- Understanding Vite
- Prerequisites
- Step 1: Initialize Your Project
- Step 2: Install Vite
- Step 3: Set Up Your Project Structure
- Step 4: Configure Vite
- Step 5: Update index.html
- Step 6: Write Your SCSS and JavaScript
- Step 7: Running Your Project
- Step 8: Building for Production
Vite, a modern front-end build tool, has gained popularity for its fast performance and simplicity. Particularly for projects using SCSS (Sassy CSS) and JavaScript, Vite offers an efficient way to handle compilation and bundling. Let's walk through the process of setting up Vite in a basic HTML project to compile SCSS and JavaScript.
Understanding Vite
Vite, designed by the creator of Vue.js, is a build tool that significantly improves the frontend development experience. It leverages modern browser features like native ES modules to serve code during development, leading to faster start times and instant updates.
Prerequisites
Before setting up Vite, ensure you have Node.js installed on your system, as Vite requires it to run.
Step 1: Initialize Your Project
Create a new directory for your project and initialize it with npm
:
mkdir my-vite-project
cd my-vite-project
npm init -y
This command creates a basic package.json
file in your project.
Step 2: Install Vite
Install Vite as a dev dependency using npm:
npm install vite --save-dev
Step 3: Set Up Your Project Structure
Create the initial project files and directories:
-
index.html
: Your main HTML file. -
src
: A directory to hold your SCSS and JavaScript files.
- main.js
: Your main JavaScript file.
- style.scss
: Your main SCSS file.
Step 4: Configure Vite
Vite automatically picks up configuration from the vite.config.js
file. Create this file in your project root:
// vite.config.js
import { defineConfig } from 'vite';
export default defineConfig({
// Configuration options
});
Vite can handle SCSS out of the box, so no extra SCSS-specific configuration is needed.
index.html
Step 5: Update Link your JavaScript file in your index.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Vite App</title>
</head>
<body>
<div id="app"></div>
<script type="module" src="/src/main.js"></script>
</body>
</html>
Step 6: Write Your SCSS and JavaScript
In src/style.scss
, write your SCSS:
body {
background-color: pink;
}
In src/main.js
, import your SCSS file and write your JavaScript:
import './style.scss';
console.log('Hello from Vite');
Step 7: Running Your Project
Add a start script to your package.json
:
"scripts": {
"dev": "vite"
}
Run your project:
npm run dev
This will start the Vite development server. Visit the URL provided in the terminal to see your project.
Step 8: Building for Production
To build your project for production, add a build script:
"scripts": {
"build": "vite build"
}
Run the build command:
npm run build
Vite compiles your SCSS and JavaScript, optimizes them, and outputs the production files in the dist
directory.
Setting up Vite in a basic HTML project for SCSS and JavaScript compilation is straightforward. With Vite, you benefit from fast build times and an efficient development experience. Whether you're working on a small project or a large-scale application, Vite is an excellent tool that streamlines your front-end development process.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
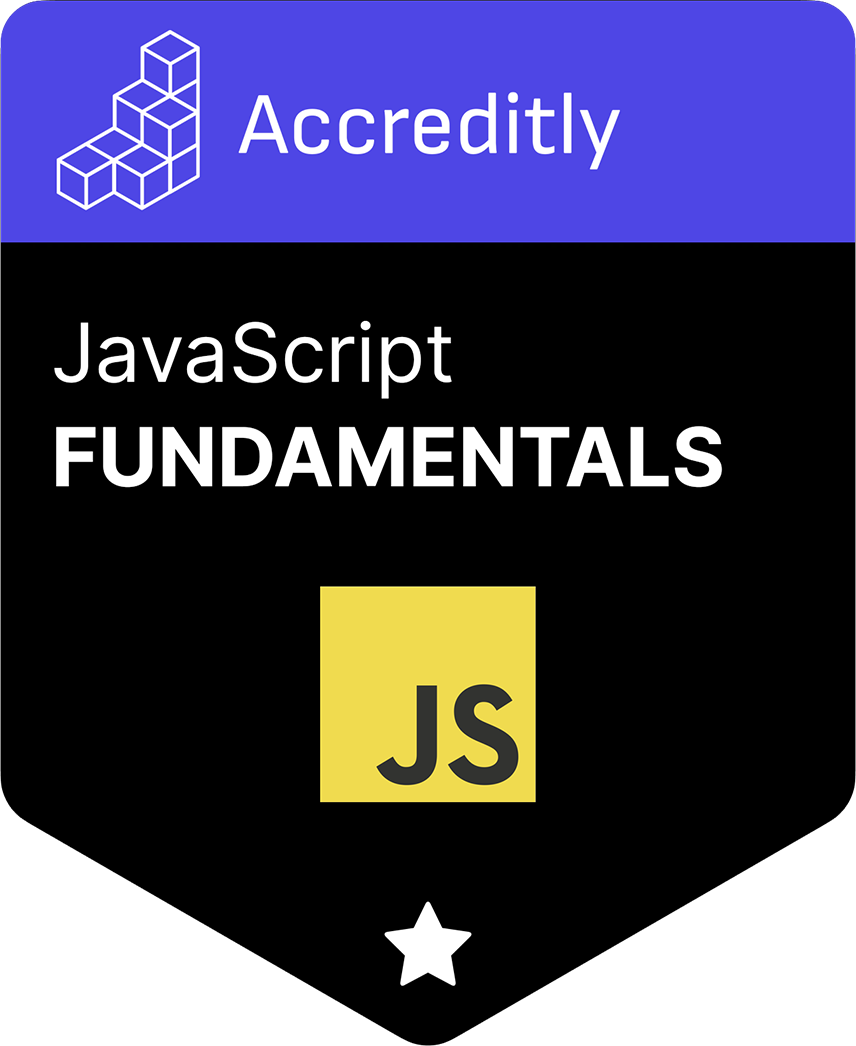