- 1. Understanding the REST API
- 2. Getting Started with the WordPress REST API
- 3. WordPress REST API Authentication
- 4. Exploring the WordPress REST API Endpoints
- 5. Performing CRUD Operations with the WordPress REST API
- 6. Extending the WordPress REST API
- Conclusion
The WordPress REST API has opened up new possibilities for developers, enabling them to create advanced applications and integrations with the popular content management system. In this comprehensive guide, we'll explore the WordPress REST API and provide you with the knowledge needed to master it as a developer. We'll cover the basics of the REST API, authentication, endpoints, and how to perform CRUD operations on various WordPress resources.
Got knowledge of this stuff already? Prove it, take a look at our WordPress Certification.
1. Understanding the REST API
The REST (Representational State Transfer) API is an architectural style that allows you to interact with your WordPress site's data using HTTP requests. The WordPress REST API exposes your site's data, such as posts, pages, media, and other resources, in a JSON format, making it easy to consume and manipulate from various client applications, including web apps, mobile apps, and other third-party integrations.
2. Getting Started with the WordPress REST API
To start using the WordPress REST API, you'll need a WordPress installation running version 4.7 or higher. The REST API is enabled by default, and you can access its endpoints through the following base URL:
https://example.com/wp-json/wp/v2
To test the API, you can use a tool like Postman or curl to send HTTP requests to the API's endpoints. Here's an example of how to retrieve all published posts using a GET request:
GET https://example.com/wp-json/wp/v2/posts
3. WordPress REST API Authentication
For public data, such as retrieving published posts or pages, the WordPress REST API doesn't require authentication. However, for protected resources, such as creating, updating, or deleting content, you'll need to authenticate your requests.
There are several authentication methods available for the WordPress REST API, including:
Cookie Authentication: This is the default authentication method for the REST API when making requests from within the WordPress admin area. It relies on the same cookies used for logging into the WordPress dashboard. OAuth 1.0a Authentication: A more secure method that allows you to authenticate using OAuth tokens instead of passing user credentials directly. This is recommended for third-party applications. JWT Authentication: A popular authentication method that uses JSON Web Tokens to authenticate requests. It's a lightweight and secure alternative to OAuth 1.0a. Choose the authentication method that best suits your application and follow the corresponding documentation to set it up.
4. Exploring the WordPress REST API Endpoints
The WordPress REST API provides numerous endpoints for interacting with your site's data. Some of the most common endpoints include:
- /wp/v2/posts: Retrieve, create, update, or delete posts.
- /wp/v2/pages: Retrieve, create, update, or delete pages.
- /wp/v2/media: Retrieve, create, update, or delete media items.
- /wp/v2/users: Retrieve, create, update, or delete users.
- /wp/v2/comments: Retrieve, create, update, or delete comments.
- /wp/v2/categories: Retrieve, create, update, or delete categories.
- /wp/v2/tags: Retrieve, create, update, or delete tags.
Each endpoint provides various query parameters that you can use to filter, sort, or paginate the results.
There are many more available too, including accessing blocks. Check out the full list on the WordPress REST API reference.
5. Performing CRUD Operations with the WordPress REST API
Using the WordPress REST API, you can perform CRUD (Create, Read, Update, Delete) operations on various resources.
5.1. Creating Resources
To create a new resource, such as a post or page, send a POST request to the corresponding endpoint with the required data in the request body. For example, to create a new post:
POST https://example.com/wp-json/wp/v2/posts
{
"title": "My New Post",
"content": "This is the content of my new post.",
"status": "publish"
}
5.2. Reading Resources
To retrieve resources, such as posts or pages, send a GET request to the corresponding endpoint. You can use query parameters to filter, sort, or paginate the results. For example, to get all published posts by a specific author:
GET https://example.com/wp-json/wp/v2/posts?author=1&status=publish
5.3. Updating Resources
To update an existing resource, such as a post or page, send a PUT or PATCH request to the corresponding endpoint with the required data in the request body. For example, to update a post's title:
PUT https://example.com/wp-json/wp/v2/posts/1
{
"title": "My Updated Post"
}
5.4. Deleting Resources
To delete a resource, such as a post or page, send a DELETE request to the corresponding endpoint. For example, to delete a post:
DELETE https://example.com/wp-json/wp/v2/posts/1
6. Extending the WordPress REST API
One of the most powerful features of the WordPress REST API is its extensibility. As a developer, you can create custom endpoints, modify existing endpoints, or even add new resources to the API.
