- Understanding NTP
- Gotchas
- List of NTP servers
- Checking Time with NTP in JavaScript
- Checking Time with NTP in PHP
- Security and Performance Considerations
Accurate timekeeping is crucial for many applications, especially those involving scheduling, timestamps, or coordination of events in distributed systems. Network Time Protocol (NTP) servers provide a way to synchronize clocks over a network. In this article, we explore how to check and synchronize the time of a client or server against an NTP server using JavaScript for client-side operations and PHP for server-side operations.
Understanding NTP
NTP is a networking protocol designed to synchronize clocks of computers over a network. It uses a hierarchical, semi-layered system of time sources. Each level of this system is called a "stratum" where stratum 0 contains highly precise timekeeping devices such as atomic clocks and GPS clocks. Common public NTP servers include those provided by organizations like pool.ntp.org, which offers a large cluster of timeservers providing reliable easy access to NTP.
Gotchas
NTP servers respond with timestamps from 1990, not 1970 like a unix timestamp, so if you're working with unix timestamps you'll need to offset by 2208988800
seconds to ensure the correct time.
List of NTP servers
The following NTP servers are very popular and reliable. In our examples below we'll use pool.ntp.org
, but feel free to swap out for any you feel appropriate from this list.
-
NTP Pool Project
- Global: 0.pool.ntp.org, 1.pool.ntp.org, 2.pool.ntp.org, 3.pool.ntp.org
- USA: us.pool.ntp.org
- Europe: europe.pool.ntp.org
- Asia: asia.pool.ntp.org
-
Google Public NTP
-
Facebook NTP Servers
-
Microsoft NTP Server
-
Apple NTP Server
-
National Institute of Standards and Technology (NIST)
Checking Time with NTP in JavaScript
JavaScript does not have native support for directly querying NTP servers due to browser security restrictions that prevent direct UDP socket communication. However, you can work around this by querying a server-side script that performs the NTP communication. Here's how you can do this using AJAX to interact with a PHP script:
1. JavaScript (Client-side):
fetch('getServerTime.php')
.then(response => response.json())
.then(data => {
console.log('Server Time:', data.serverTime);
console.log('Offset from Local Time:', Date.now() - new Date(data.serverTime).getTime());
})
.catch(error => console.error('Error fetching server time:', error));
This JavaScript code makes a call to a PHP script (getServerTime.php
) that returns the server time. It then calculates the difference between the local time and the server time.
Checking Time with NTP in PHP
On the server-side, PHP can interact with an NTP server to get accurate time data using sockets. Here’s how you can write a PHP script to query an NTP server:
2. PHP (Server-side):
<?php
function getNtpTime($host = 'pool.ntp.org') {
$socket = socket_create(AF_INET, SOCK_DGRAM, SOL_UDP);
// NTP request header
$msg = "\010" . str_repeat("\0", 47);
$server = gethostbyname($host);
socket_sendto($socket, $msg, strlen($msg), 0, $server, 123);
socket_recvfrom($socket, $recv, 48, 0, $server, $port);
// Unpack binary to unsigned long
$data = unpack('N12', $recv);
$timestamp = sprintf('%u', $data[9]);
// NTP timestamps are from 1900, Unix from 1970
$timestamp -= 2208988800;
return gmdate('Y-m-d H:i:s', $timestamp);
}
header('Content-Type: application/json');
echo json_encode(['serverTime' => getNtpTime()]);
?>
This PHP script creates a UDP socket to communicate with an NTP server, sends a formatted request, and receives the time, which it then converts from NTP's format to a human-readable form and outputs as JSON.
Security and Performance Considerations
- When implementing server-side scripts that communicate with external services, always consider potential security implications, including rate limiting and input validation.
- Regularly synchronize time in applications where precise timing is critical, but also ensure that frequent checks do not overwhelm the network or the NTP servers.
While JavaScript lacks direct capabilities to interact with NTP servers, it can work in conjunction with server-side scripts to ensure your application maintains accurate timekeeping. This approach allows for the flexibility of client-side operations with the robustness and accuracy of server-side time checking against standardized time sources. Proper implementation ensures that your application can effectively use accurate time stamps for any purpose that requires synchronization with world clocks or coordination between distributed systems.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
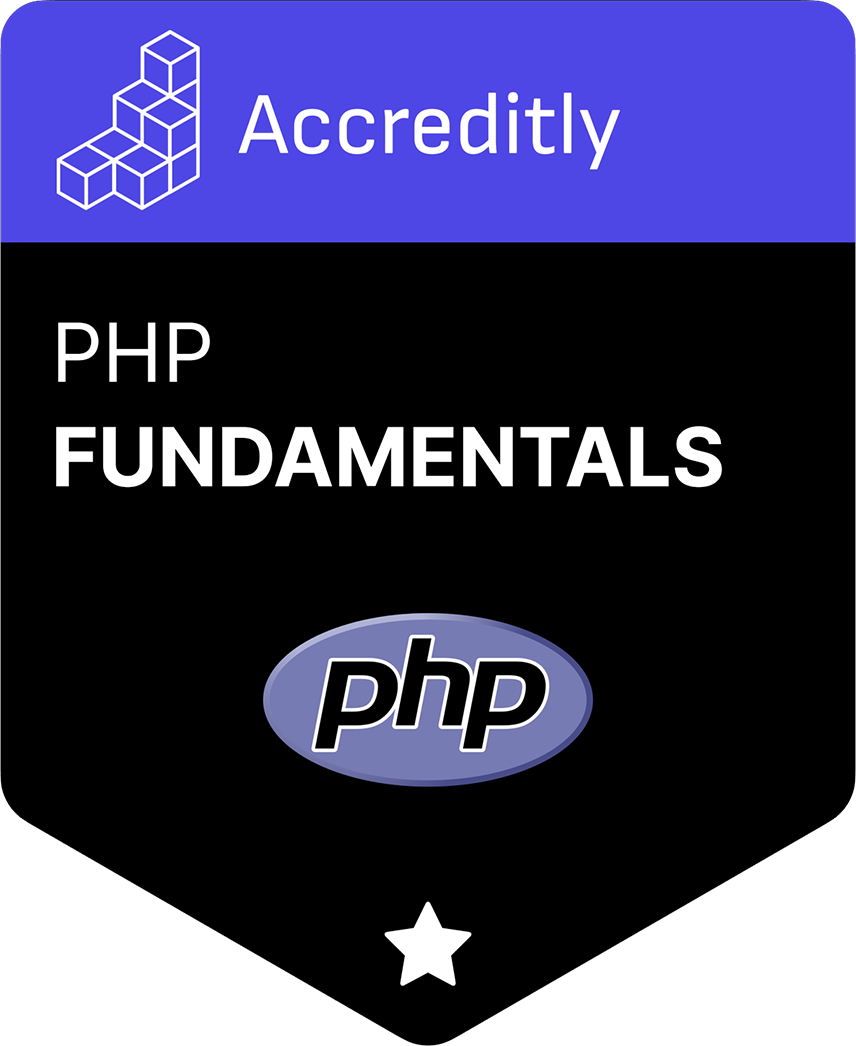