- What is the N+1 Problem?
- A JavaScript Example of the N+1 Problem
- Why is the N+1 Problem Bad?
- How to Identify the N+1 Problem in JavaScript
- Avoiding the N+1 Problem in JavaScript
- Advanced Techniques: Optimizing API and Database Interactions
- Conclusion
The N+1 problem is a well-known performance issue in software development that can affect any application interacting with a database or an API to retrieve related data. Although it's commonly associated with server-side languages and ORMs, the N+1 problem is also relevant in JavaScript, especially when working with Node.js, frontend frameworks, or any scenario where your code fetches related data in multiple network requests. In this article, we will explore the N+1 problem within the context of JavaScript, understand its implications, and discuss strategies to avoid it.
What is the N+1 Problem?
At its core, the N+1 problem occurs when an application makes N additional requests to fetch related data for N objects that have already been retrieved. This issue often arises when data is fetched lazily, one item at a time, rather than all at once in an optimized manner.
A JavaScript Example of the N+1 Problem
Let’s walk through a typical scenario where the N+1 problem might occur in a JavaScript application, particularly in a Node.js environment using a database.
Imagine you have a REST API that needs to retrieve a list of users and, for each user, fetch their associated orders from a database. A naive implementation might look like this:
async function getUsersWithOrders() {
// Fetch all users
const users = await db.query('SELECT * FROM users');
// For each user, fetch their orders
for (let user of users) {
user.orders = await db.query('SELECT * FROM orders WHERE user_id = ?', [user.id]);
}
return users;
}
In this example, if there are 10 users in the database, this code will execute 11 queries:
- One query to retrieve all users:
SELECT * FROM users;
- Then, for each user, an additional query to fetch their orders:
SELECT * FROM orders WHERE user_id = ?;
This results in an N+1 problem, where N is the number of users. The more users you have, the more database queries are executed, leading to increased latency and unnecessary load on the database.
Why is the N+1 Problem Bad?
The N+1 problem can severely degrade your application’s performance, particularly as the size of the dataset grows. Here are a few reasons why the N+1 problem is problematic in a JavaScript context:
-
Increased Latency: Each additional network request or database query introduces latency. In a Node.js application, this can slow down the response times for your API, leading to a poor user experience.
-
Higher Resource Consumption: Making many small queries or requests increases the load on your database or external APIs, consuming more resources and potentially leading to rate limits or throttling.
-
Scalability Issues: As your application scales and the number of records increases, the N+1 problem becomes more pronounced, making your application less scalable and more challenging to maintain.
How to Identify the N+1 Problem in JavaScript
Detecting the N+1 problem in your JavaScript code involves monitoring the number of requests or queries made during a particular operation. You can log these operations and review the patterns to see if multiple redundant queries or requests are being made.
For example, in a Node.js application using an SQL database, you can log the queries executed during a request lifecycle:
db.on('query', (query) => {
console.log(query.sql);
});
Similarly, if you are working with an API, you can log the HTTP requests made by your application to identify potential N+1 issues:
const fetch = require('node-fetch');
async function getData(url) {
console.log(`Fetching: ${url}`);
const response = await fetch(url);
return response.json();
}
By examining the logs, you can identify if your application is making more requests or queries than necessary.
Avoiding the N+1 Problem in JavaScript
Avoiding the N+1 problem in JavaScript typically involves fetching related data in bulk rather than in individual requests. Here are some strategies to achieve this:
1. Batch Requests
If you're working with an API, one solution is to batch multiple requests into a single call, reducing the number of round trips. For example, if your API supports fetching multiple resources at once, you can modify your code to take advantage of this:
async function getUsersWithOrders() {
// Fetch all users
const users = await db.query('SELECT * FROM users');
// Get a list of all user IDs
const userIds = users.map(user => user.id);
// Fetch all orders for these users in one query
const orders = await db.query('SELECT * FROM orders WHERE user_id IN (?)', [userIds]);
// Map orders to the corresponding users
const userOrders = users.map(user => {
user.orders = orders.filter(order => order.user_id === user.id);
return user;
});
return userOrders;
}
This change reduces the number of queries from N+1 to just two, regardless of how many users are in the database.
2. Use Eager Loading
If you're using an ORM like Sequelize or TypeORM in your Node.js application, you can use eager loading to fetch related data in a single query instead of making additional queries for each relationship. Here’s how you might do it with Sequelize:
const users = await User.findAll({
include: [{
model: Order,
as: 'orders'
}]
});
This generates a single SQL query that joins the users
and orders
tables, fetching all the required data in one go.
