- Understanding the Read-Write Database Pattern
- Setting Up Database Servers
- Configuring WordPress for Multiple Database Connections
- Implementing Read and Write Logic
- Remember
In high-traffic WordPress environments, optimizing database interactions is crucial for performance. One effective strategy is implementing a read-write database pattern. This involves using multiple MySQL connections for read operations and a single connection for write operations. Here, we'll delve into the intricacies of setting up and managing this configuration, with a special focus on handling read and write operations.
Understanding the Read-Write Database Pattern
This pattern separates database operations based on their nature: 'read' queries are distributed across several 'slave' databases, and 'write' queries are directed to a single 'master' database. This approach balances load, enhances scalability, and improves overall performance.
Setting Up Database Servers
Firstly, set up your MySQL servers: one 'master' for write operations and multiple 'slave' servers for read operations. Configure MySQL replication to ensure the slave databases mirror the master database.
Configuring WordPress for Multiple Database Connections
In wp-config.php
, define your slave databases:
define('DB_HOST_READ1', 'read1.example.com');
define('DB_HOST_READ2', 'read2.example.com');
// Add more as needed
Implementing Read and Write Logic
This is the crux of the read-write separation. You'll need to extend WordPress's wpdb
class to create a custom database router. This router will decide whether a database query should be executed against a read or write connection.
- Creating a Custom Database Router:
In your wp-config.php
or a custom plugin, define a new class extending wpdb
:
class Custom_Read_Write_DB extends wpdb {
public $read_connections = [];
public $write_connection;
function __construct() {
// Initialize the master database (write connection)
$this->write_connection = new wpdb(DB_USER, DB_PASSWORD, DB_NAME, DB_HOST);
// Initialize read connections
$this->read_connections[] = new wpdb(DB_USER, DB_PASSWORD, DB_NAME, DB_HOST_READ1);
$this->read_connections[] = new wpdb(DB_USER, DB_PASSWORD, DB_NAME, DB_HOST_READ2);
// Add more connections as needed
}
// Custom query routing logic
function query($query) {
// Determine if query is a read operation
if ($this->is_read_query($query)) {
// Implement load-balancing among read servers
$read_db = $this->select_read_connection();
return $read_db->query($query);
} else {
// For write operations, use the master database
return $this->write_connection->query($query);
}
}
// Logic to determine if a query is for reading
function is_read_query($query) {
$read_query_types = ['SELECT', 'DESCRIBE', 'EXPLAIN'];
foreach ($read_query_types as $type) {
if (stripos(trim($query), $type) === 0) {
return true;
}
}
return false;
}
// Logic to select a read connection (simple load balancer)
function select_read_connection() {
// This can be a round-robin or random selection mechanism
// Example: Random selection
return $this->read_connections[array_rand($this->read_connections)];
}
}
-
Overriding the Global
$wpdb
Instance:
Replace the default $wpdb
with an instance of your custom router:
global $wpdb;
$wpdb = new Custom_Read_Write_DB();
Remember
-
Rigorously test your setup. Check if read queries are correctly distributed among slave databases and write queries are going to the master.
-
Monitor query performance and optimize the load-balancing algorithm. You might implement more sophisticated methods like least connections or response time.
-
Implement logic to switch to another read database or the master database in case a slave database fails.
Implementing a read-write database pattern in WordPress with multiple MySQL connections for reading and a dedicated connection for writing can significantly improve the performance of high-traffic websites. While this setup requires careful configuration and testing, the benefits in scalability and efficiency make it a worthwhile investment for large-scale WordPress deployments.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
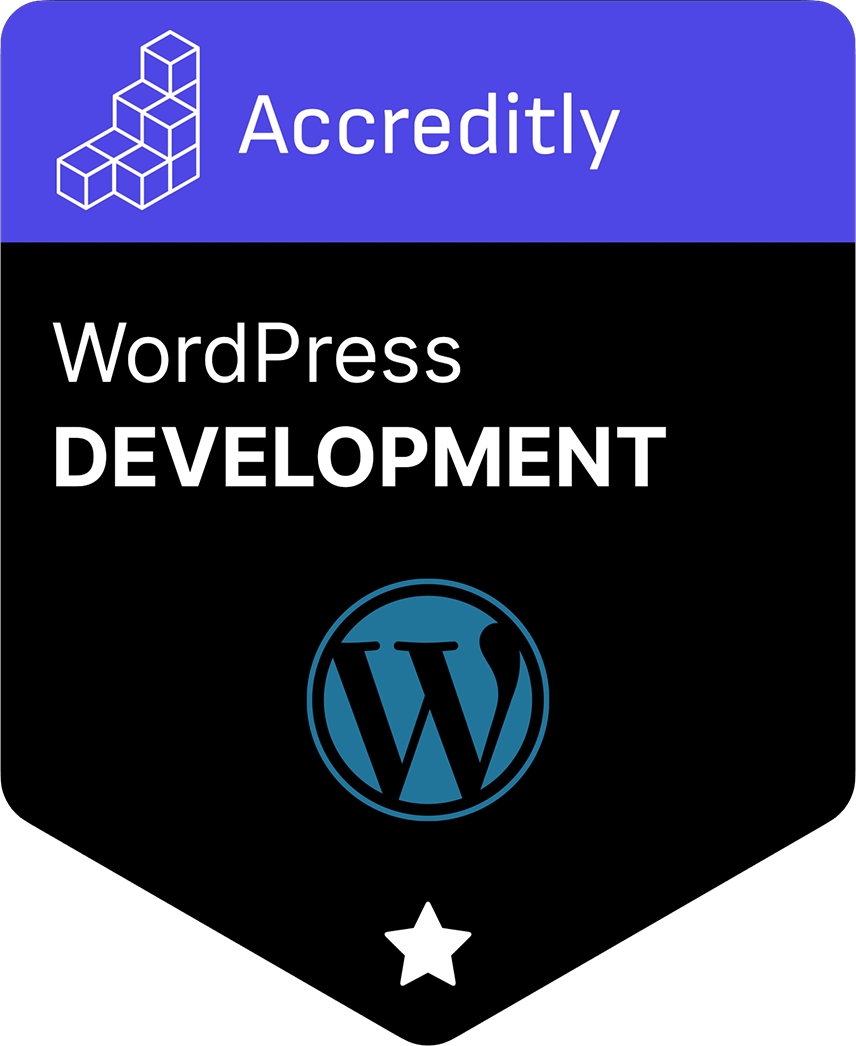