- Understanding Laravel Livewire
- Laravel Livewire vs. Vue.js
- Livewire Actions and Data Binding
- Making the Transition from Vue.js to Laravel Livewire
- Lifecycle Hooks
- Validation
- Summary
As a Vue.js developer, you're probably comfortable creating responsive, modern web applications. But if you've found your way to this article, you might be exploring new tools or methodologies to streamline your development process. Laravel Livewire could be exactly what you're looking for.
Livewire is a full-stack framework for Laravel that allows you to build dynamic interfaces simply, without leaving the comfort of Laravel. The framework leverages the power of Laravel's Blade templating engine and provides an environment similar to Vue.js. This article will introduce Laravel Livewire, drawing comparisons and contrasts with Vue.js to help you get started.
Understanding Laravel Livewire
Before we dive in, let's define Laravel Livewire. Livewire is a full-stack framework developed by Caleb Porzio, which allows you to write interactive user interfaces using PHP, in a Vue.js-like style. Livewire relies heavily on Laravel's Blade templating engine and AJAX under the hood, providing a seamless and simple way to build dynamic web apps without the need for extensive JavaScript or a separate API layer.
Laravel Livewire vs. Vue.js
Let's draw a comparison between Laravel Livewire and Vue.js to understand their similarities and differences better.
Components
Both Livewire and Vue.js make extensive use of components. Vue.js developers will be familiar with this concept, as Vue.js is heavily component-based. In Vue.js, a component might look like this:
Vue.component('example-component', {
data: function () {
return {
count: 0
}
},
template: '<button v-on:click="count++">You clicked me {{ count }} times.</button>'
});
In Laravel Livewire, components are similarly constructed but are divided into two separate files: a class file (which holds our data and actions) and a view file (which holds our HTML). Here's the Livewire equivalent:
// ExampleComponent.php
namespace App\Http\Livewire;
use Livewire\Component;
class ExampleComponent extends Component
{
public $count = 0;
public function increment()
{
$this->count++;
}
public function render()
{
return view('livewire.example-component');
}
}
// example-component.blade.php
<button wire:click="increment">You clicked me {{ $count }} times.</button>
As you can see, Livewire components are quite similar to Vue.js components, but they bring along the benefits of server-side rendering, such as SEO-friendliness and compatibility with old browsers.
A Livewire component is comprised of both a blade template and a PHP class. In the above example you'll see that we need to write much more code in the Livewire component (well we don't really, as it's mostly generated for us using php artisan make:livewire ExampleComponent
!), but in more complex components this will result in far less code when compared to Vue and a server-side component.
Livewire Actions and Data Binding
Event Handling
In Vue.js, we're used to handling DOM events using v-on:click or the shorthand @click. Livewire offers a similar way of handling events using the wire:click directive:
Vue.js:
<button @click="count++">Increment</button>
Livewire:
<button wire:click="increment">Increment</button>
Data Binding
Similarly, two-way data binding in Livewire is like Vue.js. In Vue.js, we can bind an input field using v-model:
Vue.js:
<input v-model="name">
In Livewire, you can achieve the same result using wire:model
:
Livewire:
<input wire:model="name">
Making the Transition from Vue.js to Laravel Livewire
Transitioning from Vue.js to Laravel Livewire might seem daunting at first, but if you're familiar with Vue.js, you'll find a lot of common ground. Both frameworks are component-based and provide similar features like data-binding and event handling.
Lifecycle Hooks
Just like Vue.js, Laravel Livewire offers a variety of lifecycle hooks for controlling when certain actions should be executed. This is similar to Vue.js lifecycle hooks like created, mounted, updated, etc.
In Livewire, there are three primary lifecycle hooks:
- mount: This method is called when the component is first instantiated and is a great place to put setup code.
- render: This method is called on every subsequent request (including after actions are called) and should return the rendered component view.
- hydrate: This method is called on every subsequent request and after the component has been hydrated with request data.
Here's how you can define these hooks in a Livewire component:
class ExampleComponent extends Component
{
public $name;
public function mount()
{
// This will run when the component is first instantiated...
}
public function render()
{
// This will run on every subsequent request...
return view('livewire.example-component');
}
public function hydrate()
{
// This will run on every subsequent request, after the component has been hydrated with request data...
}
}
There are lots of other lifecycle hooks available too.
Validation
In Vue.js, we often use libraries like Vuelidate or Vue-Formulate to handle form validation. Livewire, being tightly integrated with Laravel, allows us to use Laravel's built-in validation features, which is pretty neat.
