Gating content behind an email form is a common practice to collect leads and engage users. This technique requires users to provide their email address to access certain content. In this article, we'll explore how to gate content behind an email form in WordPress using plugins and custom code.
Prerequisites
Before starting, ensure you have a WordPress website set up. If you need help setting up WordPress, refer to the WordPress installation guide.
Method 1: Using a Plugin
The easiest way to gate content behind an email form in WordPress is by using a plugin. Several plugins can help you achieve this without writing custom code.
Recommended Plugin: Email Subscribers & Newsletters
Email Subscribers & Newsletters is a popular plugin that allows you to create subscription forms and manage email lists. It also provides options to restrict content based on email subscription.
Installation and Setup:
-
Install the Plugin: Go to Plugins > Add New in your WordPress dashboard, search for "Email Subscribers & Newsletters," and install it.
-
Activate the Plugin: Once installed, activate the plugin.
-
Create a Subscription Form: Go to Email Subscribers > Forms and create a new subscription form.
-
Restrict Content: Use the shortcode provided by the plugin to restrict content. For example:
[email-subscribers namefield="YES" desc="" group="Public"]
Method 2: Using Custom Code
For more control and customization, you can use custom code to gate content behind an email form. This method involves creating a custom form, handling form submissions, and restricting content access.
Step 1: Create a Custom Form
First, create a custom form using HTML and place it in your WordPress theme or a custom plugin.
// Custom form HTML
function custom_email_form() {
if (!isset($_POST['submit'])) {
?>
<form method="post">
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<input type="submit" name="submit" value="Submit">
</form>
<?php
}
}
add_shortcode('custom_email_form', 'custom_email_form');
Step 2: Handle Form Submission
Next, handle the form submission and store the email address in the database or send it to an email marketing service.
function handle_email_form_submission() {
if (isset($_POST['submit'])) {
$email = sanitize_email($_POST['email']);
if (is_email($email)) {
// Store email or send to email marketing service
// For example, store in a custom database table
global $wpdb;
$table_name = $wpdb->prefix . 'email_subscribers';
$wpdb->insert($table_name, ['email' => $email]);
// Set a cookie to remember the user
setcookie('email_subscribed', '1', time() + (86400 * 30), '/'); // 30 days expiration
// Redirect to the same page to display content
wp_redirect(get_permalink());
exit;
}
}
}
add_action('init', 'handle_email_form_submission');
Step 3: Restrict Content Access
Finally, restrict access to the content based on whether the user has submitted their email.
function restrict_content($content) {
if (!isset($_COOKIE['email_subscribed'])) {
return do_shortcode('[custom_email_form]'); // Display the form
}
return $content; // Display the content
}
add_filter('the_content', 'restrict_content');
Conclusion
Gating content behind an email form in WordPress is an effective way to collect leads and engage users. By using a plugin like Email Subscribers & Newsletters or implementing custom code, you can easily restrict access to your content and collect email addresses.
For more detailed information, refer to the WordPress Plugin Handbook and the WordPress Codex. By following these steps, you can enhance your website's user engagement and lead generation capabilities.
Interested in proving your knowledge of this topic? Take the WordPress Development certification.
WordPress Development
Covering all aspects of WordPress web development, from theme development, plugin development, server set up and configuration and optimisation.
$99
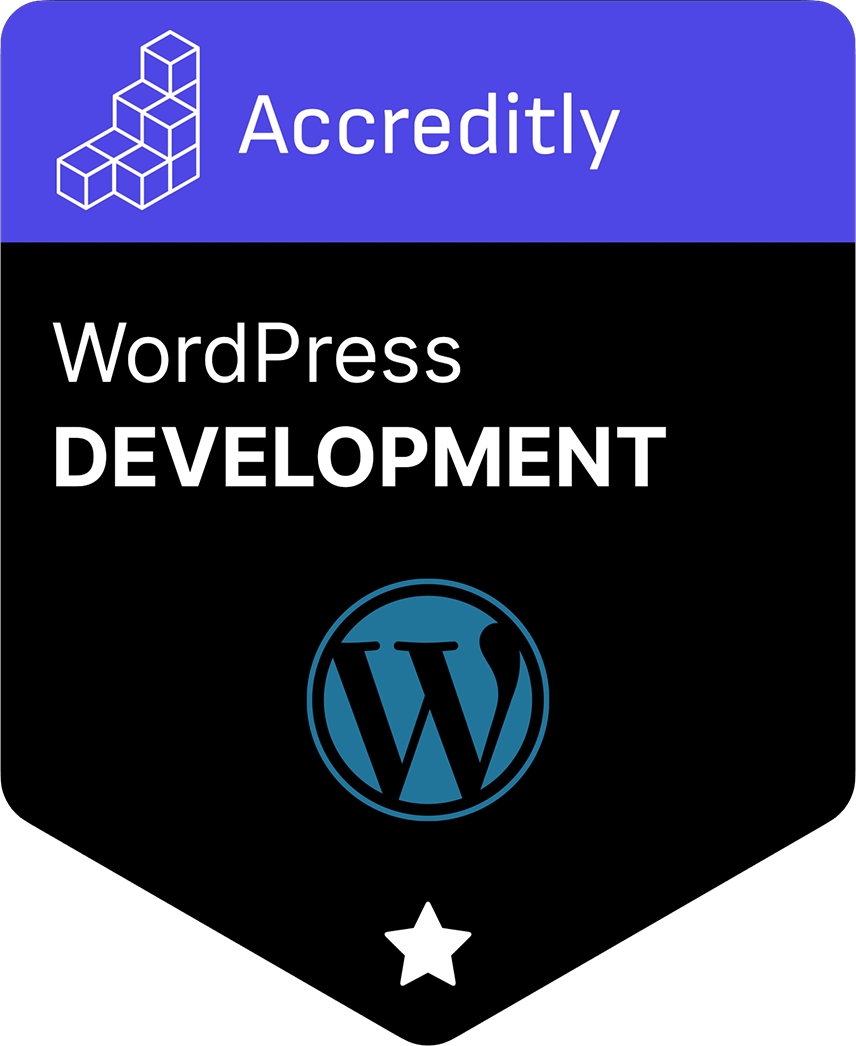