- 1. Understanding the Problem
- 2. The FileReader API
- 3. Streaming and Chunking
- 4. Advantages of Chunking
- 5. Best Practices
Reading large files in JavaScript can be a challenge. Traditional file reading methods can freeze the UI, leading to a poor user experience. Efficiently reading such files requires a different approach -- one that prioritizes memory usage and application responsiveness.
1. Understanding the Problem
Traditional file reading operations in JavaScript can be memory-intensive. Loading a large file entirely into memory can:
- Lead to high memory usage.
- Cause the browser or application to freeze or crash.
- Result in long wait times for users.
2. The FileReader API
The FileReader
API allows web applications to read the contents of files (or raw data buffers) asynchronously.
Standard Approach:
let input = document.getElementById('fileInput');
input.addEventListener('change', handleFile, false);
function handleFile(e) {
let file = e.target.files[0];
let reader = new FileReader();
reader.onload = function(event) {
console.log(event.target.result); // This contains the file data.
}
reader.readAsText(file);
}
This method reads the entire file at once, which isn't suitable for large files.
3. Streaming and Chunking
Chunking involves breaking a file into smaller parts and processing each chunk sequentially, instead of processing the entire file at once.
function readInChunks(file) {
let CHUNK_SIZE = 512 * 1024; // 512KB
let offset = 0;
let reader = new FileReader();
reader.onload = function(event) {
if (event.target.result.length > 0) {
console.log(event.target.result); // process the chunk of data here
offset += CHUNK_SIZE;
readNext();
} else {
// Done reading file
console.log("Finished reading file.");
}
};
function readNext() {
let slice = file.slice(offset, offset + CHUNK_SIZE);
reader.readAsText(slice);
}
readNext();
}
let input = document.getElementById('fileInput');
input.addEventListener('change', function(e) {
readInChunks(e.target.files[0]);
}, false);
4. Advantages of Chunking
-
Memory Efficiency: Since only a portion of the file is read into memory at any time, chunking is more memory-efficient.
-
Responsive UI: As the browser isn't trying to process a large file in one go, the UI remains responsive.
5. Best Practices
-
Adaptive Chunk Size: You might need to adjust the
CHUNK_SIZE
based on the type and size of the files you're dealing with. -
Handling Errors: The
FileReader
API emits errors, which should be captured using theonerror
handler to ensure a graceful failure. -
Feedback to Users: For a better user experience, provide feedback like progress bars to indicate the progress of the file reading process.
Reading large files in JavaScript requires an understanding of the challenges associated with large datasets and the techniques to mitigate them. By adopting chunking and streaming, developers can efficiently read large files, ensuring both optimal performance and a responsive user experience.
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
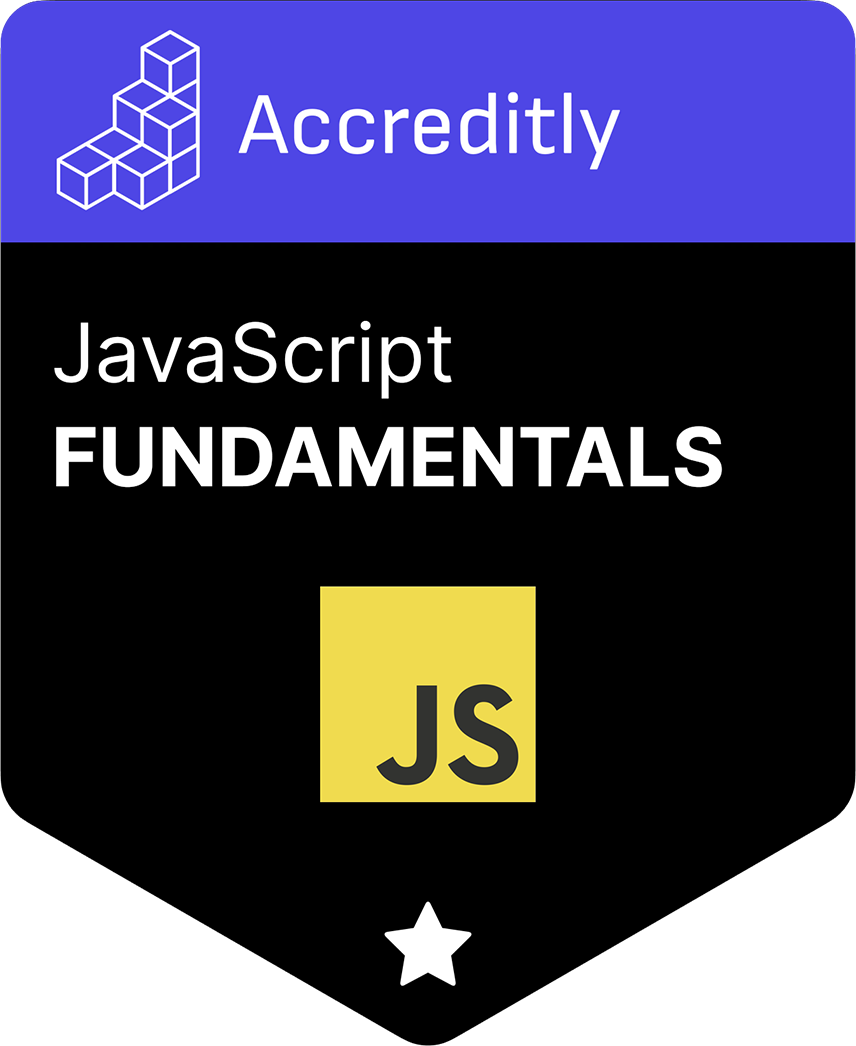