- 1. Copying Text to Clipboard
- 2. Pasting Text from Clipboard
- 3. Use Cases and Practical Examples
- 4. Important Considerations
The ability to programmatically copy and paste content using JavaScript can be quite valuable for enhancing user experience. Think of features like "click to copy" for promo codes or email addresses. In this guide, we will delve into how you can implement copy and paste functionalities in your web applications using JavaScript.
1. Copying Text to Clipboard
Using the Clipboard API
The Clipboard API provides a straightforward way to interact with the clipboard.
function copyTextToClipboard(text) {
navigator.clipboard.writeText(text).then(function() {
console.log('Text successfully copied!');
}).catch(function(err) {
console.error('Unable to copy text: ', err);
});
}
// Usage
const btnCopy = document.getElementById('btnCopy');
btnCopy.addEventListener('click', function() {
const textToCopy = document.getElementById('textToCopy').value;
copyTextToClipboard(textToCopy);
});
document.execCommand
Fallback Using For older browsers, you can use the document.execCommand('copy')
method. However, this is now considered obsolete.
function fallbackCopyTextToClipboard(text) {
const textArea = document.createElement('textarea');
textArea.value = text;
document.body.appendChild(textArea);
textArea.focus();
textArea.select();
try {
document.execCommand('copy');
console.log('Text successfully copied!');
} catch (err) {
console.error('Unable to copy text: ', err);
}
document.body.removeChild(textArea);
}
2. Pasting Text from Clipboard
Pasting content from the clipboard using the Clipboard API is similar to copying. However, for security reasons, most browsers will prompt the user for permission.
function pasteTextFromClipboard() {
navigator.clipboard.readText().then(function(clipboardText) {
console.log('Pasted content from clipboard:', clipboardText);
}).catch(function(err) {
console.error('Unable to paste text: ', err);
});
}
// Usage
const btnPaste = document.getElementById('btnPaste');
btnPaste.addEventListener('click', function() {
pasteTextFromClipboard();
});
3. Use Cases and Practical Examples
Click to Copy a Promo Code
A common e-commerce pattern is to offer promo codes that users can copy to their clipboard with one click.
document.getElementById('promoCode').addEventListener('click', function() {
const promoCode = this.textContent;
copyTextToClipboard(promoCode);
alert('Promo code copied to clipboard!');
});
Paste Content into a Textbox
Allow users to paste content from their clipboard into a textbox, which could be useful for features like "import from clipboard."
document.getElementById('pasteContent').addEventListener('click', function() {
pasteTextFromClipboard();
});
4. Important Considerations
- Security: Be careful when dealing with clipboard data. Ensure that you handle the pasted content safely, especially if you plan to use or store it.
- Browser Support: While the Clipboard API is widely supported in modern browsers, always ensure you've implemented fallbacks for older ones, especially if you're targeting a broad user base. Sometimes libraries can help with this, but try and keep it vanilla if you can.
- User Experience: Always notify users when content is copied or pasted, typically with a quick alert or notification.
Incorporating copy and paste functionalities can significantly boost your web application's user experience. By leveraging JavaScript and the Clipboard API, developers have the tools they need to make content sharing a breeze. However, always consider security and browser compatibility to ensure a seamless experience for your users.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
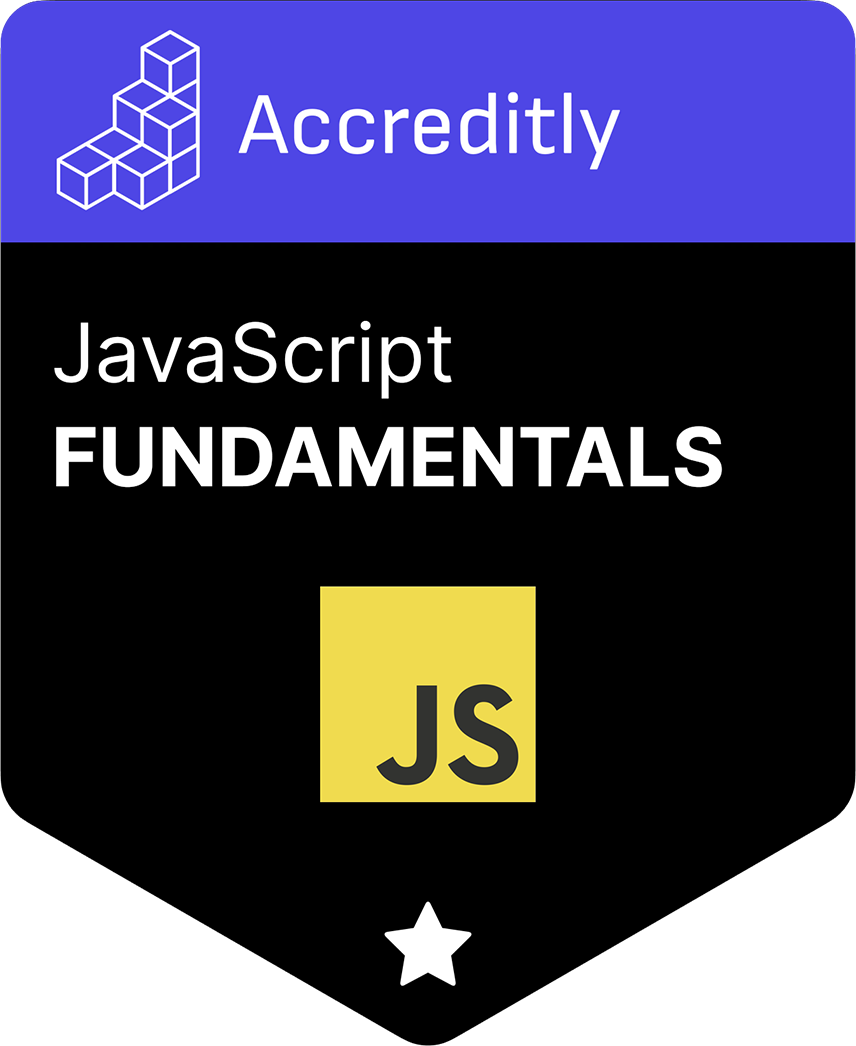