- Getting Started
- Example 1: Performing a Simple GET Request
- Example 2: GET Request with Query Parameters
- Example 3: POST Request with Data
- Example 4: Uploading a File with a POST Request
- Example 5: PATCH and PUT Requests
- Example 6: DELETE Request
- Example 7: Using Async/Await with Axios
- Example 8: Configuring Request Headers
- Example 9: Handling Errors
- Example 10: Cancellation
- Example 11: Interceptors
Axios is a powerful, promise-based HTTP client for JavaScript, designed for use in both the browser and Node.js environments. It provides an easy-to-use and consistent API for making HTTP requests, handling responses, and managing errors. With Axios, you can perform various HTTP operations such as GET, POST, PUT, DELETE, and more.
The popularity of Axios stems from its features, which include intercepting requests and responses, cancellation of requests, automatic transformation of JSON data, and protection against Cross-Site Request Forgery (CSRF) attacks.
In this blog post, we will explore Axios in detail and demonstrate how to use it effectively in your JavaScript applications. We will provide various examples of Axios usage to cover different aspects of the library.
Getting Started
Before diving into examples, let's first install Axios and import it into our project. Axios can be installed using npm or yarn:
npm install axios
or
yarn add axios
Once Axios is installed, you can import it in your JavaScript file:
const axios = require('axios'); // For Node.js
// or
import axios from 'axios'; // For ES6 modules
If you're working in a browser environment, you can include Axios using a script tag:
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
Now that Axios is installed and imported, let's move on to some examples. In all of the examples we use Typicode's JSON Placeholder service.
Example 1: Performing a Simple GET Request
Axios makes it easy to perform HTTP requests. Let's start with a simple GET request to fetch data from a public API:
axios
.get('https://jsonplaceholder.typicode.com/todos/1')
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
In this example, we use the axios.get()
method to fetch data from the JSONPlaceholder API. The method returns a promise, which we handle using .then()
to access the response data and .catch()
to handle errors.
Example 2: GET Request with Query Parameters
Sometimes, you may need to include query parameters in your GET requests. Axios makes this easy by allowing you to pass a params
object:
const queryParams = {
userId: 1,
};
axios
.get('https://jsonplaceholder.typicode.com/todos', { params: queryParams })
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
In this example, we fetch todos for a specific user by passing the userId
as a query parameter. The params
object is added as a property of the configuration object, which is the second argument of the axios.get()
method.
Example 3: POST Request with Data
To create a new resource using a POST request, you can use the axios.post()
method:
const data = {
title: 'My new todo',
completed: false,
};
axios
.post('https://jsonplaceholder.typicode.com/todos', data)
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
Here, we pass an object containing the new todo's data to the axios.post()
method.
Axios have a post request example on their website too.
Example 4: Uploading a File with a POST Request
Axios can also be used to upload files by sending a POST request with a FormData
object:
const fileInput = document.querySelector('#fileInput');
const formData = new FormData();
fileInput.addEventListener('change', (event) => {
formData.append('file', event.target.files[0]);
axios
.post('https://api.example.com/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data',
},
})
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
});
In this example, we create a FormData
object and append a file from a file input element to it. Then, we send the FormData
object in a POST request to the server with the appropriate Content-Type
header.
There are other methods too, as detailed in the docs.
Example 5: PATCH and PUT Requests
Axios also supports PATCH and PUT requests to update resources. Here's an example of using the axios.patch()
method:
const data = {
title: 'Updated todo',
};
axios
.patch('https://jsonplaceholder.typicode.com/todos/1', data)
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
And here's an example of using the axios.put()
method:
const data = {
title: 'Updated todo',
completed: true,
};
axios
.put('https://jsonplaceholder.typicode.com/todos/1', data)
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
In both examples, we pass the updated data as the second argument to the respective methods.
Example 6: DELETE Request
To delete a resource, you can use the axios.delete()
method:
axios
.delete('https://jsonplaceholder.typicode.com/todos/1')
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
This example demonstrates how to delete a todo by sending a DELETE request to the server.
Example 7: Using Async/Await with Axios
Axios is promise-based, making it compatible with async
/await
. Here's an example using async
/await
with a GET request:
async function fetchTodo() {
try {
const response = await axios.get('https://jsonplaceholder.typicode.com/todos/1');
console.log(response.data);
} catch (error) {
console.error(error);
}
}
fetchTodo();
In this example, we create an async
function called fetchTodo()
and use the await keyword to wait for the Axios request to complete. This approach simplifies the code, making it more readable.
Example 8: Configuring Request Headers
Axios allows you to set custom headers for your HTTP requests. Here's an example of setting the Content-Type
and Authorization
headers:
const config = {
headers: {
'Content-Type': 'application/json',
Authorization: 'Bearer ' + 'your_access_token',
},
};
axios
.get('https://api.example.com/secure-data', config)
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
In this example, we create a config object containing our custom headers and pass it to the axios.get()
method.
