- Step 1: Setting Up Your Project
- Step 2: Install Commander
- Step 3: Create Your CLI Tool
- Step 4: Enable Running Your Script
- Step 5: Testing Your CLI Tool
- Conclusion
JavaScript isn't just for web browsers. With Node.js, you can use JavaScript to build versatile command-line applications (CLIs) that perform tasks, handle automation, or even interact with APIs and process data. In this tutorial, you'll learn how to build a basic CLI tool using Node.js and the commander
library, which simplifies the creation of command-line interfaces.
Step 1: Setting Up Your Project
To start, you need Node.js installed on your computer. If you haven't installed Node.js yet, you can download it from nodejs.org.
-
Create a New Directory for Your Project:
mkdir my-cli-app cd my-cli-app
-
Initialize a New Node.js Project: Run
npm init
ornpm init -y
(to skip the questions and accept defaults) to create apackage.json
file that will manage your project dependencies and metadata.
Step 2: Install Commander
The commander
library is a popular choice for handling command-line options and commands in Node.js applications. Install it by running:
npm install commander
Step 3: Create Your CLI Tool
Create a new file called index.js
. This file will be the entry point of your CLI application.
-
Import Commander and Set Up Basic Command:
const { program } = require('commander'); program .version('0.1.0') .description('An example CLI for processing files'); program.parse(process.argv);
-
Add Commands and Options: You can define commands and options that your CLI will accept. For instance, let’s add a command to process a file:
program .command('process <file>') .description('Process the specified file') .option('-u, --uppercase', 'Output in uppercase') .action((file, options) => { const fs = require('fs'); fs.readFile(file, 'utf8', (err, data) => { if (err) { console.error(err); return; } let output = data; if (options.uppercase) { output = output.toUpperCase(); } console.log(output); }); }); program.parse(process.argv);
This command
process
expects a filename and has an optional flag-u
or--uppercase
to convert text to uppercase.
Step 4: Enable Running Your Script
-
Make
index.js
Executable: Add a shebang line at the top ofindex.js
to specify the interpreter:#!/usr/bin/env node
-
Update
package.json
: Add abin
section to yourpackage.json
to specify thatindex.js
can be installed as an executable:"bin": { "my-cli-app": "./index.js" }
-
Link Your Application (for development): Run
npm link
in your project directory. This command creates a global symlink to your project, allowing you to run your CLI tool from anywhere on your system usingmy-cli-app
.
Step 5: Testing Your CLI Tool
Open a terminal and try running your new CLI tool:
my-cli-app process example.txt --uppercase
This should display the contents of example.txt
in uppercase, assuming the file exists and is readable.
Conclusion
Building command-line applications in JavaScript with Node.js and the commander
library allows you to create powerful tools for a variety of tasks, from simple file processing to complex automation scripts. This tutorial covered the basics to get you started. As you become more comfortable, consider adding more features like error handling, more complex commands, and integrating other Node.js modules to expand the functionality of your CLI tool. Happy coding!
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
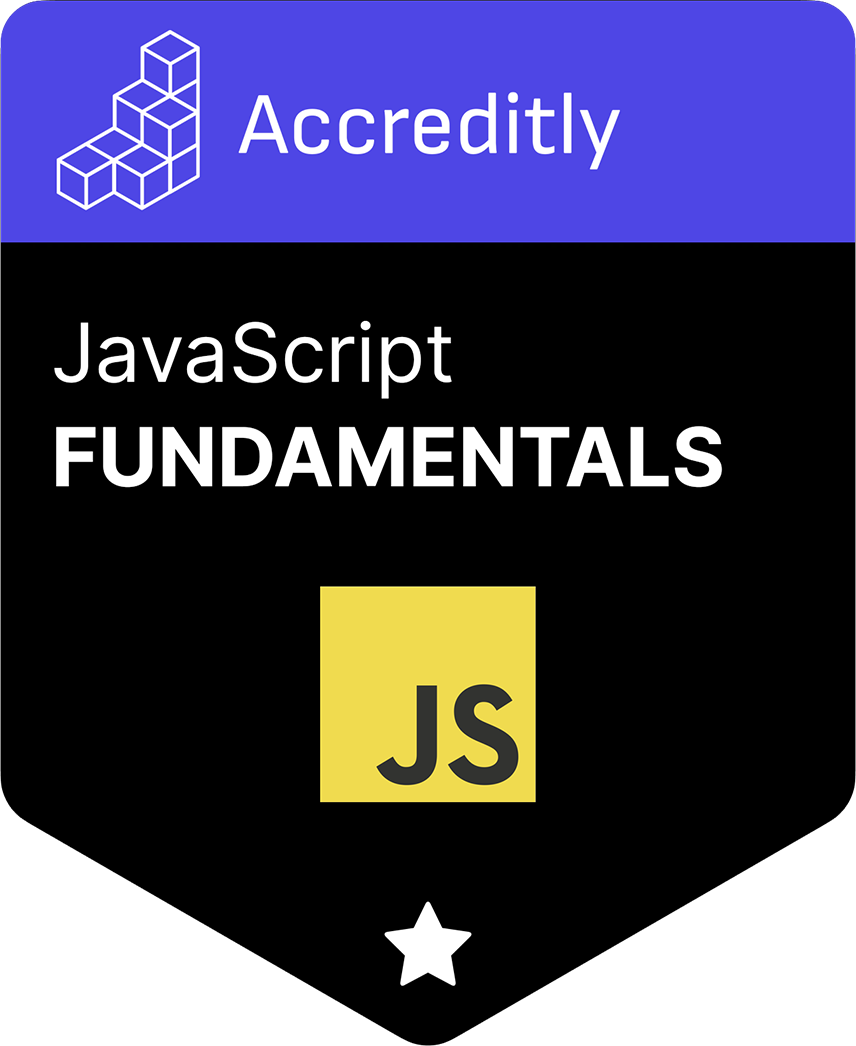