3. GraphQL: Efficient Data Fetching
In the context of APIs, especially when using GraphQL, you can avoid the N+1 problem by carefully structuring your resolvers. GraphQL allows you to batch queries or use DataLoader, a library that batches and caches requests, ensuring that each field resolver doesn’t result in a separate database query:
const DataLoader = require('dataloader');
// Create a DataLoader for orders
const orderLoader = new DataLoader(async (userIds) => {
const orders = await db.query('SELECT * FROM orders WHERE user_id IN (?)', [userIds]);
return userIds.map(userId => orders.filter(order => order.user_id === userId));
});
// Use the DataLoader in your resolver
const resolvers = {
User: {
orders: (user) => orderLoader.load(user.id)
}
};
This approach minimizes the number of database queries, significantly reducing the potential for the N+1 problem.
Advanced Techniques: Optimizing API and Database Interactions
Beyond the basic solutions, you can employ more advanced techniques to optimize your API and database interactions:
-
Caching: Implement caching strategies at different layers of your application to avoid unnecessary queries. This can include caching database results, HTTP responses, or using in-memory caches like Redis.
-
Optimizing Queries: Write more efficient SQL queries or API calls that retrieve all necessary data in one go, avoiding the need for multiple round-trips.
-
Pagination and Limits: When dealing with large datasets, implement pagination and limits to fetch only the data you need at any given time, reducing the risk of performance bottlenecks.
Conclusion
The N+1 problem is a common issue in JavaScript applications, particularly when dealing with databases or APIs. Understanding how it occurs and implementing strategies to avoid it is crucial for building efficient, scalable applications. Whether you’re working with Node.js, frontend frameworks, or any JavaScript environment, being mindful of the N+1 problem will help you optimize your data fetching strategies, ensuring that your application remains performant as it grows.
By using techniques like batch requests, eager loading, and DataLoader, you can significantly reduce the number of queries or requests your application makes, improving both performance and user experience.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
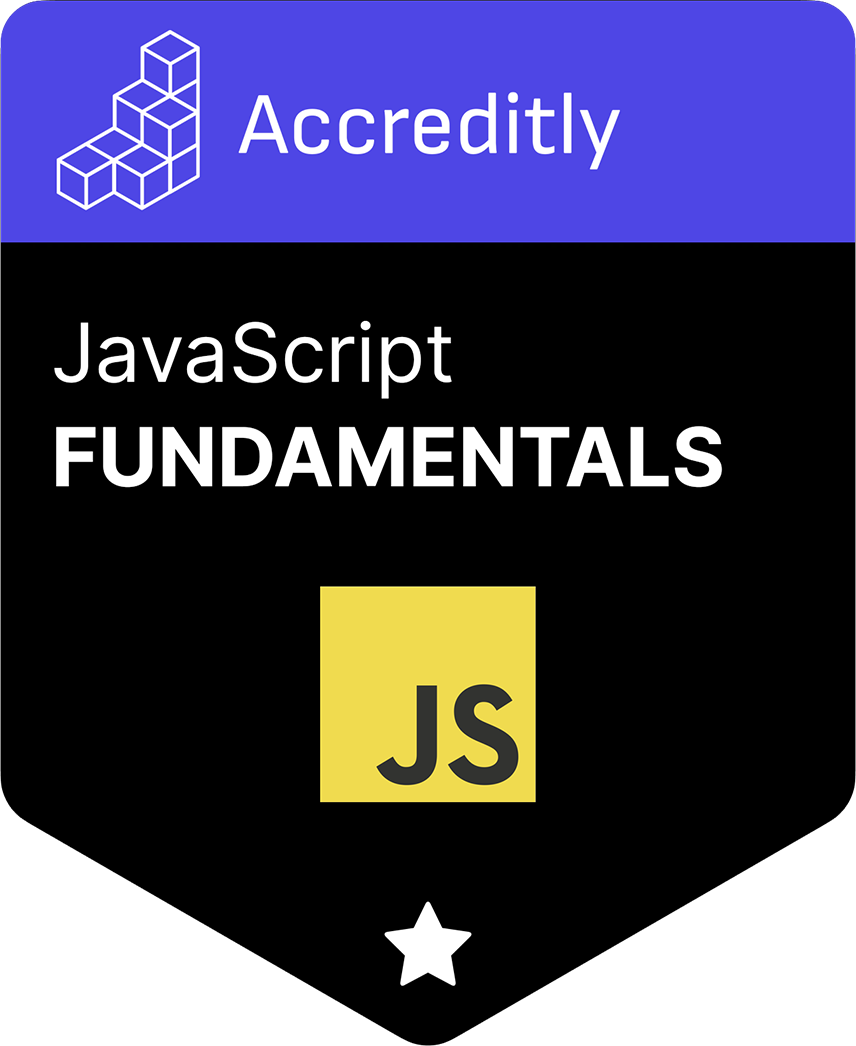