Example 9: Handling Errors
Axios allows you to handle errors effectively. The .catch() block can be used to catch any errors that occur during the request:
axios
.get('https://jsonplaceholder.typicode.com/todos/1')
.then((response) => {
console.log(response.data);
})
.catch((error) => {
if (error.response) {
// The request was made, and the server responded with a status code that falls out of the range of 2xx
console.error(error.response.status, error.response.data);
} else if (error.request) {
// The request was made, but no response was received
console.error(error.request);
} else {
// Something happened in setting up the request that triggered an Error
console.error('Error', error.message);
}
console.error(error.config);
});
This example demonstrates how to handle different types of errors that might occur during an Axios request.
Axios have a few other examples of handling errors in their documentation.
Example 10: Cancellation
Axios supports cancellation of requests using the CancelToken
:
const CancelToken = axios.CancelToken;
const source = CancelToken.source();
axios
.get('https://jsonplaceholder.typicode.com/todos/1', {
cancelToken: source.token,
})
.then((response) => {
console.log(response.data);
})
.catch((thrown) => {
if (axios.isCancel(thrown)) {
console.log('Request canceled', thrown.message);
} else {
// handle error
console.error(thrown);
}
});
// Cancel the request
source.cancel('Operation cancelled by the user.');
In this example, we create a CancelToken
and pass it to the request configuration. The request can be cancelled later by calling the source.cancel()
method.
Axios have some more examples in their docs.
Example 11: Interceptors
Axios interceptors allow you to modify requests and responses before they are handled by the .then()
or .catch()
blocks. Here's an example of using interceptors to add a request header:
axios.interceptors.request.use((config) => {
config.headers['X-Custom-Header'] = 'CustomHeaderValue';
return config;
});
axios
.get('https://jsonplaceholder.typicode.com/todos/1')
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
In this example, we add an interceptor to modify the request configuration and add a custom header before the request is sent. Read more about interceptors in the docs.
These examples should give you a comprehensive understanding of Axios and its various features. By exploring the Axios documentation, you can learn about more advanced features and configurations to further enhance your JavaScript applications. Happy coding!
Interested in proving your knowledge of this topic? Take the JavaScript Fundamentals certification.
JavaScript Fundamentals
Showcase your knowledge of JavaScript in this exam, featuring questions on the language, syntax and features.
$99
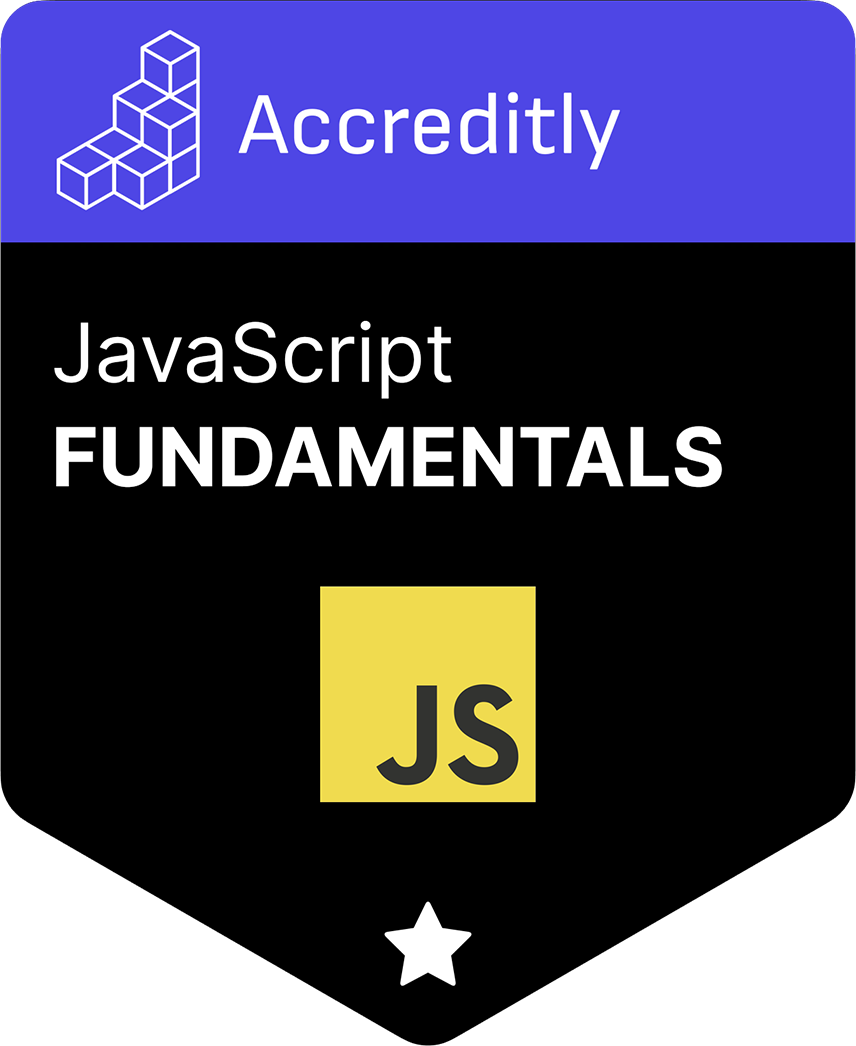