- Step 1: Set Up User Tracking
- Step 2: Create a Function to Count Online Users
- Step 3: Displaying Online Users
User interaction is a key indicator of a website's health and vitality. One interactive element that can boost engagement and create a sense of community is displaying the number of users currently online on your WordPress website. This feature can be particularly useful for membership sites, forums, or e-commerce platforms where user engagement is paramount.
In this tutorial, we'll go through the process of creating a functionality in WordPress that allows you to display the number of users currently online.
Step 1: Set Up User Tracking
Firstly, we'll need to start tracking when users are active on our website. We can do this by setting a transient whenever a user loads a page. The transient will expire after a certain amount of time, indicating that the user is no longer active.
Add the following code to your theme's functions.php
file:
function track_user_online() {
if (is_user_logged_in()) {
$current_user = wp_get_current_user();
set_transient('user_online_' . $current_user->ID, true, 300);
}
}
add_action('wp', 'track_user_online');
Check out the docs for set_transient()
.
In this code snippet, we're setting a transient that lasts for 300 seconds (or 5 minutes) whenever a logged-in user loads a page. The transient's name includes the user's ID to ensure it's unique.
Step 2: Create a Function to Count Online Users
Next, we need to create a function that counts how many users are currently online. We can do this by looping through all of our users and checking if the transient for each user is set.
function get_online_users() {
$user_query = new WP_User_Query(array('fields' => array('ID')));
$users = $user_query->get_results();
$online_count = 0;
foreach ($users as $user_id) {
if (get_transient('user_online_' . $user_id)) {
$online_count++;
}
}
return $online_count;
}
This function queries all users and checks if their user_online_[ID]
transient is set. If the transient is set, it means the user has been active in the last 5 minutes.
Check out the docs for get_transient()
.
Step 3: Displaying Online Users
Now we have a function that gets the number of online users. Let's use this function to display the number of online users on the frontend. You can call the function get_online_users()
in your template files like so:
echo '<p>Online Users: ' . get_online_users() . '</p>';
Remember to place the code where you want the number of online users to be displayed, such as in a sidebar widget, footer, or header.
By following this guide, you can show the number of users currently online on your WordPress website. This provides a real-time view of user activity and fosters community interaction. However, it's important to note that this approach only tracks logged-in users and is more suitable for websites with a substantial user base. If your site receives significant traffic from non-logged-in users, you may need to consider more sophisticated tracking methods, such as setting an arbitrary pseudo user ID in a session variable against the user and using that to track your transient entries. However, just be aware of heavy read/writes on your database for high traffic sites.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
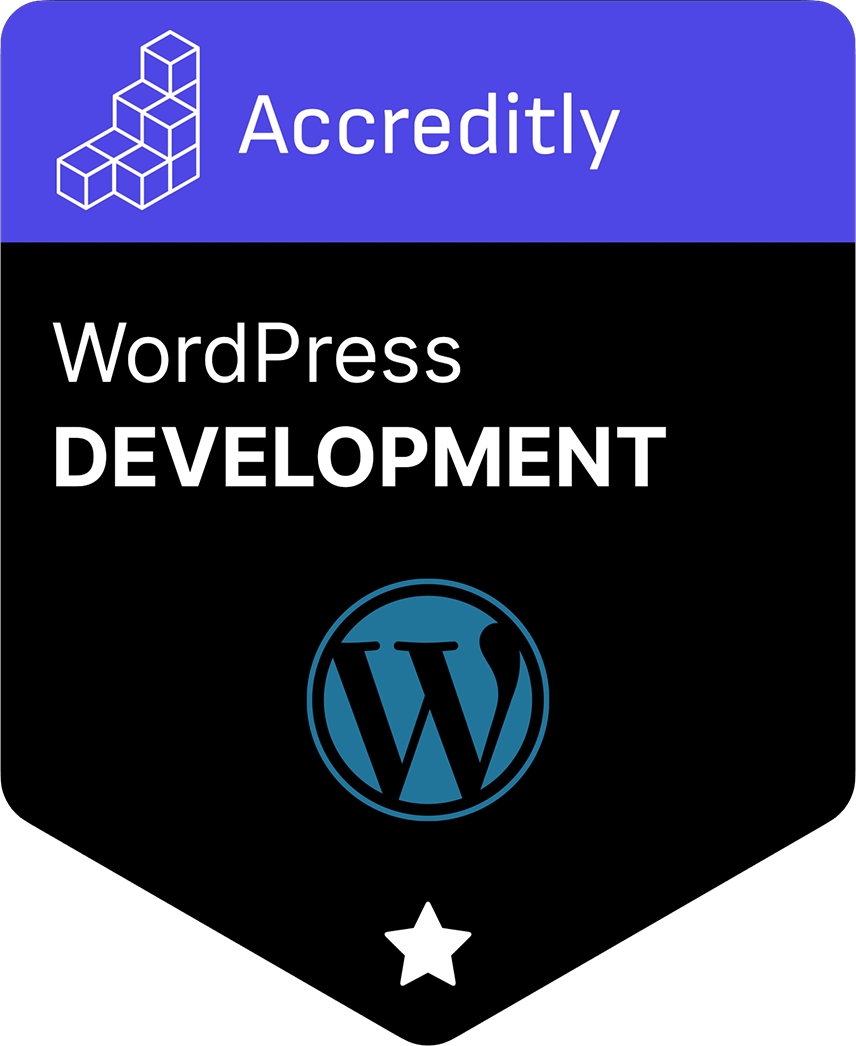