- 1. Implicit Route Model Binding
- 2. Customizing The Key Name
- 3. Explicit Route Model Binding
- 4. Route Model Binding with Custom Controllers
- Keep It Clean
Laravel's Eloquent ORM is one of the key features that developers love about the framework. While Eloquent makes database interactions extremely easy, Laravel takes it a step further with Route Model Binding. This feature helps you directly resolve Eloquent models from route parameters, reducing repetitive code and making controllers neater.
So, how can you leverage Route Model Binding to its fullest? Let's explore.
1. Implicit Route Model Binding
Laravel attempts to simplify and automate many common tasks, and Implicit Route Model Binding is a great example. Instead of explicitly querying for a model in your controller's method, Laravel will do it for you.
Here's a basic comparison:
Without Route Model Binding:
public function show($id) {
$post = Post::findOrFail($id);
return view('posts.show', compact('post'));
}
With Implicit Route Model Binding:
public function show(Post $post) {
return view('posts.show', compact('post'));
}
If the specified post exists, it's automatically injected into the method. If not, a 404 response is generated.
2. Customizing The Key Name
By default, Laravel uses the Eloquent model's primary key for model binding. However, there are times when you might want to bind a model based on another column, like a slug.
In your model, override the getRouteKeyName
method:
public function getRouteKeyName() {
return 'slug';
}
Now, Laravel will automatically use the slug
column for route model binding.
3. Explicit Route Model Binding
For more control, Laravel offers Explicit Route Model Binding. This allows you to define custom logic to resolve your bindings. To use this, add bindings in the boot
method of your RouteServiceProvider
:
public function boot() {
parent::boot();
Route::bind('post', function ($value) {
return App\Models\Post::where('name', $value)->firstOrFail();
});
}
Now, whenever a post
parameter appears in the route, Laravel will use the provided closure to fetch the model.
4. Route Model Binding with Custom Controllers
You aren't limited to using Route Model Binding just with resource controllers. It works seamlessly with any controller method as long as the type-hinted variable name matches the route segment name.
Route::get('posts/{post}/comments/{comment}', [CommentController::class, 'show']);
In your controller:
public function show(Post $post, Comment $comment) {
// Both $post and $comment are automatically resolved.
}
Keep It Clean
Route Model Binding is more than just a cool feature; it embodies the Laravel philosophy of elegant coding. By removing redundant model queries from controllers, your application becomes cleaner and more maintainable. It's another reason Laravel continues to be a favorite among web developers.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
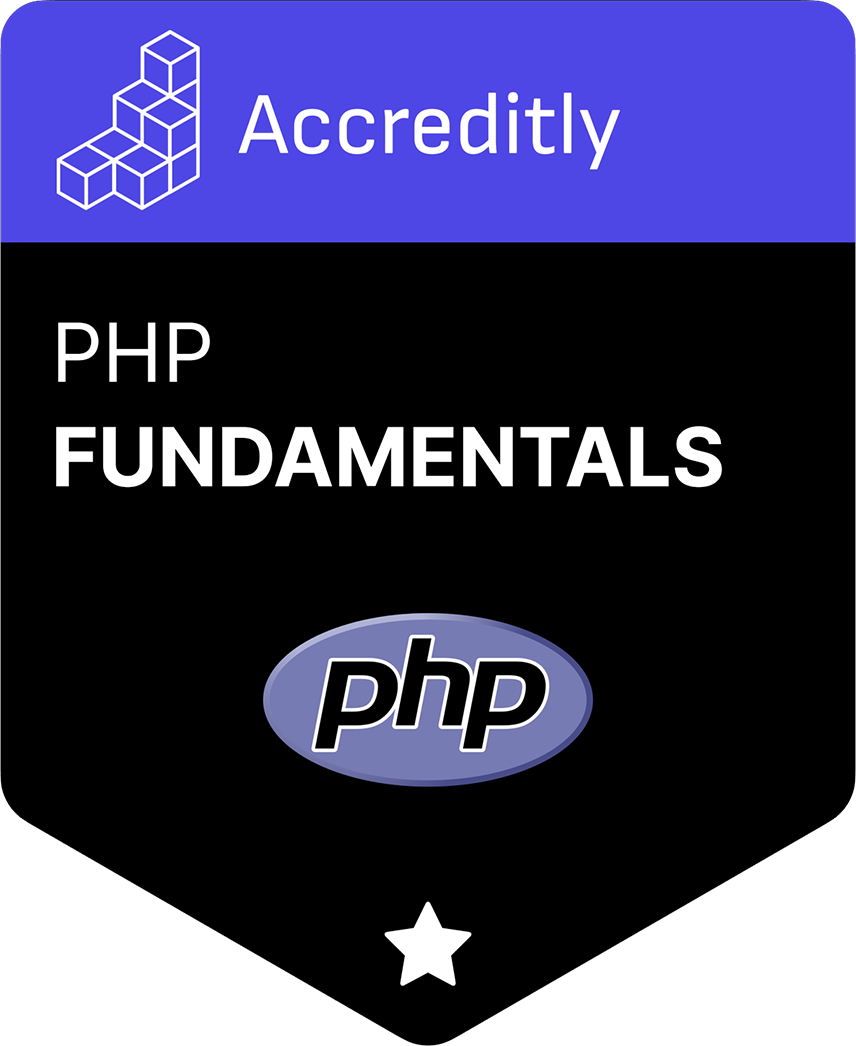