- Installing the PHP dotenv Library
- Setting Up Environment Variables
- Loading Environment Variables
- Accessing Environment Variables
Environmental variables are an essential part of application development, particularly when dealing with sensitive information such as API keys, database credentials, and other configuration data. Environment variables enable developers to keep sensitive data out of the codebase. This article guides you through storing and accessing environment variables in PHP applications using the vlucas/phpdotenv
library.
Installing the PHP dotenv Library
To get started, install the vlucas/phpdotenv library via Composer. If you don't have Composer installed, you can download it from the official website.
composer require vlucas/phpdotenv
Setting Up Environment Variables
Once you have installed the dotenv library, create a new .env
file in the root directory of your project. This file will store all of your environment variables:
DB_HOST=localhost
DB_NAME=sample_db
DB_USER=username
DB_PASSWORD=password
API_KEY=your_api_key
Each line in the .env
file represents an environment variable. The variable name is followed by an equals sign (=) and the variable value.
Loading Environment Variables
To load the environment variables, you need to initialize a new instance of the Dotenv\Dotenv
class and call the load()
method. Typically, you would do this at the start of your application, such as in the bootstrap or configuration file:
<?php
require_once __DIR__ . '/vendor/autoload.php';
$dotenv = Dotenv\Dotenv::createImmutable(__DIR__);
$dotenv->load();
In the above code, the createImmutable()
method takes the path of the directory containing your .env
file. Then, the load()
method is called to load the environment variables.
Accessing Environment Variables
After loading the environment variables, you can access them using the getenv()
, $_ENV
, or $_SERVER
superglobal:
$dbHost = getenv('DB_HOST');
$dbName = $_ENV['DB_NAME'];
$dbUser = $_SERVER['DB_USER'];
All these methods will return the corresponding value of the environment variable.
Storing sensitive data in environment variables is a good practice for application security and configuration. By using the vlucas/phpdotenv library in your PHP applications, you can efficiently manage your environment variables. Remember never to commit your .env
files into version control systems to keep your sensitive data secure.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
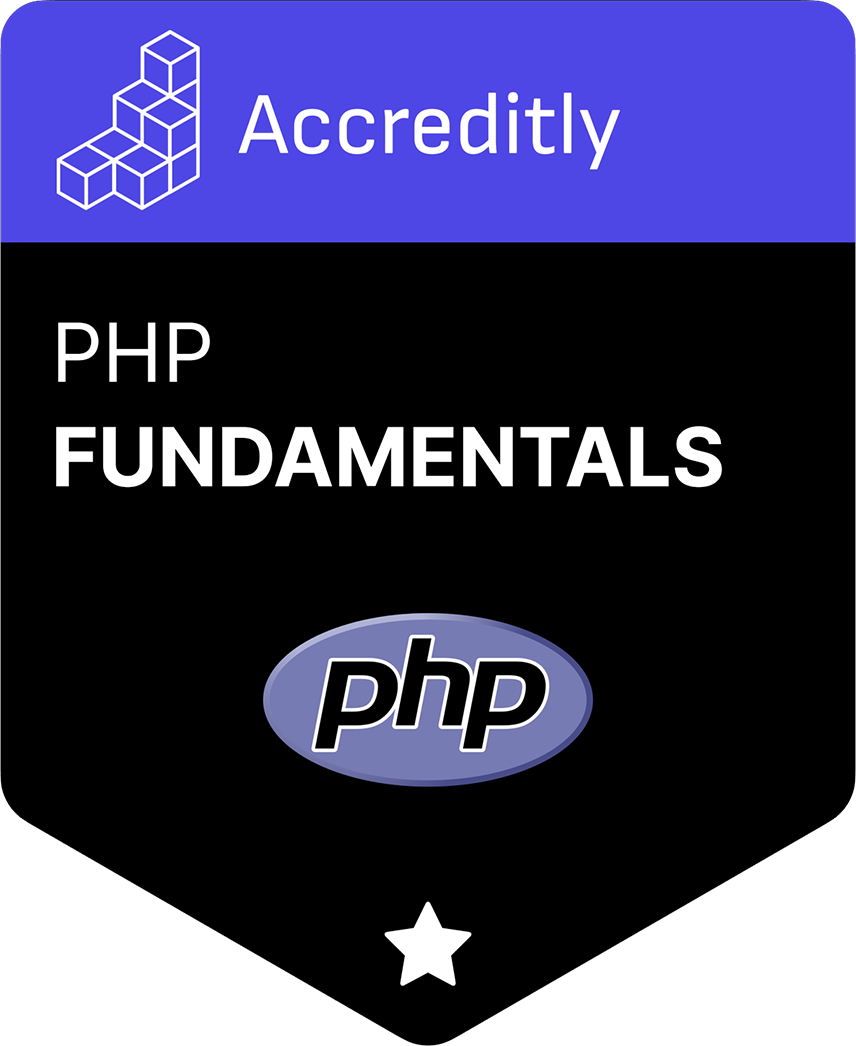