- Why Use QR Codes?
- Setting Up the Project
- Creating the HTML Structure
- Writing the JavaScript Code
- Running the Project
- Enhancing the QR Code Generator
- Summing up
QR codes have become a ubiquitous part of modern technology, used for everything from sharing contact information to facilitating payments. Generating a QR code programmatically can be useful in many web applications. In this tutorial, we'll explore how to generate a QR code in JavaScript using the qrcode
library.
Why Use QR Codes?
Before we dive into the code, let's briefly discuss why QR codes are so popular:
- Ease of Use: QR codes can be scanned quickly with a smartphone, making them user-friendly.
- Versatility: They can store various types of data such as URLs, contact details, Wi-Fi credentials, and more.
- Error Correction: QR codes include error correction, allowing them to be read even if partially damaged or obscured.
Setting Up the Project
To generate QR codes in JavaScript, we'll use the popular qrcode
library. Follow these steps to set up your project:
-
Create a New Project: Create a new directory for your project and navigate into it.
mkdir qr-code-generator cd qr-code-generator
-
Initialize a New Node.js Project: Initialize a new Node.js project with
npm init
.npm init -y
-
Install the
qrcode
Library: Install theqrcode
library using npm.npm install qrcode
-
Create an HTML File: Create an
index.html
file to serve as the frontend for our QR code generator.
Creating the HTML Structure
We'll start by creating a simple HTML structure to hold the QR code and provide an input for the data.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>QR Code Generator</title>
<style>
body {
font-family: Arial, sans-serif;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
#qrcode {
margin-top: 20px;
}
</style>
</head>
<body>
<h1>QR Code Generator</h1>
<input type="text" id="text-input" placeholder="Enter text or URL" />
<button id="generate-btn">Generate QR Code</button>
<canvas id="qrcode"></canvas>
<script src="index.js"></script>
</body>
</html>
Writing the JavaScript Code
Now, let's write the JavaScript code to generate the QR code. Create a file named index.js
in the same directory as your index.html
.
import QRCode from 'qrcode';
// Function to generate QR code
function generateQRCode(text) {
const canvas = document.getElementById('qrcode');
QRCode.toCanvas(canvas, text, (error) => {
if (error) {
console.error('Error generating QR code', error);
} else {
console.log('QR code generated successfully');
}
});
}
// Event listener for button click
document.getElementById('generate-btn').addEventListener('click', () => {
const textInput = document.getElementById('text-input').value;
if (textInput) {
generateQRCode(textInput);
} else {
alert('Please enter some text or URL to generate QR code.');
}
});
Running the Project
To run the project, you need to serve your HTML file using a local server. You can use the http-server
package for this:
-
Install
http-server
Globally: If you don't havehttp-server
installed, you can install it globally.npm install -g http-server
-
Start the Server: Start the server in your project directory.
http-server
-
Open the Browser: Open your browser and navigate to
http://localhost:8080
(or whatever porthttp-server
is using). You should see your QR code generator.
Enhancing the QR Code Generator
While the basic QR code generator works, you might want to enhance it with additional features such as:
- Customization Options: Allow users to customize the QR code's size, color, and error correction level.
- Download Option: Provide a button for users to download the generated QR code as an image file.
- History: Maintain a history of generated QR codes that users can revisit.
Here’s an example of how you can add a download button:
<button id="download-btn">Download QR Code</button>
document.getElementById('download-btn').addEventListener('click', () => {
const canvas = document.getElementById('qrcode');
const url = canvas.toDataURL('image/png');
const link = document.createElement('a');
link.href = url;
link.download = 'qrcode.png';
link.click();
});
Summing up
Generating a QR code in JavaScript is straightforward with the help of the qrcode
library. This tutorial covered setting up a project, creating a simple HTML structure, and writing the JavaScript code to generate and download QR codes. With these basics, you can expand and customize the QR code generator to suit your needs.
For more information and advanced usage, you can refer to the qrcode library documentation.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
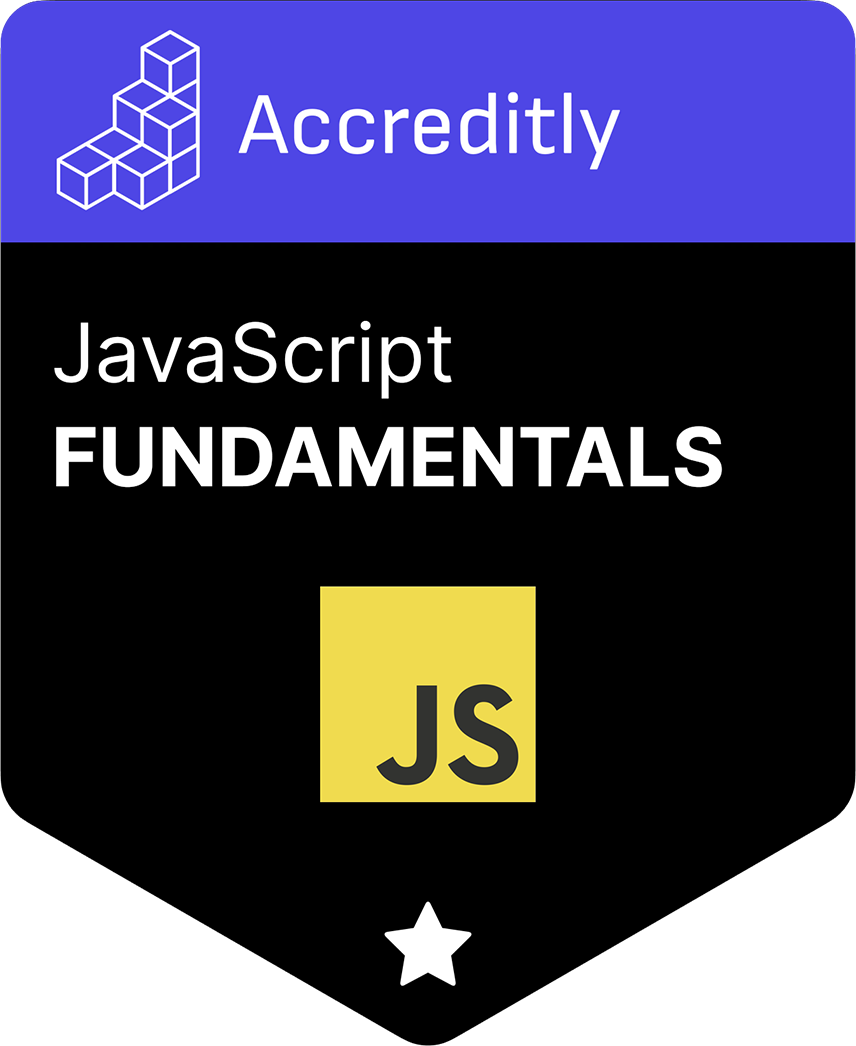