- Preparation
- Step 1: Define Your Opening Hours
- Step 2: Display Opening Hours
- Step 3: Add the Function to Your Theme
- Step 4: Styling
Incorporating opening hours directly into a WordPress theme not only provides essential information to your visitors but also enhances user engagement by dynamically highlighting the current day and showing real-time open/closed status. This approach requires a bit of PHP coding and an understanding of how to modify your theme files. By the end of this article, you'll know how to implement a fully dynamic opening hours feature into your WordPress theme.
Preparation
Before diving into the code, decide where you want the opening hours to appear on your site. Common places include the footer, sidebar, or a dedicated contact page. Ensure you have access to your theme files, either through an FTP client or your hosting file manager.
Step 1: Define Your Opening Hours
Start by defining your opening hours. For this example, we'll store the hours in an array. Place this code in your theme's functions.php
file or in a custom plugin.
function get_business_hours() {
return [
'Monday' => ['08:00', '17:00'],
'Tuesday' => ['08:00', '17:00'],
'Wednesday' => ['08:00', '17:00'],
'Thursday' => ['08:00', '17:00'],
'Friday' => ['08:00', '17:00'],
'Saturday' => ['10:00', '14:00'],
'Sunday' => 'closed',
];
}
Step 2: Display Opening Hours
Next, create a function to display the opening hours. This function checks the current day and time to highlight today's hours and indicate if the business is currently open.
function display_opening_hours() {
$currentDay = date('l'); // Get the current day
$currentTime = date('H:i'); // Get the current time
$hours = get_business_hours();
foreach ($hours as $day => $times) {
echo "<div";
if ($day === $currentDay) {
echo " style='font-weight:bold;'"; // Highlight the current day
if (is_array($times)) {
[$open, $close] = $times;
$isOpen = $currentTime >= $open && $currentTime <= $close;
echo ">{$day}: {$open} - {$close}";
echo $isOpen ? " <span style='color:green;'>Open</span>" : " <span style='color:red;'>Closed</span>";
} else {
echo ">{$day}: Closed";
}
} else {
echo ">{$day}: ";
echo is_array($times) ? "{$times[0]} - {$times[1]}" : "Closed";
}
echo "</div>";
}
}
This function iterates over the opening hours, checks if the current day matches each day in the loop, and applies styling to highlight it. It also determines if the current time falls within today's opening hours to display an "Open" or "Closed" status.
Step 3: Add the Function to Your Theme
To integrate the opening hours into your site, call display_opening_hours()
in the appropriate theme file. For instance, to add it to the footer, edit footer.php
, and place the following where you want the hours to appear:
<?php display_opening_hours(); ?>
Step 4: Styling
The function includes basic inline styling to highlight the current day and indicate the open/closed status. For more advanced styling, consider adding classes to the elements and styling them via your theme's CSS file:
.open-status {
font-weight: bold;
}
.open-status.open {
color: green;
}
.open-status.closed {
color: red;
}
You'd then modify the PHP function to use these classes instead of inline styles.
Integrating dynamic opening hours into your WordPress theme enhances the user experience by providing valuable information in an interactive format. Highlighting the current day and displaying the real-time open/closed status can significantly impact how users interact with your site, planning their visits according to your business hours. While the implementation requires a bit of coding, the end result is a tailored feature that fits seamlessly into your theme, reflecting the professionalism and attention to detail of your business.
Remember, whenever editing theme files, it's good practice to use a child theme to preserve your changes against future theme updates.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
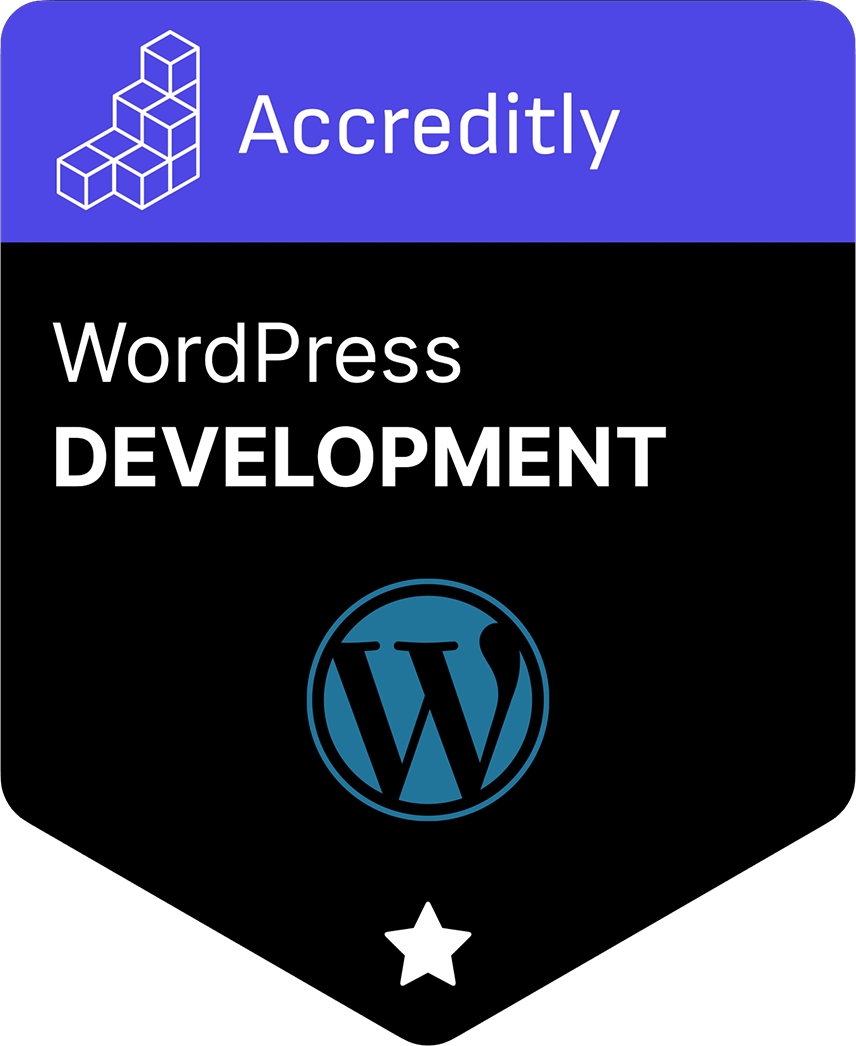