- What is collect.js?
- Installing collect.js
- Getting Started
- Basic Usage
- Common Methods
- Advanced Usage
- Real-World Example
- Conclusion
If you are a Laravel developer transitioning to JavaScript or simply looking for a powerful, fluent API for working with arrays and objects, collect.js
is the library for you. Inspired by Laravel’s Collections, collect.js
brings the ease and elegance of Laravel collections to JavaScript.
In this tutorial, we’ll explore how to use collect.js
to manipulate and interact with arrays and objects in JavaScript, mirroring the collection methods you might be familiar with from Laravel.
collect.js
?
What is collect.js
is a JavaScript library that provides a fluent, convenient wrapper for working with arrays and objects. It mimics the API of Laravel’s Collection class, making it easy to chain multiple operations and work with complex data structures.
collect.js
Installing You can install collect.js
using npm or yarn.
Using npm:
npm install collect.js
Using yarn:
yarn add collect.js
Getting Started
To start using collect.js
, import it into your JavaScript file:
const collect = require('collect.js');
Or, if you are using ES6 modules:
import collect from 'collect.js';
Basic Usage
The basic unit of collect.js
is the Collection. You create a collection by passing an array or an object to the collect
function.
Creating a Collection
const collection = collect([1, 2, 3, 4, 5]);
Retrieving All Items
To get all items in the collection, use the all
method:
console.log(collection.all());
// Output: [1, 2, 3, 4, 5]
Common Methods
Let's explore some of the most commonly used methods in collect.js
.
each
The each
method iterates over the items in the collection and executes a callback for each item.
collection.each((item, index) => {
console.log(`Index: ${index}, Item: ${item}`);
});
map
The map
method iterates over the collection and applies a callback to each item, returning a new collection of the results.
const newCollection = collection.map(item => item * 2);
console.log(newCollection.all());
// Output: [2, 4, 6, 8, 10]
filter
The filter
method filters the collection using a callback and returns a new collection with the items that pass the callback’s test.
const filtered = collection.filter(item => item > 2);
console.log(filtered.all());
// Output: [3, 4, 5]
reduce
The reduce
method reduces the collection to a single value using a callback.
const sum = collection.reduce((carry, item) => carry + item, 0);
console.log(sum);
// Output: 15
pluck
The pluck
method retrieves all values for a given key from an array of objects.
const users = collect([
{ name: 'John', age: 25 },
{ name: 'Jane', age: 30 },
{ name: 'Doe', age: 22 },
]);
const names = users.pluck('name');
console.log(names.all());
// Output: ['John', 'Jane', 'Doe']
sortBy
The sortBy
method sorts the collection by a given key.
const sorted = users.sortBy('age');
console.log(sorted.all());
// Output: [{ name: 'Doe', age: 22 }, { name: 'John', age: 25 }, { name: 'Jane', age: 30 }]
Advanced Usage
Chaining Methods
One of the strengths of collect.js
is the ability to chain multiple methods together for more complex operations.
const result = users
.filter(user => user.age > 23)
.sortBy('age')
.pluck('name');
console.log(result.all());
// Output: ['John', 'Jane']
Working with Objects
You can also work with objects directly using collect.js
.
const user = collect({
name: 'John Doe',
email: '[email protected]',
age: 25
});
console.log(user.get('name'));
// Output: John Doe
const keys = user.keys();
console.log(keys.all());
// Output: ['name', 'email', 'age']
groupBy
The groupBy
method groups the collection by a given key.
const people = collect([
{ name: 'John', age: 25 },
{ name: 'Jane', age: 30 },
{ name: 'Doe', age: 22 },
{ name: 'Jake', age: 25 }
]);
const grouped = people.groupBy('age');
console.log(grouped.all());
// Output:
// {
// '22': [{ name: 'Doe', age: 22 }],
// '25': [{ name: 'John', age: 25 }, { name: 'Jake', age: 25 }],
// '30': [{ name: 'Jane', age: 30 }]
// }
merge
The merge
method merges the given array or object with the collection.
const merged = collection.merge([6, 7, 8]);
console.log(merged.all());
// Output: [1, 2, 3, 4, 5, 6, 7, 8]
unique
The unique
method returns all of the unique items in the collection.
