- Understanding Serialize and JSON Encode
- Looking at the data
- Pros and Cons of Serialize and JSON Encode
PHP offers two popular methods for storing complex data structures: serialize
and JSON encoding via json_encode
. Both methods are powerful and have their unique use cases, but it can be challenging to decide which one is the right choice for your project. In this article, we'll dive into the differences between serialize and JSON encode, their pros and cons, and when it's appropriate to use each approach. We'll also provide code examples to illustrate their usage in real-world scenarios.
Understanding Serialize and JSON Encode
Serialize
PHP's serialize
function converts complex data types (such as arrays and objects) into a storable format, which can then be easily stored in a database or a file. When you need to retrieve the data, you can use the unserialize
function to convert it back to its original form.
Here's an example of using serialize
and unserialize
:
$data = array(
'name' => 'John Doe',
'age' => 30,
'email' => '[email protected]'
);
$serialized_data = serialize($data);
// Store the serialized data in a file
file_put_contents('data.txt', $serialized_data);
// Read the serialized data from the file
$serialized_data = file_get_contents('data.txt');
// Unserialize the data
$unserialized_data = unserialize($serialized_data);
print_r($unserialized_data);
Here we take an array, serialize it, store that array into a text file and then load it again.
JSON Encode
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. PHP provides the json_encode
function to convert data structures into JSON format and the json_decode
function to convert JSON data back to their original PHP data structures.
Here's an example of using json_encode
and json_decode
:
$data = array(
'name' => 'John Doe',
'age' => 30,
'email' => '[email protected]'
);
$json_data = json_encode($data);
// Store the JSON data in a file
file_put_contents('data.json', $json_data);
// Read the JSON data from the file
$json_data = file_get_contents('data.json');
// Decode the JSON data
$decoded_data = json_decode($json_data, true);
print_r($decoded_data);
In this example we do the exact same thing as the previous example but with JSON; take an array, encode it as JSON, save it to a file and then read it again.
Looking at the data
The data itself will look different when you look at the strings created by serialize
and json_encode
. Let's start by taking a look at the string created in the above example.
Serialize
a:3:{s:4:"name";s:8:"John Doe";s:3:"age";i:30;s:5:"email";s:16:"[email protected]";}
The data elements themselves such as the keys and values are readable, but they are surrounded by various characters (eg a:3
, s:4
, s:8
, etc) that are less readable.
These additional characters are what unserialize
uses internally to extra the data back into an array or object. The general premise is that a letter dictate the data type, so in the above example a
denotes an array
and s
denotes a string
. The numbers denote the length of the item, so a:3
means an array with 3 items, and s:4
denotes a string of length 4.
Although this makes sense and isn't too difficult to read once you understand the characters it does make it a lot more difficult to manipulate. You cannot easily edit the serialized data without loading it back into PHP. That may not be a problem for your use case but it will be an issue if you need to store this data in a database and update values on-mass.
JSON
Now let's look at the JSON equivalent:
{"name":"John Doe","age":30,"email":"[email protected]"}
It's a much shorter string as it doesn't contain the strings that denote data types, this saves on transfer bytes and storage bytes. It's also easier to read and, more importantly, easier to edit.
Pros and Cons of Serialize and JSON Encode
Serialize
Pros:
- Preserves data types: Serialize preserves data types, such as objects and resources, which can be essential when working with custom classes or third-party libraries.
- Language-specific: Since serialize is a PHP-specific function, it works best when storing data that will only be used within PHP applications.
Cons:
- Less human-readable: Serialized data can be harder to read and understand, making debugging more difficult.
- Incompatible with other languages: Serialize is PHP-specific, so sharing serialized data with applications written in other languages can be challenging.
- Not easily editable: Similarly to the previous point, it's not easy to edit the raw data.
JSON Encode
Pros:
- Human-readable: JSON data is more readable than serialized data, making it easier to understand and debug.
- Human-editable: You can open it in a text editor and edit it, simple. This means you can also edit it on-mass in a database.
- Language-independent: JSON is a widely supported format across various programming languages, making it ideal for sharing data between applications written in different languages.
Cons:
- Limited data types: JSON does not support all PHP data types, such as resources and certain objects. Converting these data types to JSON may result in loss of information.
- Less efficient for PHP-only applications: If you're only working with PHP, using JSON may not be as efficient as using serialize, especially when it comes to preserving data types.
Both serialize and JSON encode offer powerful ways to store and manage complex data structures in PHP. Choosing the right approach depends on factors such as data types, readability, and compatibility with other programming languages. Serialize is better suited for PHP-only applications or when preserving complex data types is crucial, while JSON encode is ideal for sharing data across different languages or when human readability is a priority.
And, of course, sometimes neither of these options will suffice and you may need to consider something else.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
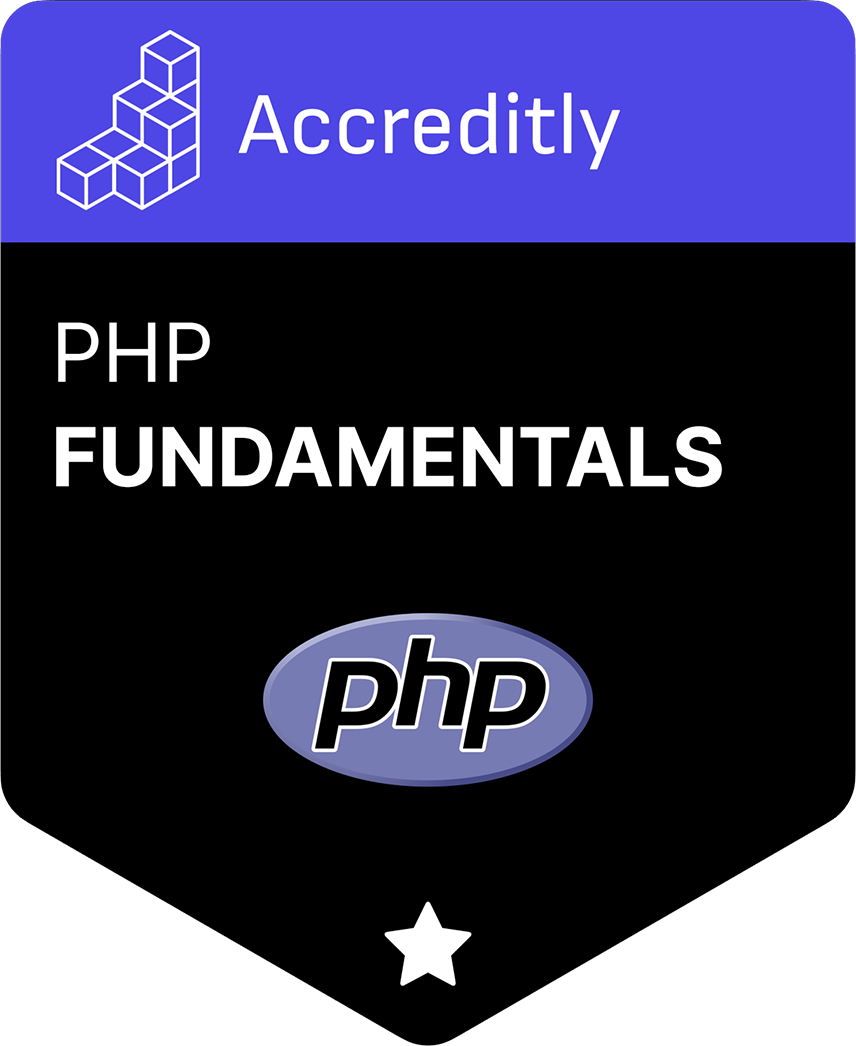