- 1. Designing the Form Structure
- 2. Creating the Form in WordPress
- 3. Styling the Form
- 4. Adding Navigation Logic with JavaScript
- 5. Handling Form Submission with PHP
- 6. Testing and Validation
- 7. Possible improvements
Multi-step forms enhance user engagement by breaking down complex forms into manageable sections. Building one in WordPress without plugins allows for greater customization. This guide walks you through creating a multi-step form with actual fields and demonstrates how to handle submission by sending an email.
1. Designing the Form Structure
Decide on the number of steps and the fields you want to include in each. For instance:
- Step 1: Personal Information
- Step 2: Contact Details
- Step 3: Confirmation & Submission
2. Creating the Form in WordPress
You can embed the form in a WordPress page or post, or directly in a theme file. Here's an example HTML structure:
<form id="multiStepForm" method="post">
<!-- Step 1: Personal Information -->
<div class="form-step" id="step1">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="age">Age:</label>
<input type="number" id="age" name="age" required>
<button type="button" onclick="nextStep()">Next</button>
</div>
<!-- Step 2: Contact Details -->
<div class="form-step" id="step2" style="display:none;">
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<button type="button" onclick="previousStep()">Back</button>
<button type="button" onclick="nextStep()">Next</button>
</div>
<!-- Step 3: Confirmation & Submission -->
<div class="form-step" id="step3" style="display:none;">
<p>Please confirm your information and submit the form.</p>
<button type="submit">Submit</button>
</div>
</form>
3. Styling the Form
Add CSS to style your form. This can be included in your theme’s main stylesheet or in a style tag on your page.
.form-step {
margin-bottom: 20px;
}
input, button {
margin-top: 10px;
}
4. Adding Navigation Logic with JavaScript
Use JavaScript to handle navigation between form steps:
let currentStep = 1;
function showStep(step) {
document.getElementById('step' + currentStep).style.display = 'none';
document.getElementById('step' + step).style.display = 'block';
currentStep = step;
}
function nextStep() {
showStep(currentStep + 1);
}
function previousStep() {
showStep(currentStep - 1);
}
5. Handling Form Submission with PHP
In your theme’s functions.php
file or a custom plugin, handle the form submission:
if ($_SERVER['REQUEST_METHOD'] == 'POST' && !empty($_POST['email'])) {
$to = '[email protected]';
$subject = 'New Submission from Multi-Step Form';
$message = "Name: " . $_POST['name'] . "\n";
$message .= "Age: " . $_POST['age'] . "\n";
$message .= "Email: " . $_POST['email'];
$headers = 'From: [email protected]';
wp_mail($to, $subject, $message, $headers);
// Redirect or display a success message
}
6. Testing and Validation
Ensure all fields work as expected, and the form submission sends the email correctly. Implement validation to improve user experience and data integrity.
7. Possible improvements
Add a Progress Bar Consider showing a progress bar as the user moves through the form.
Responsive Design Make sure the form is accessible and user-friendly on all devices. You'd usually handle this within a framework of some kind, but we've left this out of this tutorial to not confuse things.
Client-Side Validation Use JavaScript to validate fields before submission for immediate feedback. This is not a replacement for server-side validation, but instead as an addition which shows the user validation feedback without needing to submit a HTTP request.
Creating a multi-step form in WordPress without a plugin is a practical approach to customizing user experience and functionality. By embedding the form into your WordPress site and handling submissions via email, you can maintain control over the form’s appearance and behavior. This approach not only enhances user engagement but also aligns with your website's specific requirements.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
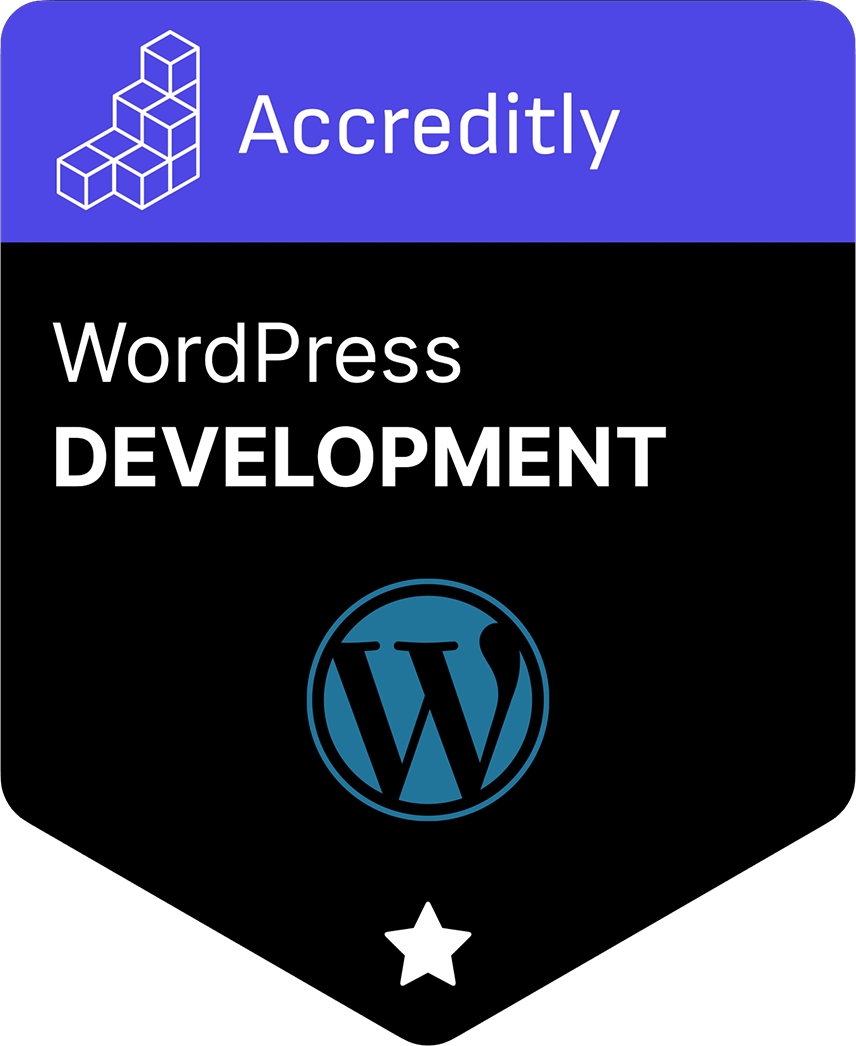