SQL injection is a common security vulnerability that affects web applications that use databases. By exploiting an SQL injection vulnerability, an attacker can manipulate, steal, or even delete sensitive data. In this blog post, we will discuss SQL injection and how to prevent it in PHP using various techniques such as PDO, MySQLi, PHP 8.2's execute_query, and PHP 8.1's prepare with bind_params (which is available from PHP 5 onwards).
What is SQL Injection?
SQL injection is a type of security attack where an attacker submits malicious SQL code through user input fields, such as forms or URL parameters. This malicious code can then be executed by the database server, giving the attacker unauthorized access to manipulate or retrieve data.
For example, consider a simple login form where users input their email and password. An attacker could input a specially crafted SQL statement in the email field, which, when executed, bypasses the password check and grants them access to the user's account.
Preventing SQL Injection in PHP
To prevent SQL injection, we need to ensure that user input is properly sanitized and validated before being used in SQL queries. There are several techniques to achieve this in PHP:
Using PDO (PHP Data Objects)
PDO is an extension that provides a consistent interface for working with databases in PHP. One of the benefits of using PDO is its support for prepared statements, which help prevent SQL injection by separating SQL query logic from user-supplied data. Here's an example of how to use PDO with prepared statements:
// Connect to the database using PDO
$dsn = "mysql:host=localhost;dbname=mydb";
$username = "username";
$password = "password";
$conn = new PDO($dsn, $username, $password);
// Prepare an SQL statement with placeholders for user-supplied data
$sql = "INSERT INTO users (username, email) VALUES (:username, :email)";
$stmt = $conn->prepare($sql);
// Bind user-supplied data to placeholders and execute the statement
$stmt->bindParam(':username', $_POST['username'], PDO::PARAM_STR);
$stmt->bindParam(':email', $_POST['email'], PDO::PARAM_STR);
$stmt->execute();
Using MySQLi (MySQL Improved Extension)
MySQLi is another extension that provides an interface for working with MySQL databases in PHP. Like PDO, MySQLi supports prepared statements, which can help prevent SQL injection. Here's an example of how to use MySQLi with prepared statements:
// Connect to the database using MySQLi
$conn = new mysqli("localhost", "username", "password", "mydb");
// Prepare an SQL statement with placeholders for user-supplied data
$sql = "INSERT INTO users (username, email) VALUES (?, ?)";
$stmt = $conn->prepare($sql);
// Bind user-supplied data to placeholders and execute the statement
$stmt->bind_param("ss", $_POST['username'], $_POST['email']);
$stmt->execute();
$stmt->close();
$conn->close();
Using PHP 8.2's execute_query
PHP 8.2 introduced a new function called execute_query, which simplifies the process of executing prepared statements. This function automatically handles parameter binding and query execution, making it easier to prevent SQL injection:
$conn = new mysqli("localhost", "username", "password", "mydb");
// Prepare and execute an SQL statement with user-supplied data
$sql = "INSERT INTO users (username, email) VALUES (?, ?)";
$result = $conn->execute_query($sql, [$_POST['username'], $_POST['email']]);
$conn->close();
Wrapping it up
In conclusion, SQL injection is a serious security threat that can lead to unauthorized access and manipulation of sensitive data. It has been one of the most exploited attack vectors for poorly written websites for a long time.
By using proper techniques like PDO, MySQLi, and PHP 8.2's execute_query, developers can effectively prevent SQL injection in their PHP applications. Always ensure that user input is properly sanitized and validated before being used in SQL queries to maintain the security and integrity of your web applications.
Interested in proving your knowledge of this topic? Take the PHP Fundamentals certification.
PHP Fundamentals
Covering the required knowledge to create and build web applications in PHP.
$99
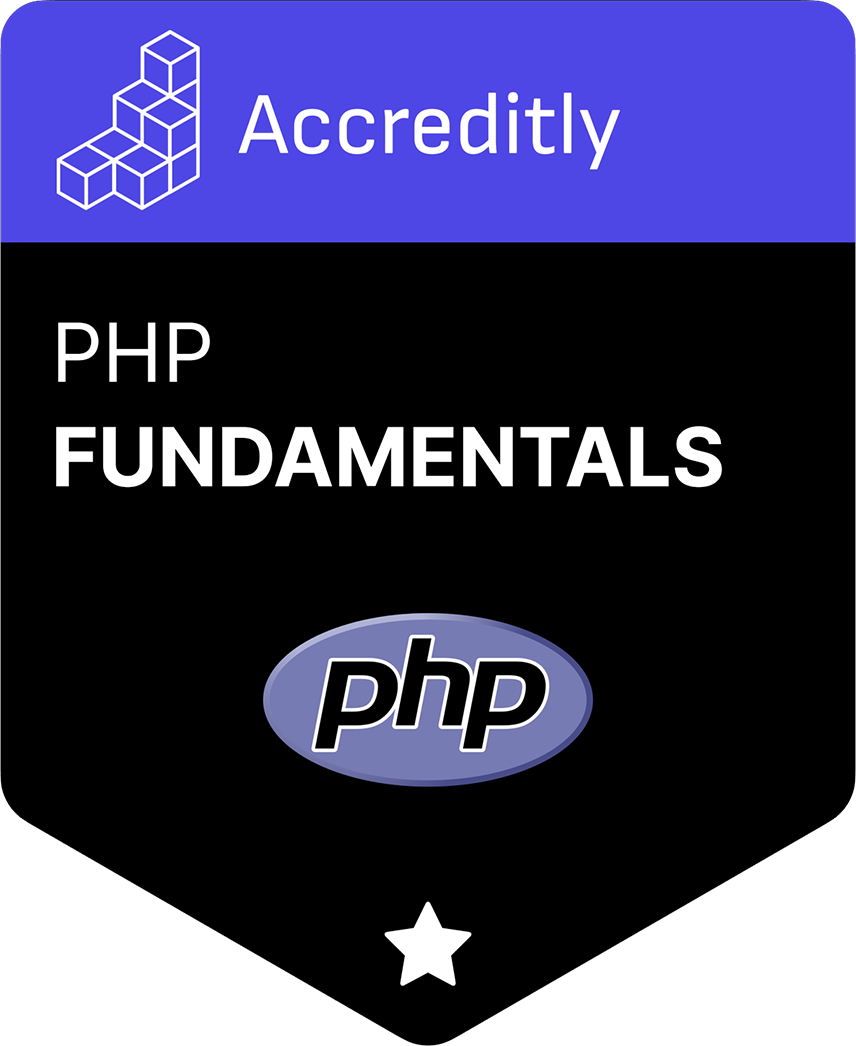