const duplicates = collect([1, 2, 2, 3, 4, 4, 5]);
const uniqueItems = duplicates.unique();
console.log(uniqueItems.all());
// Output: [1, 2, 3, 4, 5]
Real-World Example
Let's consider a real-world example where collect.js
can simplify data manipulation. Suppose you have an array of users, and you need to filter out users below 18 years of age, sort them by name, and then pluck their email addresses.
const users = collect([
{ name: 'John Doe', email: '[email protected]', age: 25 },
{ name: 'Jane Doe', email: '[email protected]', age: 17 },
{ name: 'Jake Doe', email: '[email protected]', age: 20 },
{ name: 'Doe John', email: '[email protected]', age: 30 }
]);
const emails = users
.filter(user => user.age >= 18)
.sortBy('name')
.pluck('email');
console.log(emails.all());
// Output: ['[email protected]', '[email protected]', '[email protected]']
Conclusion
collect.js
is a powerful library that brings the expressive and convenient API of Laravel collections to JavaScript. Whether you are a Laravel developer or simply looking for a better way to handle arrays and objects in JavaScript, collect.js
can simplify your code and improve its readability.
By mastering collect.js
, you can leverage its numerous methods to perform complex data manipulations with ease, making your JavaScript code more efficient and easier to maintain.
For more information and to explore all the available methods, check out the official collect.js documentation.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
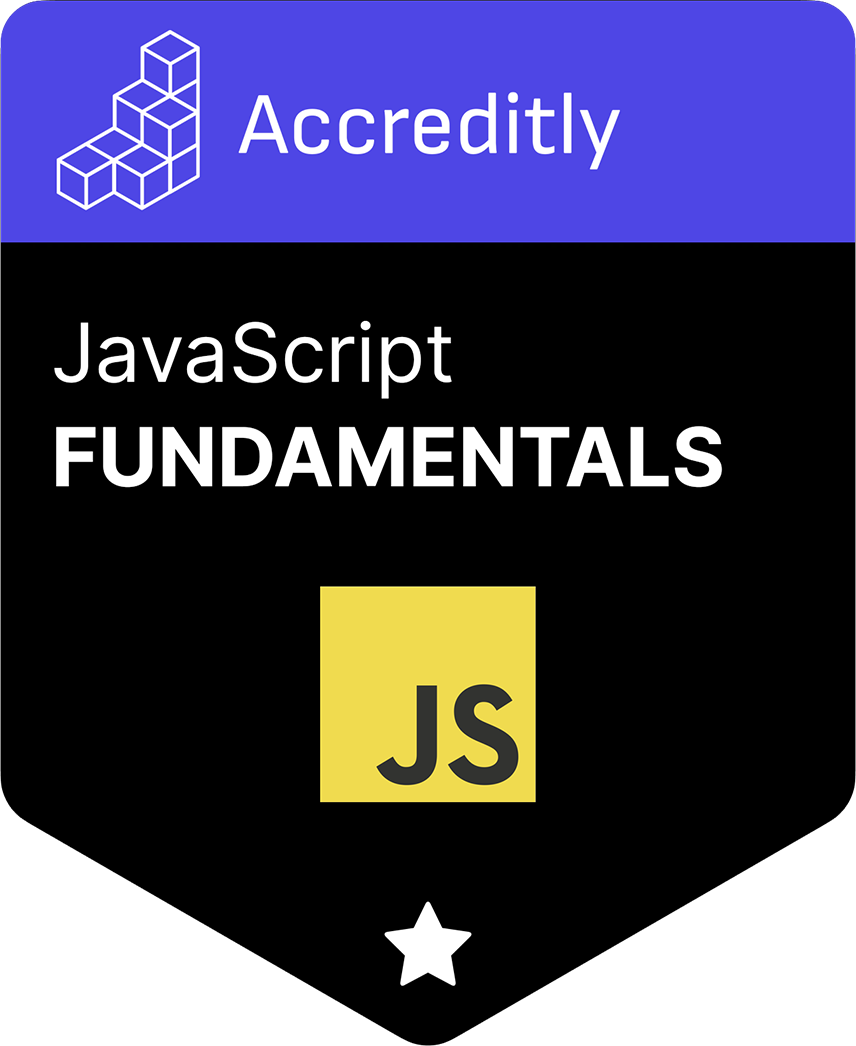