To create a custom endpoint, you can use the register_rest_route() function in your plugin or theme's functions.php
file. Here's an example of how to create a custom endpoint that returns a list of all post titles:
add_action('rest_api_init', function () {
register_rest_route('myplugin/v1', '/post-titles', array(
'methods' => 'GET',
'callback' => 'myplugin_get_post_titles',
));
});
function myplugin_get_post_titles() {
$args = array(
'post_type' => 'post',
'post_status' => 'publish',
'posts_per_page' => -1,
'fields' => 'ids',
);
$post_ids = get_posts($args);
$post_titles = array();
foreach ($post_ids as $post_id) {
$post_titles[] = get_the_title($post_id);
}
return $post_titles;
}
This custom endpoint will be accessible at https://example.com/wp-json/myplugin/v1/post-titles
.
Conclusion
Mastering the WordPress REST API opens up a world of possibilities for developers to create advanced applications and integrations with the popular content management system. By understanding the basics of the REST API, authentication, endpoints, and CRUD operations, you'll be well on your way to leveraging the full potential of the WordPress REST API in your projects. Don't forget to explore the API's extensibility by creating custom endpoints and resources tailored to your needs.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
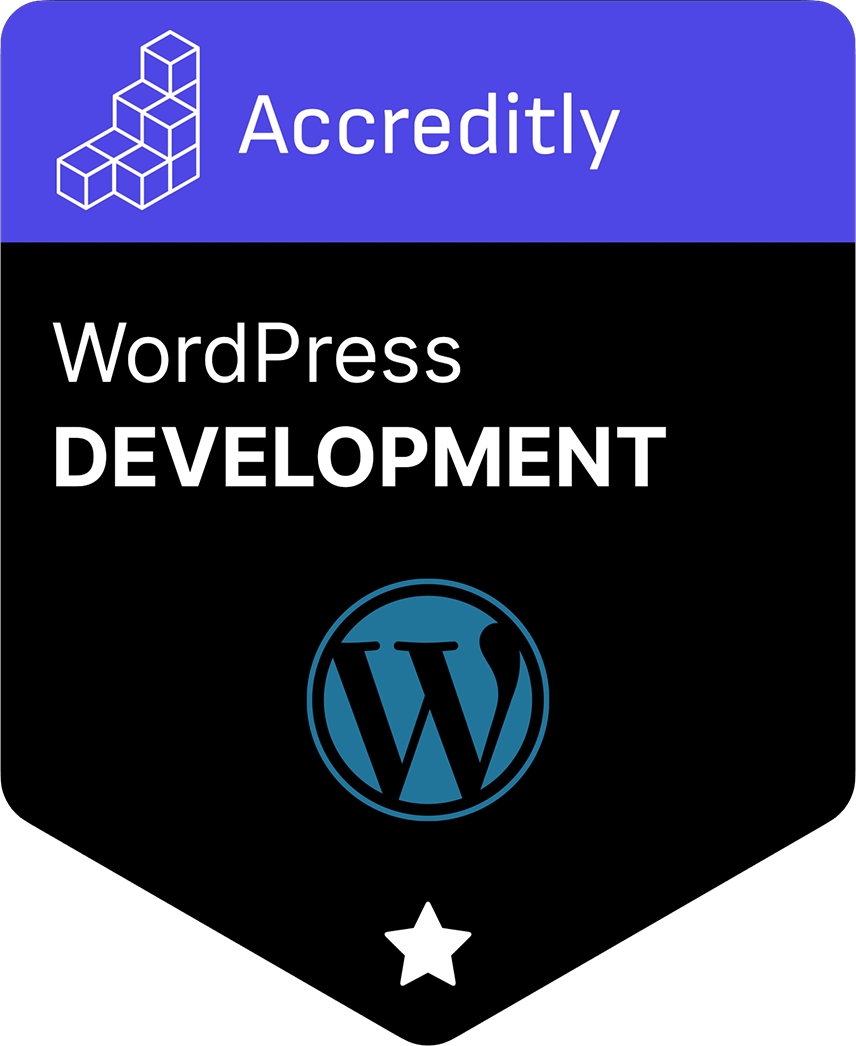