- Understanding the Need for Sharing Data
- Method 1: Using wp_localize_script()
- Method 2: Inline Script Tag with wp_add_inline_script()
- Security Considerations
In WordPress development, there are often scenarios where you need to pass data from PHP (server-side) to JavaScript (client-side). Whether it's to initialize a JavaScript plugin with server-side settings, pass user data for a personalized experience, or provide API URLs for AJAX calls, understanding how to bridge PHP and JavaScript is crucial. This article explores the mechanisms WordPress offers for this purpose, ensuring your data flows securely and efficiently between back-end and front-end.
Understanding the Need for Sharing Data
Integrating PHP and JavaScript allows for dynamic and interactive user experiences. WordPress, being a PHP-based content management system, generates HTML pages served to the user's browser where JavaScript runs. The direct sharing of data from PHP scripts to JavaScript enriches the possibilities for interactive, data-driven front-end development.
wp_localize_script()
Method 1: Using The wp_localize_script()
function is WordPress's native solution for passing PHP data to JavaScript. Despite its name suggesting localization, it's widely used for echoing PHP variables to JavaScript.
How to Use wp_localize_script()
:
-
Enqueue Your JavaScript File: First, ensure your JavaScript file is properly enqueued using
wp_enqueue_script()
.
function mytheme_enqueue_scripts() {
wp_enqueue_script('mytheme-script', get_template_directory_uri() . '/js/my-script.js', array(), '1.0.0', true);
}
add_action('wp_enqueue_scripts', 'mytheme_enqueue_scripts');
-
Localize the Script: After enqueuing the script, use
wp_localize_script()
to attach data to it. This function must be called afterwp_enqueue_script()
.
function mytheme_localize_script() {
wp_localize_script('mytheme-script', 'myThemeData', array(
'ajax_url' => admin_url('admin-ajax.php'),
'nonce' => wp_create_nonce('mytheme_nonce'),
'customData' => 'This is some data',
));
}
add_action('wp_enqueue_scripts', 'mytheme_localize_script');
-
Access Data in JavaScript: In your enqueued JavaScript file, you can access the data using the object named in the second parameter of
wp_localize_script()
, in this case,myThemeData
.
console.log(myThemeData.customData); // Outputs: This is some data
wp_add_inline_script()
Method 2: Inline Script Tag with For more complex data or when you prefer not to use the localization approach, wp_add_inline_script()
allows you to add inline JavaScript code directly after an enqueued script file. This method is particularly useful for structured data like arrays or objects that don't fit well with the wp_localize_script()
approach.
How to Use wp_add_inline_script()
:
-
Enqueue Your JavaScript File: Ensure your JavaScript file is enqueued.
-
Add Inline Script: Use
wp_add_inline_script()
to attach your PHP data as a JavaScript snippet. Make sure to properly encode your PHP data withwp_json_encode()
to ensure JavaScript compatibility.
function mytheme_inline_script() {
$data = array(
'ajax_url' => admin_url('admin-ajax.php'),
'nonce' => wp_create_nonce('mytheme_nonce'),
'customData' => 'This is some data',
);
wp_add_inline_script('mytheme-script', 'const myInlineData = ' . wp_json_encode($data) . ';', 'before');
}
add_action('wp_enqueue_scripts', 'mytheme_inline_script');
-
Access Data in JavaScript: The data is now directly accessible as a JavaScript variable (
myInlineData
in the example above).
Security Considerations
When sharing data between PHP and JavaScript, especially data related to WordPress internals or user information, always consider security:
- For actions that make changes (e.g., form submissions via AJAX), use nonces to verify requests.
- Ensure any dynamic data passed to JavaScript is properly sanitized and escaped to prevent cross-site scripting (XSS) attacks.
- Only pass the data necessary for the task at hand to minimize potential exposure.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
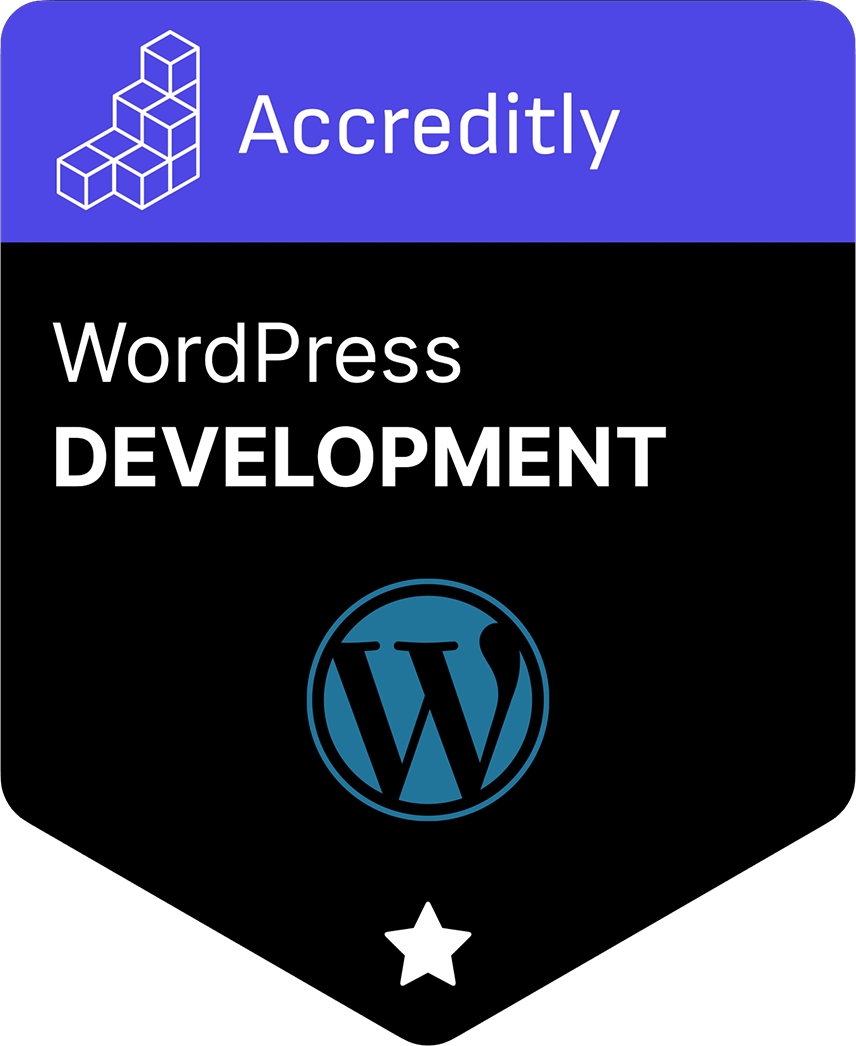