Here's how you can define a simple form and handle form submission and validation in a Livewire component:
class ContactForm extends Component
{
public $email;
public $message;
protected $rules = [
'email' => 'required|email',
'message' => 'required',
];
public function submit()
{
$this->validate();
// Form submission logic...
}
public function render()
{
return view('livewire.contact-form');
}
}
The validate method
will automatically validate the component's public properties based on the rules you've defined in the $rules
property. If validation fails, an exception will be thrown and the component's render method will be called again to re-render the component with error messages.
You get all of the power of Laravel and its validation system baked right into Livewire, so defining your own rules is trivial.
<form wire:submit.prevent="submit">
<input type="text" wire:model="email">
@error('email') <span>{{ $message }}</span> @enderror
<textarea wire:model="message"></textarea>
@error('message') <span>{{ $message }}</span> @enderror
<button type="submit">Send</button>
</form>
Summary
This article has just scratched the surface of what's possible with Laravel Livewire. If you're coming from a Vue.js background, you'll find many similarities and might enjoy the benefits that Livewire offers, such as tighter integration with Laravel and server-side rendering.
Learning Laravel Livewire doesn't mean you have to abandon Vue.js. In fact, having both in your toolbox will make you a more versatile developer. Depending on the project requirements, you might find Vue.js to be a better fit, or you might prefer Laravel Livewire. Ultimately, the choice is yours.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
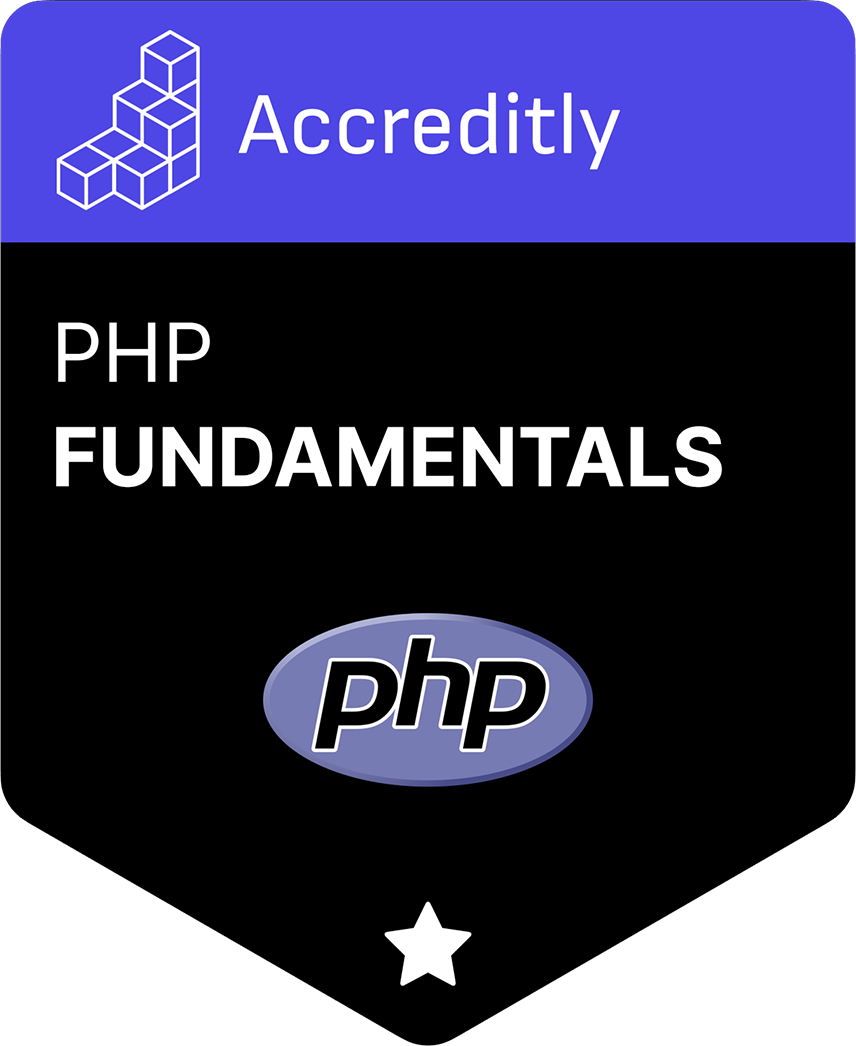