- Why Combine and Minify with Vite?
- Prerequisites
- Step 1: Initialize Your Project
- Step 2: Install Dependencies
- Step 3: Configure Vite for SCSS and JavaScript
- Step 4: Organize Your SCSS and JavaScript Files
- Step 5: Link Your Files in the HTML Template
- Step 6: Running the Development Server
- Step 7: Building for Production
Vite, a modern front-end build tool, is acclaimed for its fast and efficient handling of assets like SCSS and JavaScript. One of Vite's key strengths is its ability to combine and minify these files, which is crucial for optimizing web performance. Let's walk through setting up Vite in your project to combine and minify SCSS and JavaScript files.
Why Combine and Minify with Vite?
Combining and minifying files reduces the number of HTTP requests and the size of files, respectively. This leads to faster loading times and improved website performance, both critical for user experience and SEO.
Prerequisites
- Node.js installed on your system.
- Basic knowledge of SCSS/Sass and JavaScript.
Step 1: Initialize Your Project
Start by creating a new project directory and initializing it:
mkdir my-vite-project
cd my-vite-project
npm init @vitejs/app
Follow the prompts to set up your project. Choose a project name and select a template (e.g., Vanilla, Vue, React).
Step 2: Install Dependencies
Navigate to your project directory and install the necessary dependencies:
npm install
For SCSS, you may need to install additional packages:
npm install -D sass
Step 3: Configure Vite for SCSS and JavaScript
Vite can handle SCSS out of the box. To configure SCSS file handling, modify the vite.config.js
file:
// vite.config.js
import { defineConfig } from 'vite';
export default defineConfig({
css: {
preprocessorOptions: {
scss: {
additionalData: `$injectedColor: orange;`
}
}
}
});
This configuration allows you to set global SCSS variables and options.
Step 4: Organize Your SCSS and JavaScript Files
Create a src
directory in your project root. Inside src
, organize your SCSS and JavaScript files. For example:
-
src/main.js
: Your entry JavaScript file. -
src/styles.scss
: Your main SCSS file.
Step 5: Link Your Files in the HTML Template
In your index.html
(or equivalent), link your main JavaScript file which will import the SCSS file:
<script type="module" src="/src/main.js"></script>
In main.js
, import your SCSS file:
import './styles.scss';
// Your JavaScript code
Step 6: Running the Development Server
Run the Vite development server:
npm run dev
Vite will automatically compile and serve your SCSS and JavaScript files.
Step 7: Building for Production
To combine and minify your SCSS and JavaScript files for production, run:
npm run build
Vite processes your files, combining and minifying them into efficient, production-ready assets in the dist
directory.
Setting up Vite in your project to handle SCSS and JavaScript files not only streamlines your development process but also significantly enhances the performance of your final product. With Vite's fast build times and efficient asset handling, you can focus more on development and less on the intricacies of optimization.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
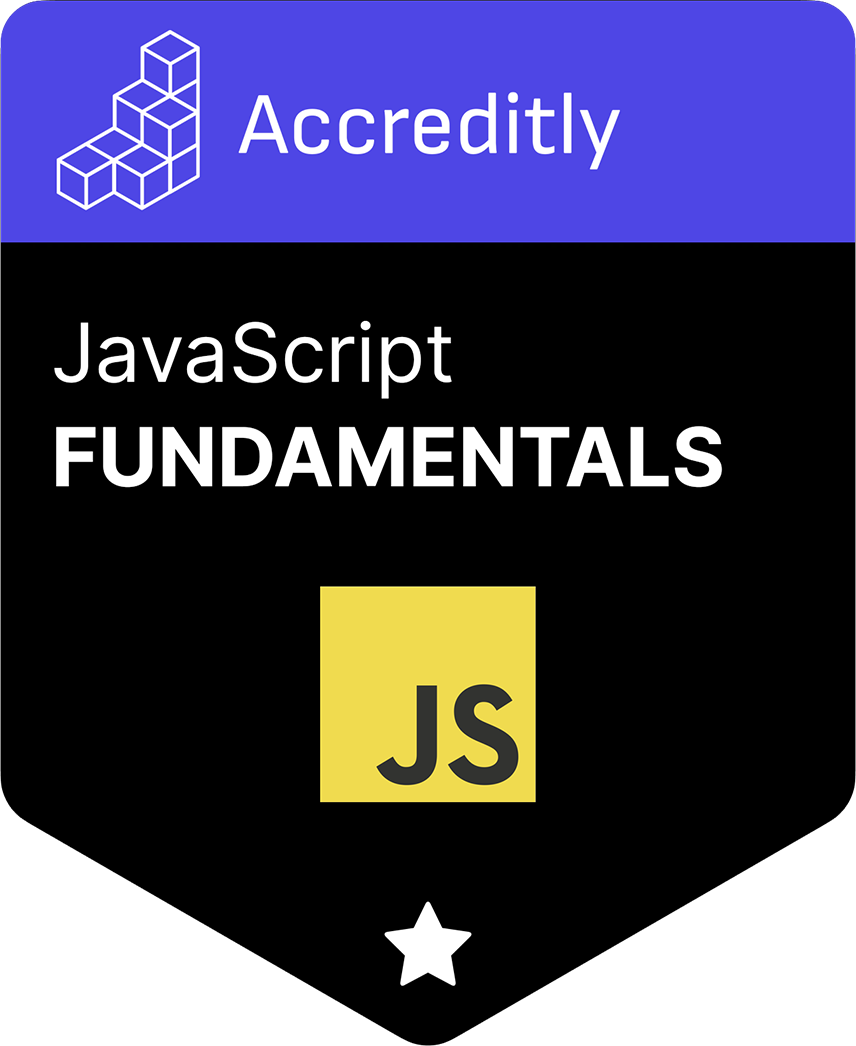
Related articles
Tutorials WordPress Database Design Tooling
How to store and output currency values in WordPress
Effectively manage currency values in WordPress, from storage best practices to displaying currency amounts on your website. This guide covers everything to ensure accurate and user-friendly currency handling in WordPress.