- Basics of Laravel Scheduling
-
Available Scheduling Frequencies
- 1. ->everyMinute();
- 2. ->everyTwoMinutes();
- 3. ->everyFiveMinutes();
- 4. ->everyTenMinutes();
- 5. ->everyFifteenMinutes();
- 6. ->everyThirtyMinutes();
- 7. ->hourly();
- 8. ->hourlyAt(17);
- 9. ->daily();
- 10. ->dailyAt('13:00');
- 11. ->twiceDaily(1, 13);
- 12. ->weekly();
- 13. ->weeklyOn(1, '8:00');
- 14. ->monthly();
- 15. ->monthlyOn(4, '15:00');
- 16. ->quarterly();
- 17. ->yearly();
- 18. ->weekdays();
- 19. ->weekends();
- Combining Constraints
Laravel's task scheduling is a powerful feature that simplifies the management of periodic tasks such as sending out emails, cleaning up databases, or generating reports. Instead of creating a Cron job for each task, Laravel allows you to define your schedule within your application, providing a more readable and maintainable approach. This article provides an overview of all available scheduling schedules in Laravel.
Basics of Laravel Scheduling
Laravel's scheduler is defined in the app/Console/Kernel.php
file. The scheduler offers a fluent API to expressively define the frequency of commands and tasks. To enable it, a single Cron entry on your server is required, which executes the Laravel scheduler every minute:
* * * * * cd /path-to-your-project && php artisan schedule:run >> /dev/null 2>&1
Available Scheduling Frequencies
Laravel offers a variety of scheduling frequencies, allowing for granular control over task execution. Here are the most commonly used options:
->everyMinute();
1. The task will run every minute:
$schedule->command('emails:send')->everyMinute();
->everyTwoMinutes();
2. To run a task every two minutes:
$schedule->command('emails:send')->everyTwoMinutes();
->everyFiveMinutes();
3. For tasks that should occur every five minutes:
$schedule->command('emails:send')->everyFiveMinutes();
->everyTenMinutes();
4. To schedule a task every ten minutes:
$schedule->command('emails:send')->everyTenMinutes();
->everyFifteenMinutes();
5. To run a task every fifteen minutes:
$schedule->command('emails:send')->everyFifteenMinutes();
->everyThirtyMinutes();
6. For tasks that should run every thirty minutes:
$schedule->command('emails:send')->everyThirtyMinutes();
->hourly();
7. To run a task at the beginning of every hour:
$schedule->command('report:generate')->hourly();
->hourlyAt(17);
8. Run a task at a specific minute past the hour:
$schedule->command('report:generate')->hourlyAt(17);
->daily();
9. For tasks that should run once a day:
$schedule->command('database:backup')->daily();
->dailyAt('13:00');
10. To run a task every day at a specific time:
$schedule->command('reminder:send')->dailyAt('13:00');
->twiceDaily(1, 13);
11. To run a task twice a day at specific times:
$schedule->command('report:generate')->twiceDaily(1, 13);
->weekly();
12. For tasks that should run once a week:
$schedule->command('emails:clean')->weekly();
->weeklyOn(1, '8:00');
13. Run a task on a specific day and time of the week (0 = Sunday, 1 = Monday, etc.):
$schedule->command('report:weekly')->weeklyOn(1, '8:00');
->monthly();
14. To run a task once a month:
$schedule->command('billing:generate')->monthly();
->monthlyOn(4, '15:00');
15. Run a task on a specific day of the month at a specific time:
$schedule->command('subscription:renew')->monthlyOn(4, '15:00');
->quarterly();
16. For tasks that should run every quarter:
$schedule->command('report:quarterly')->quarterly();
->yearly();
17. To run a task once a year:
$schedule->command('audit:yearly')->yearly();
->weekdays();
18. Run a task every weekday:
$schedule->command('report:daily')->weekdays();
->weekends();
19. For tasks to run on weekends:
$schedule->command('server:restart')->weekends();
Combining Constraints
You can combine multiple time constraints for a single task:
$schedule->command('report:generate')
->weekdays()
->at('23:00');
Laravel's scheduling system provides a robust and fluent way to manage timed tasks in your application. With a wide range of scheduling options, you can tailor tasks to run precisely when needed, keeping your application efficient and responsive. By learning these scheduling capabilities, you can ensure your Laravel application performs optimally with minimal manual intervention.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
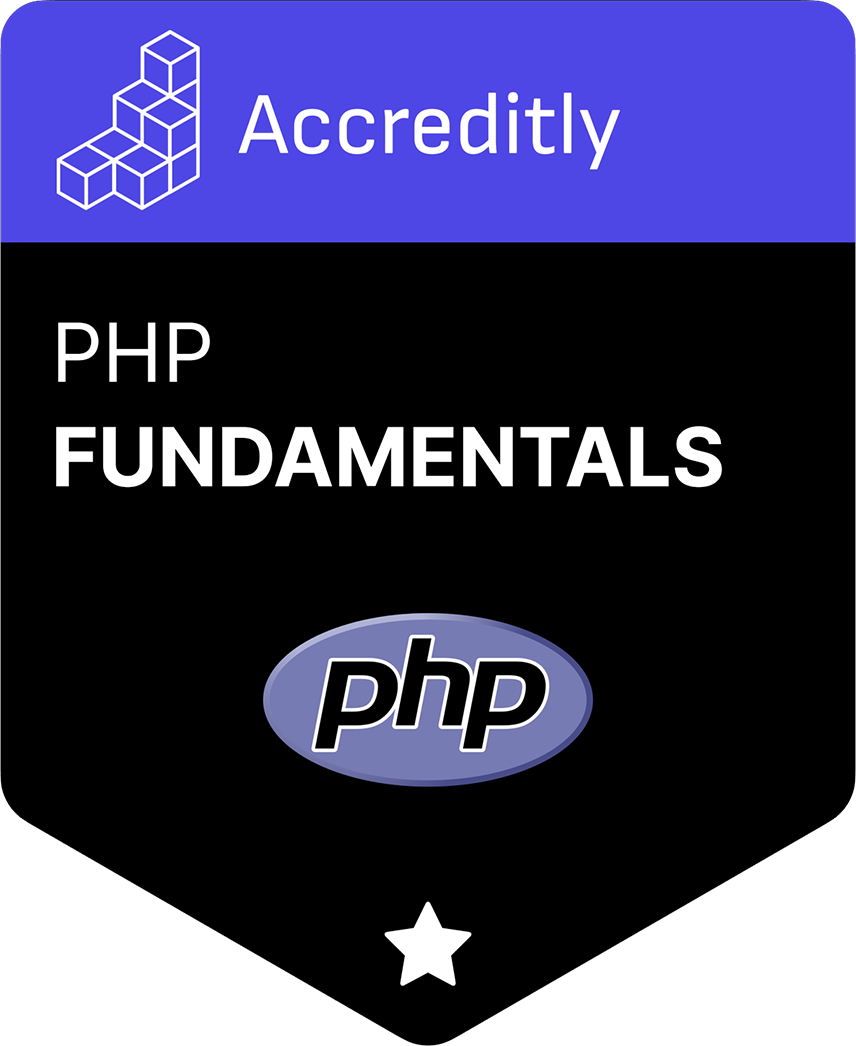