didn't split things out then you would probably be looking at a functions.php
file that is around 700+ lines long.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
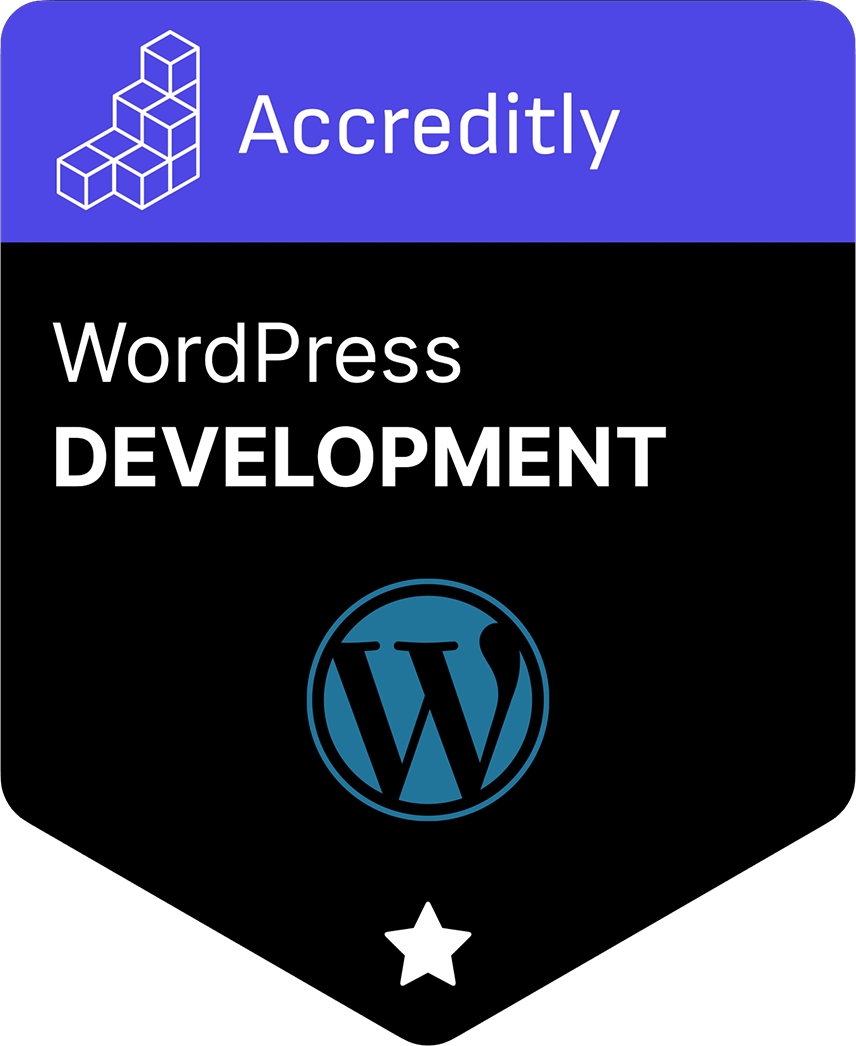